Leetcode 988: Smallest String Starting From Leaf
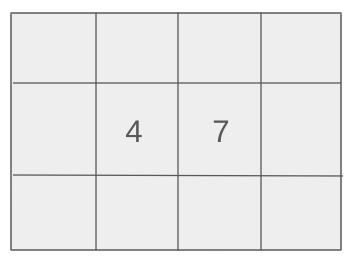
You are given the root of a binary tree, where each node contains a value between 0 and 25, corresponding to letters from ‘a’ to ‘z’. Your task is to find the lexicographically smallest string that can be formed by traversing from a leaf node to the root node, using the values in each node as letters.
Problem
Approach
Steps
Complexity
Input: The input consists of the root of a binary tree, where each node has an integer value in the range [0, 25].
Example: root = [0, 1, 2, 3, 4, 3, 4]
Constraints:
• The number of nodes in the tree is between 1 and 8500.
• 0 <= Node.val <= 25.
Output: The output should be the lexicographically smallest string that starts from a leaf node and ends at the root.
Example: Output: "dbd"
Constraints:
• The string must be constructed by following the path from a leaf node to the root.
Goal: The goal is to traverse the binary tree from each leaf node to the root, constructing strings and determining the lexicographically smallest one.
Steps:
• Traverse the tree recursively from root to leaf nodes.
• At each leaf node, generate the corresponding string by adding the character of the node to the string.
• Compare the generated string with the current smallest string and update accordingly.
Goal: The solution must handle the constraints efficiently given the possible size of the binary tree.
Steps:
• The number of nodes is between 1 and 8500, so a recursive solution with backtracking will be efficient enough.
Assumptions:
• The tree is a binary tree with no cycles.
• The tree contains at least one node (i.e., the root is not null).
• Input: root = [0, 1, 2, 3, 4, 3, 4]
• Explanation: In this example, the binary tree has leaf nodes with values corresponding to 'd', 'b', and 'd'. The lexicographically smallest string is formed by starting from the leaf 'd' and traversing back to the root, resulting in 'dbd'.
Approach: The approach involves recursively traversing the tree and constructing strings from leaf nodes to the root while keeping track of the lexicographically smallest string.
Observations:
• We need to traverse each path from a leaf to the root and compare the strings lexicographically.
• Using recursion allows us to easily explore all paths from leaf to root and compare the strings efficiently.
Steps:
• Initialize a variable to store the lexicographically smallest string.
• Use a recursive function to explore each node and append its value as a character to the string.
• At each leaf node, compare the constructed string with the current smallest string and update if necessary.
Empty Inputs:
• Handle the case where the tree has only one node.
Large Inputs:
• Ensure the solution handles the maximum number of nodes efficiently.
Special Values:
• Consider trees with values representing letters near the boundaries of the alphabet, such as 'a' (0) or 'z' (25).
Constraints:
• Ensure the algorithm runs efficiently for a large number of nodes and is optimized for recursion.
string ans = "~";
string smallestFromLeaf(TreeNode* root) {
recur(root, "");
return ans;
}
void recur(TreeNode* node, string s) {
if(!node) return;
if(!node->left && !node->right) {
ans = min(ans, char(node->val + 'a') + s);
}
cout << node->val<<'\n';
recur(node->left, char(node->val + 'a') + s);
recur(node->right, char(node->val + 'a') + s);
}
1 : Variable Initialization
string ans = "~";
Initializes the variable `ans` to `~`, which will be used to store the smallest string found from a leaf to the root during the traversal.
2 : Function Definition
string smallestFromLeaf(TreeNode* root) {
Defines the function `smallestFromLeaf`, which takes the root node of a binary tree and returns the lexicographically smallest string from a leaf node to the root.
3 : Recursive Call
recur(root, "");
Calls the helper function `recur` to start the recursive traversal of the tree. The empty string `""` is passed as the initial path.
4 : Return Result
return ans;
Returns the variable `ans`, which contains the lexicographically smallest string from a leaf node to the root after the recursion completes.
5 : Helper Function Definition
void recur(TreeNode* node, string s) {
Defines the helper function `recur`, which performs the recursive traversal of the binary tree, building the string path from the leaf to the root.
6 : Base Case
if(!node) return;
Checks if the current node is null, which is the base case for recursion. If the node is null, the function returns without doing anything.
7 : Leaf Node Check
if(!node->left && !node->right) {
Checks if the current node is a leaf node (both left and right children are null). If it's a leaf, the function proceeds to check and update the answer.
8 : Update Answer
ans = min(ans, char(node->val + 'a') + s);
Updates the `ans` variable with the lexicographically smaller string between the current `ans` and the string formed by adding the current node's value (converted to a character) to the path `s`.
9 : Recursive Call for Left Child
recur(node->left, char(node->val + 'a') + s);
Recursively calls the `recur` function for the left child of the current node, passing the current node's value (converted to a character) prepended to the string path `s`.
10 : Recursive Call for Right Child
recur(node->right, char(node->val + 'a') + s);
Recursively calls the `recur` function for the right child of the current node, prepending the current node's value (converted to a character) to the string path `s`.
Best Case: O(n) where n is the number of nodes, as each node is visited once.
Average Case: O(n) where n is the number of nodes, as each node contributes to a string comparison.
Worst Case: O(n) where n is the number of nodes, since we visit each node in the tree once.
Description: The time complexity is O(n), where n is the number of nodes in the tree, due to the tree traversal.
Best Case: O(1) if the tree is very shallow or balanced.
Worst Case: O(h) where h is the height of the tree, due to recursion stack space.
Description: The space complexity is O(h), where h is the height of the tree, due to recursion.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus