Leetcode 893: Groups of Special-Equivalent Strings
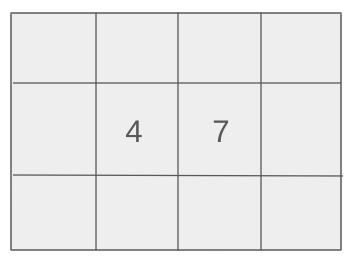
You are given an array of strings where each string is of the same length. In one move, you can swap any two characters at even indexed positions or any two characters at odd indexed positions in a string. Two strings are considered special-equivalent if they can be made identical after performing any number of such moves. Your task is to determine how many distinct groups of special-equivalent strings exist in the given array.
Problem
Approach
Steps
Complexity
Input: The input consists of an array of strings, all of which have the same length. Each string contains only lowercase English letters.
Example: Input: words = ["hello","oellh","elhol","aabb","bbaa","abab"]
Constraints:
• 1 <= words.length <= 1000
• 1 <= words[i].length <= 20
• All strings have the same length.
• Each string contains lowercase English letters.
Output: Return the number of distinct groups of special-equivalent strings in the given array.
Example: Output: 3
Constraints:
• The output should be the number of distinct groups of special-equivalent strings.
Goal: The goal is to classify the strings into distinct groups where all strings within a group are special-equivalent.
Steps:
• For each string in the array, split the characters into two groups: one for even indices and one for odd indices.
• Sort both groups (even indexed characters and odd indexed characters) to standardize their structure.
• Use a set to store each string's standardized representation (sorted even and odd indexed groups).
• The number of distinct groups will be equal to the size of the set.
Goal: The constraints ensure that the solution should be efficient enough to handle up to 1000 strings with a maximum length of 20.
Steps:
• The input will always consist of lowercase English letters.
• All the strings will be of the same length.
Assumptions:
• The problem guarantees that the input strings have the same length.
• Input: Input: words = ["hello","oellh","elhol","aabb","bbaa","abab"]
• Explanation: In this case, the strings 'hello', 'oellh', and 'elhol' are all special-equivalent to each other because their even and odd indexed characters can be rearranged to match. The strings 'aabb', 'bbaa', and 'abab' form another group since they can be rearranged similarly. Thus, there are 3 groups in total.
• Input: Input: words = ["abcd", "dcab", "acbd", "xyzz", "zzxy"]
• Explanation: In this example, the first three strings form one group as they are special-equivalent. The last two strings form another group as they can be made identical by rearranging even and odd indexed characters.
Approach: To solve this problem, we need to identify groups of strings that are special-equivalent. This can be achieved by separating each string into even and odd indexed characters, sorting them, and using a set to track distinct combinations.
Observations:
• We can separate the characters in the string based on their indices (even or odd).
• Sorting the characters within each group (even and odd) helps in identifying equivalence.
• We can use a set data structure to track unique combinations of sorted even and odd indexed characters.
Steps:
• 1. For each word, separate its characters into two groups: one for even indexed characters and one for odd indexed characters.
• 2. Sort both groups individually.
• 3. Store the tuple of the sorted even and odd groups in a set.
• 4. The number of distinct groups is the size of the set.
Empty Inputs:
• If the input is empty, the result is 0.
Large Inputs:
• Ensure the solution can handle up to 1000 strings efficiently.
Special Values:
• Strings with only one character will always form their own group.
Constraints:
• Ensure efficient handling of strings up to length 20 and 1000 words.
int numSpecialEquivGroups(vector<string>& words) {
set<pair<string, string>> s;
for(const auto& w: words) {
pair<string, string> p;
for(int i = 0; i < w.size(); i++) {
if (i % 2) p.first += w[i];
else p.second += w[i];
}
sort(p.first.begin(), p.first.end());
sort(p.second.begin(), p.second.end());
s.insert(p);
}
return s.size();
}
1 : Function Definition
int numSpecialEquivGroups(vector<string>& words) {
Define the function `numSpecialEquivGroups`, which takes a vector of strings as input and returns the number of unique special equivalent groups.
2 : Variable Declaration
set<pair<string, string>> s;
Declare a set `s` to store pairs of sorted odd and even indexed characters from each string.
3 : Loop Through Words
for(const auto& w: words) {
Iterate through each string `w` in the input vector `words`.
4 : Pair Initialization
pair<string, string> p;
Initialize a pair `p` to store the sorted characters from the odd and even indices of the string.
5 : Character Grouping
for(int i = 0; i < w.size(); i++) {
Iterate through each character in the string `w`.
6 : Odd Index Character Grouping
if (i % 2) p.first += w[i];
For characters at odd indices, append them to `p.first`.
7 : Even Index Character Grouping
else p.second += w[i];
For characters at even indices, append them to `p.second`.
8 : End Inner Loop
}
End the loop that processes characters in the string `w`.
9 : Sorting Odd Group
sort(p.first.begin(), p.first.end());
Sort the characters stored in `p.first` (odd indexed characters).
10 : Sorting Even Group
sort(p.second.begin(), p.second.end());
Sort the characters stored in `p.second` (even indexed characters).
11 : Insert Pair into Set
s.insert(p);
Insert the sorted pair `p` into the set `s` to ensure uniqueness of the combinations.
12 : End Outer Loop
}
End the loop that processes each word in the `words` vector.
13 : Return Unique Group Count
return s.size();
Return the size of the set `s`, which contains the number of unique special equivalent groups.
14 : End Function
}
End the function definition.
Best Case: O(n * m log m)
Average Case: O(n * m log m)
Worst Case: O(n * m log m)
Description: The time complexity is O(n * m log m), where n is the number of strings, and m is the length of each string. Sorting the even and odd indexed characters in each string takes O(m log m).
Best Case: O(n)
Worst Case: O(n * m)
Description: The space complexity is O(n * m) because we store each string's even and odd indexed characters separately, along with the set of unique combinations.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus