Leetcode 900: RLE Iterator
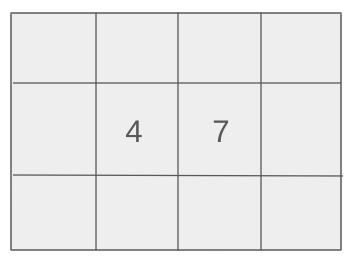
We are given a run-length encoded array where every even-indexed element specifies how many times the following odd-indexed element (a number) is repeated in the original sequence. Your task is to implement an iterator that can return the next ’n’ elements from the decoded sequence when requested.
Problem
Approach
Steps
Complexity
Input: You are given a run-length encoded array where encoding[i] gives the frequency of encoding[i+1] in the sequence. You need to create an iterator that can return the next 'n' elements when requested.
Example: Input: ['RLEIterator', 'next', 'next', 'next', 'next']
[[[2, 10, 1, 20, 3, 5]], [1], [2], [3], [4]]
Constraints:
• 2 <= encoding.length <= 1000
• encoding.length is even.
• 0 <= encoding[i] <= 10^9
• 1 <= n <= 10^9
• At most 1000 calls will be made to next.
Output: The output is the value of the last element returned after exhausting the next 'n' elements from the sequence, or -1 if there are no more elements to exhaust.
Example: Output: [null, 20, 20, 5, -1]
Constraints:
• The result for each next(n) call will be an integer or -1 if there are no more elements.
Goal: To create an iterator that processes run-length encoded data and efficiently returns the next 'n' elements in sequence.
Steps:
• 1. Use an index to traverse the run-length encoded array.
• 2. For each next(n) call, decrement the count of the remaining elements in the current sequence while keeping track of the last number encountered.
• 3. Once the count of a sequence is exhausted, move to the next run-length encoded pair and continue.
• 4. If no more elements are available, return -1.
Goal: The solution should efficiently process the next() calls and ensure that it handles large sequences correctly.
Steps:
• At most 1000 calls will be made to next.
• The input encoding array is guaranteed to have an even length.
Assumptions:
• The input encoding array will always have an even length.
• Each encoding pair will be a valid representation of a sequence of numbers.
• The solution should efficiently handle repeated calls to the 'next' method.
• Input: Input: ['RLEIterator', 'next', 'next', 'next', 'next']
[[[2, 10, 1, 20, 3, 5]], [1], [2], [3], [4]]
• Explanation: The run-length encoded array [2, 10, 1, 20, 3, 5] represents the sequence [10, 10, 20, 5, 5, 5]. Calling next(1) returns 20, next(2) returns 20 again, next(3) returns 5, and next(4) returns -1 as the sequence is exhausted.
Approach: The approach involves iterating over the run-length encoded array, processing each 'run' of repeated values, and returning the appropriate number of elements in each next() call.
Observations:
• Since the encoded array is compact, iterating through it and extracting the required elements should be efficient if done incrementally.
• Efficient traversal is key, and handling large values for 'n' is important to prevent timeouts or excessive memory usage.
Steps:
• 1. Initialize an index to traverse the encoded array.
• 2. For each next(n) call, keep track of how many elements need to be skipped or returned.
• 3. Once a 'run' is exhausted, move to the next element pair and repeat the process.
• 4. Return -1 if there are no more elements available to return.
Empty Inputs:
• The problem guarantees that encoding will not be empty, as the length is at least 2.
Large Inputs:
• Large inputs with values of 'n' up to 10^9 should be handled by efficiently skipping over the run-length sequences.
Special Values:
• Zero values in the encoding array should be handled gracefully.
Constraints:
• Efficient memory usage and computation time are necessary given the problem's constraints.
int idx = 0;
public:
RLEIterator(vector<int>& encoding) {
arr = encoding;
idx = 0;
}
int next(int n) {
while(idx < arr.size()) {
if(n > arr[idx]) {
n -= arr[idx];
idx += 2;
} else {
arr[idx] -= n;
return arr[idx + 1];
}
}
return -1;
}
};
/**
* Your RLEIterator object will be instantiated and called as such:
* RLEIterator* obj = new RLEIterator(encoding);
* int param_1 = obj->next(n);
1 : Variable Declaration
int idx = 0;
The variable `idx` is declared and initialized to 0, representing the current position in the encoded array.
2 : Access Specifier
public:
The access specifier `public` is used to indicate that the following methods are accessible outside the class.
3 : Constructor
RLEIterator(vector<int>& encoding) {
The constructor `RLEIterator` is defined to initialize the object with a reference to the encoded data array `encoding`.
4 : Array Initialization
arr = encoding;
The constructor initializes the member variable `arr` with the provided encoding array.
5 : Index Initialization
idx = 0;
The constructor initializes the index variable `idx` to 0, which will be used to traverse the encoding array.
6 : Constructor End
}
End of the constructor definition.
7 :
8 : Method Definition
int next(int n) {
The method `next(int n)` is defined to retrieve the next element in the RLE sequence based on the value `n`.
9 : While Loop
while(idx < arr.size()) {
A while loop starts, which continues as long as the index `idx` is less than the size of the array `arr`.
10 : Condition Check
if(n > arr[idx]) {
The condition checks if the remaining value `n` is greater than the current frequency value `arr[idx]`.
11 : Subtract Frequency
n -= arr[idx];
If `n` is greater than the current frequency, subtract the current frequency `arr[idx]` from `n` and move to the next pair in the encoding.
12 : Index Update
idx += 2;
The index `idx` is updated to the next element in the encoding (which is a pair of frequency and value, so the index moves by 2).
13 : Else Condition
} else {
If `n` is not greater than the current frequency `arr[idx]`, the method proceeds to return the value corresponding to this frequency.
14 : Update Frequency
arr[idx] -= n;
The frequency at the current index is reduced by `n`, which represents the number of times this value has been accessed.
15 : Return Value
return arr[idx + 1];
The value corresponding to the current frequency (i.e., `arr[idx + 1]`) is returned.
16 : End Else Block
}
End of the else block.
17 : End While Loop
}
End of the while loop.
18 : Return -1
return -1;
If no value can be returned (i.e., the encoding has been exhausted), the function returns -1.
19 : Method End
}
End of the `next` method.
20 : Class End
};
End of the `RLEIterator` class definition.
21 :
22 : Documentation
/**
The following section provides documentation on how to instantiate and use the `RLEIterator` class.
23 : Instantiation Example
* Your RLEIterator object will be instantiated and called as such:
Example usage of the `RLEIterator` class.
24 : Object Creation
* RLEIterator* obj = new RLEIterator(encoding);
This line shows how to instantiate an `RLEIterator` object with a given encoding vector.
25 : Method Call
* int param_1 = obj->next(n);
This line shows how to call the `next` method to retrieve the next element in the sequence.
Best Case: O(1) per next() call when there are enough elements left in the current 'run'.
Average Case: O(1) per next() call, assuming the sequence is processed efficiently.
Worst Case: O(n) per next() call in extreme cases when 'n' is large and needs to exhaust multiple 'runs'.
Description: In the worst case, each next() call may require iterating over several elements of the encoded array.
Best Case: O(1) as the iterator operates in constant space.
Worst Case: O(1) as no additional space is required beyond storing the encoded array.
Description: The space complexity is constant, as we only need space to store the input and an index to traverse it.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus