Leetcode 926: Flip String to Monotone Increasing
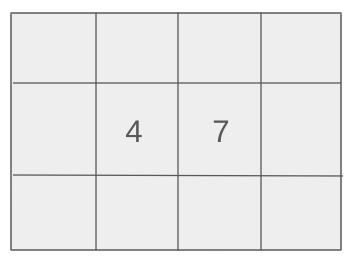
You are given a binary string s consisting of only ‘0’s and ‘1’s. A binary string is called monotone increasing if all ‘0’s appear before ‘1’s. You can flip any character in the string, changing it from ‘0’ to ‘1’ or from ‘1’ to ‘0’. Your task is to return the minimum number of flips required to make the string monotone increasing.
Problem
Approach
Steps
Complexity
Input: You are given a binary string s. The string consists of only '0' and '1'.
Example: Input: s = "01010"
Constraints:
• 1 <= s.length <= 10^5
• Each character in s is either '0' or '1'.
Output: Return the minimum number of flips required to make the string monotone increasing.
Example: Output: 2
Constraints:
• The string will have at least one character.
Goal: To find the minimum number of flips needed to make the string monotone increasing.
Steps:
• 1. Initialize two counters: one for the number of '1's encountered and one for the number of flips.
• 2. Iterate through the string. For each '1', increment the counter for '1's.
• 3. For each '0', update the flip counter to the minimum of the current flips or the number of '1's encountered so far, as flipping '0' to '1' can make the string more monotone.
• 4. Return the final value of the flip counter.
Goal: The string contains only '0's and '1's, and the length of the string is at least 1 and at most 100,000 characters.
Steps:
• 1 <= s.length <= 100000
• s consists of only '0's and '1's.
Assumptions:
• The input string is valid and consists only of '0's and '1's.
• Input: Input: s = "101010"
• Explanation: In this case, we can flip the '1' at position 1 and the '0' at position 2 to get a monotone increasing string '000111'. Thus, the minimum number of flips is 2.
• Input: Input: s = "11000"
• Explanation: Here, the string is already monotone increasing, so no flips are required, and the output is 0.
Approach: The solution approach involves traversing the string once and tracking the number of '1's and '0's. We update the minimum flips required dynamically based on the current characters.
Observations:
• The string needs to be split into two parts: all '0's followed by all '1's.
• Flipping any '1' to '0' or '0' to '1' can help achieve a monotone string.
• We can solve this problem in linear time by tracking the number of '1's and calculating the minimum flips as we traverse the string.
Steps:
• Step 1: Initialize two variables: 'cnt_one' to track the number of '1's and 'cnt_flip' to track the minimum flips required.
• Step 2: Iterate over each character in the string. If it's '1', increment 'cnt_one'. If it's '0', increment 'cnt_flip' and update it to the minimum of 'cnt_one' and 'cnt_flip'.
• Step 3: After the loop, 'cnt_flip' will contain the minimum number of flips required to make the string monotone increasing.
Empty Inputs:
• There will always be at least one character in the input string.
Large Inputs:
• For large inputs (up to 100,000 characters), the algorithm needs to run efficiently in linear time.
Special Values:
• If the string is already monotone increasing, the minimum flips should be 0.
Constraints:
• The input string consists of only '0's and '1's.
int minFlipsMonoIncr(string s) {
int cnt_one = 0, cnt_flip = 0;
for(char c: s) {
if(c == '1') cnt_one++;
else cnt_flip++;
cnt_flip = min(cnt_one, cnt_flip);
}
return cnt_flip;
}
1 : Function Definition
int minFlipsMonoIncr(string s) {
Define the function 'minFlipsMonoIncr' that takes a binary string 's' and returns an integer representing the minimum number of flips required to make the string non-decreasing.
2 : Variable Initialization
int cnt_one = 0, cnt_flip = 0;
Initialize two variables, 'cnt_one' to count the number of 1s in the string, and 'cnt_flip' to track the number of flips required to make the string non-decreasing.
3 : For Loop
for(char c: s) {
Start a loop to iterate through each character 'c' in the string 's'.
4 : Character Check - 1
if(c == '1') cnt_one++;
If the current character is '1', increment the 'cnt_one' variable to count the 1s.
5 : Character Check - 0
else cnt_flip++;
If the current character is '0', increment the 'cnt_flip' variable to track the flips required to make the string non-decreasing.
6 : Update Flip Count
cnt_flip = min(cnt_one, cnt_flip);
At each step, update 'cnt_flip' to be the minimum of the current 'cnt_flip' and 'cnt_one'. This ensures we are counting the minimum number of flips necessary at each point.
7 : Return Result
return cnt_flip;
Return the value of 'cnt_flip', which now contains the minimum number of flips required to make the string non-decreasing.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the string. We only make one pass over the string.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we only need a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus