Leetcode 963: Minimum Area Rectangle II
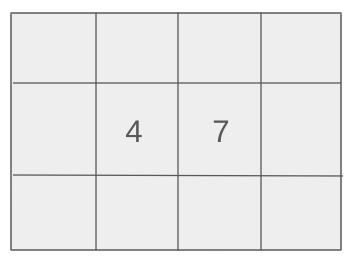
You are given a set of points in a 2D plane, represented as an array where each point is in the format [xi, yi]. Your task is to find the minimum possible area of any rectangle formed by these points, where the sides of the rectangle do not need to be parallel to the X or Y axes. If no rectangle can be formed, return 0. Answers within 10^-5 of the actual area are considered correct.
Problem
Approach
Steps
Complexity
Input: The input is an array of unique points in a 2D plane. Each point is represented as a pair of integers [xi, yi], where xi and yi denote the coordinates of the point.
Example: Input: points = [[0,2],[1,1],[2,0],[1,3],[3,1]]
Constraints:
• 1 <= points.length <= 50
• points[i].length == 2
• 0 <= xi, yi <= 40,000
• All points are unique.
Output: The output is a floating-point number representing the minimum possible area of a rectangle formed by the given points. If no rectangle can be formed, return 0.
Example: Output: 1.00000
Constraints:
• The result must be accurate within 10^-5 of the actual area.
Goal: Find the minimum area of a rectangle that can be formed using the given points. If no rectangle exists, return 0.
Steps:
• 1. Use combinations of two points to define potential rectangle sides.
• 2. Use a hashing technique to group points by their center and length for efficient pair comparisons.
• 3. Calculate the area for each possible rectangle, keeping track of the minimum value.
• 4. If no rectangle is formed, return 0.
Goal: The input array will contain between 1 and 50 points. All points are unique and lie within the range [0, 40,000] on both axes.
Steps:
• 1 <= points.length <= 50
• 0 <= xi, yi <= 40,000
Assumptions:
• The input points are unique and will not have duplicates.
• It is possible for no rectangle to be formed, in which case the output should be 0.
• Input: Input: points = [[0,2],[2,0],[1,1],[3,3]]
• Explanation: The minimum area rectangle is formed by points [0,2],[2,0],[1,1],[3,3] with an area of 2.00000.
• Input: Input: points = [[1,0],[2,2],[3,1],[0,3]]
• Explanation: No valid rectangle can be formed from these points, so the output is 0.
• Input: Input: points = [[0,0],[0,2],[2,0],[2,2]]
• Explanation: The minimum area rectangle is formed by points [0,0],[0,2],[2,0],[2,2] with an area of 4.00000.
Approach: To solve the problem, consider all pairs of points that define diagonals of a rectangle. Use hashing to efficiently group potential rectangles based on shared center and diagonal lengths. Compute the area for each valid rectangle.
Observations:
• A rectangle can be identified by its diagonals, as they share the same midpoint and length.
• Using a hash map allows efficient grouping of diagonals to form rectangles.
• Iterate through all pairs of points, calculate their center and diagonal length, and group them using a hash map.
• Check for rectangles in each group and compute their area, keeping track of the minimum area.
Steps:
• 1. Define a helper function to compute the square of the distance between two points.
• 2. Use two nested loops to generate all pairs of points.
• 3. For each pair, compute their center and diagonal length, and store them in a hash map.
• 4. For each group of potential rectangles, compute the area and update the minimum area if applicable.
• 5. If no rectangle is found, return 0.
Empty Inputs:
• The input array will always have at least one point, so empty input is not possible.
Large Inputs:
• Even with the maximum constraint of 50 points, the solution must efficiently handle O(n^2) pairwise comparisons.
Special Values:
• All points lying on a single line (horizontal, vertical, or diagonal) will not form any rectangle.
Constraints:
• All points are unique, and their coordinates lie within the valid range.
size_t d2(int x1, int y1, int x2, int y2) {
return (x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2);
}
double minAreaFreeRect(vector<vector<int>>& ps, size_t res = 0) {
unordered_map<size_t, unordered_map<size_t, vector<vector<int>>>> m;
for (auto i = 0; i < ps.size(); ++i)
for (auto j = i + 1; j < ps.size(); ++j) {
auto center = ((size_t)(ps[i][0] + ps[j][0]) << 16) + ps[i][1] + ps[j][1];
auto len = d2(ps[i][0], ps[i][1], ps[j][0], ps[j][1]);
m[center][len].push_back({ ps[i][0], ps[i][1], ps[j][0], ps[j][1] });
}
for (auto it_c = begin(m); it_c != end(m); ++it_c)
for (auto it_l = begin(it_c->second); it_l != end(it_c->second); ++it_l)
for (auto i = 0; i < it_l->second.size(); ++i)
for (auto j = i + 1; j < it_l->second.size(); ++j) {
auto &p1 = it_l->second[i], &p2 = it_l->second[j];
auto area = d2(p1[0], p1[1], p2[0], p2[1]) * d2(p1[0], p1[1], p2[2], p2[3]);
if (res == 0 || res > area) res = area;
}
return sqrt(res);
}
1 : Function Declaration
size_t d2(int x1, int y1, int x2, int y2) {
Declares the helper function to calculate the squared distance between two points.
2 : Distance Calculation
return (x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2);
Computes the squared Euclidean distance to avoid using square root for performance.
3 : Main Function Declaration
double minAreaFreeRect(vector<vector<int>>& ps, size_t res = 0) {
Declares the main function to calculate the minimum rectangle area.
4 : Hash Map Initialization
unordered_map<size_t, unordered_map<size_t, vector<vector<int>>>> m;
Initializes a hash map to group point pairs by their center and distance.
5 : Outer Loop
for (auto i = 0; i < ps.size(); ++i)
Iterates through all points as the first point of a pair.
6 : Inner Loop
for (auto j = i + 1; j < ps.size(); ++j) {
Iterates through subsequent points to form a pair with the current point.
7 : Center Calculation
auto center = ((size_t)(ps[i][0] + ps[j][0]) << 16) + ps[i][1] + ps[j][1];
Calculates a unique key representing the center of the line segment formed by the pair.
8 : Distance Calculation
auto len = d2(ps[i][0], ps[i][1], ps[j][0], ps[j][1]);
Computes the squared length of the line segment formed by the pair.
9 : Group Pairs
m[center][len].push_back({ ps[i][0], ps[i][1], ps[j][0], ps[j][1] });
Groups the pair into the hash map based on center and distance.
10 : Iterate Groups
for (auto it_c = begin(m); it_c != end(m); ++it_c)
Iterates over each center group in the hash map.
11 : Iterate Lengths
for (auto it_l = begin(it_c->second); it_l != end(it_c->second); ++it_l)
Iterates over each length group within a center group.
12 : Compare Pairs
for (auto i = 0; i < it_l->second.size(); ++i)
Iterates over pairs in the current group to compare them.
13 : Pair Comparisons
for (auto j = i + 1; j < it_l->second.size(); ++j) {
Compares pairs to check if they form a rectangle.
14 : Rectangle Properties
auto &p1 = it_l->second[i], &p2 = it_l->second[j];
Extracts the two pairs of points to calculate rectangle properties.
15 : Area Calculation
auto area = d2(p1[0], p1[1], p2[0], p2[1]) * d2(p1[0], p1[1], p2[2], p2[3]);
Calculates the area of the rectangle formed by the pairs.
16 : Update Result
if (res == 0 || res > area) res = area;
Updates the minimum area if a smaller rectangle is found.
17 : Return Result
return sqrt(res);
Returns the square root of the minimum area found.
Best Case: O(n^2)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The time complexity is O(n^2) due to the nested loop for generating pairs of points.
Best Case: O(n)
Worst Case: O(n^2)
Description: The space complexity is determined by the size of the hash map storing potential diagonals.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus