Leetcode 885: Spiral Matrix III
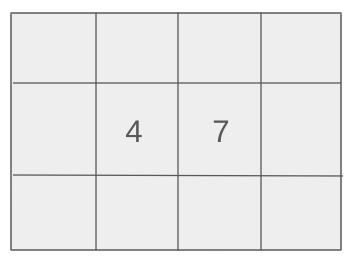
You are given a grid of size rows x cols, where each cell represents a coordinate. You start at a given coordinate (rStart, cStart), facing east, and your goal is to walk in a clockwise spiral through the grid. As you walk, you must visit each cell exactly once. Once you reach the boundary of the grid, continue walking outside the grid, but you may return to the grid later. Return a list of the coordinates you visit in the order you encounter them.
Problem
Approach
Steps
Complexity
Input: You are given the dimensions of the grid (rows, cols) and your starting position (rStart, cStart).
Example: Input: rows = 3, cols = 3, rStart = 1, cStart = 1
Constraints:
• 1 <= rows, cols <= 100
• 0 <= rStart < rows
• 0 <= cStart < cols
Output: You should return a list of coordinates, where each coordinate is represented as an array [r, c], in the order they were visited.
Example: Output: [[1,1],[1,2],[0,2],[0,1],[0,0],[1,0],[2,0],[2,1],[2,2]]
Constraints:
• The output should contain all coordinates visited in the spiral traversal.
Goal: The goal is to simulate the spiral traversal, carefully switching directions and ensuring no cell is skipped.
Steps:
• Start from the given position and begin moving east.
• Continue spiraling in clockwise direction, changing direction after hitting a boundary or previously visited cell.
• Use a list to keep track of the coordinates you visit.
Goal: The problem must be solved efficiently within the constraints.
Steps:
• The grid size is not larger than 100x100.
• You must return all coordinates in the order they are visited.
Assumptions:
• The grid has at least one cell.
• You can go outside the grid and return later when needed.
• Input: Input: rows = 3, cols = 3, rStart = 1, cStart = 1
• Explanation: The grid is 3x3 with the start position at (1,1). The spiral traversal starts at (1,1), moves east to (1,2), then north to (0,2), then west to (0,1), then south to (1,0), and continues until all cells are visited. The output is the list of coordinates visited in this order.
• Input: Input: rows = 2, cols = 2, rStart = 0, cStart = 0
• Explanation: The grid is 2x2 with the start position at (0,0). The spiral traversal visits (0,0), moves east to (0,1), then south to (1,1), and finally west to (1,0).
Approach: The problem can be solved by simulating the spiral movement using direction changes. The directions follow a clockwise pattern: east -> south -> west -> north. After each move, check if the new position is within the grid's bounds. If it is, add the position to the result. If not, change direction.
Observations:
• The movement follows a strict clockwise pattern and will continue until all cells are visited.
• The algorithm should efficiently track the positions visited while respecting the boundaries and changing directions accordingly.
Steps:
• Initialize the current position as the starting point.
• Set the direction to east (right).
• Simulate movement, changing direction after each boundary or previously visited cell is encountered.
• Keep track of visited coordinates and add them to the result list.
Empty Inputs:
• The problem constraints guarantee that there will always be at least one row and one column.
Large Inputs:
• The algorithm must handle grids as large as 100x100 efficiently.
Special Values:
• The starting point could be in any cell within the grid.
Constraints:
• The grid size and position constraints are relatively small, so a direct simulation approach will work efficiently.
vector<vector<int>> spiralMatrixIII(int rows, int cols, int rStart, int cStart) {
vector<vector<int>> res = {{rStart, cStart}};
int dx = 0, dy = 1, tmp;
for(int n = 0; res.size() < rows* cols; n++) {
for(int i = 0; i < n / 2 + 1; i++) {
rStart += dx, cStart += dy;
if(rStart >= 0 && rStart < rows && cStart >= 0 && cStart < cols)
res.push_back({rStart, cStart});
}
tmp = dx, dx = dy, dy = -tmp;
}
return res;
}
1 : Function Definition
vector<vector<int>> spiralMatrixIII(int rows, int cols, int rStart, int cStart) {
This line defines the function `spiralMatrixIII` which takes the grid dimensions (`rows`, `cols`) and the starting position (`rStart`, `cStart`) as parameters.
2 : Initialization
vector<vector<int>> res = {{rStart, cStart}};
A result vector `res` is initialized with the starting position (`rStart`, `cStart`). This will store the coordinates as the spiral is generated.
3 : Variable Setup
int dx = 0, dy = 1, tmp;
Two variables `dx` and `dy` are initialized to control the movement direction in the grid. `dx` controls row movement (initially 0), and `dy` controls column movement (initially 1). `tmp` is used for swapping direction.
4 : Loop Setup
for(int n = 0; res.size() < rows* cols; n++) {
The loop continues until all positions in the grid are covered, and `res.size()` is less than the total number of cells (`rows * cols`).
5 : Inner Loop Setup
for(int i = 0; i < n / 2 + 1; i++) {
The inner loop iterates through each direction step (n / 2 + 1 times). This determines how far to move in the current direction.
6 : Position Update
rStart += dx, cStart += dy;
The current position (`rStart`, `cStart`) is updated by adding `dx` to `rStart` and `dy` to `cStart` to move in the current direction.
7 : Boundary Check
if(rStart >= 0 && rStart < rows && cStart >= 0 && cStart < cols)
The function checks if the new position is within the bounds of the grid. If valid, it proceeds to add the new position to the result.
8 : Position Add to Result
res.push_back({rStart, cStart});
If the new position is valid, it is added to the result vector `res`.
9 : Direction Update
tmp = dx, dx = dy, dy = -tmp;
This line swaps the direction of movement by rotating `dx` and `dy`. This ensures the spiral continues in the correct order (right, down, left, up).
10 : Return Statement
return res;
The function returns the `res` vector containing all coordinates in the spiral order.
Best Case: O(n * m)
Average Case: O(n * m)
Worst Case: O(n * m)
Description: In the worst case, the algorithm needs to visit all cells in the grid, which takes O(n * m) time where n is the number of rows and m is the number of columns.
Best Case: O(n * m)
Worst Case: O(n * m)
Description: The space complexity is O(n * m) because we need to store all the visited coordinates.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus