Leetcode 877: Stone Game
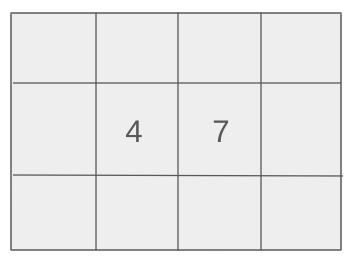
Alice and Bob are playing a game with an array of piles of stones. Each pile contains a positive number of stones, and the game proceeds as follows: Alice and Bob take turns, with Alice starting first. On each turn, a player can choose the entire pile of stones either from the beginning or the end of the array. The player who ends up with the most stones wins. Given the piles array, return true if Alice wins the game, or false if Bob wins, assuming both players play optimally.
Problem
Approach
Steps
Complexity
Input: You are given an array of integers representing piles of stones. The length of the array is even, and the sum of the stones in all piles is odd.
Example: Input: piles = [4, 6, 2, 8]
Constraints:
• 2 <= piles.length <= 500
• piles.length is even.
• 1 <= piles[i] <= 500
• sum(piles[i]) is odd.
Output: Return true if Alice wins, meaning she accumulates more stones than Bob. Otherwise, return false.
Example: Output: true
Constraints:
• The output is a boolean indicating if Alice wins the game.
Goal: The goal is to determine if Alice, starting first, can win the game by optimally picking stones from the piles.
Steps:
• Use dynamic programming to simulate both players' optimal moves.
• Memoize the results to avoid recalculating the same state.
• For each state, choose the pile from either the start or the end, and subtract the value of the opponent's optimal play.
Goal: The solution must account for optimal moves and the constraints on the length and values of the piles.
Steps:
• The list of piles contains an even number of elements.
• The sum of the stones across all piles is odd.
Assumptions:
• Both Alice and Bob play optimally, meaning they always make the best possible move.
• The game is played to completion with no interruptions.
• Input: Input: piles = [4, 6, 2, 8]
• Explanation: In this example, Alice can start by taking the last pile (8), leaving Bob with the choice of taking 4 or 2. No matter what Bob does, Alice will win by selecting optimally.
• Input: Input: piles = [3, 7, 2, 3]
• Explanation: Alice can start by taking the first pile (3), and based on Bob's choices, Alice will be able to win by making optimal selections.
Approach: We use dynamic programming to solve this problem by simulating both Alice's and Bob's moves, taking into account all possible choices they can make. The solution involves recursion and memoization to optimize the state evaluations.
Observations:
• The optimal strategy for both players is to always choose the pile that maximizes their advantage, either from the start or the end.
• Using memoization will help avoid redundant calculations and improve efficiency, especially given the problem constraints.
Steps:
• Define a recursive function with two indices, i and j, representing the current range of piles.
• Memoize the results to store already computed subproblems.
• For each subproblem, compute the optimal value by choosing the maximum stones between picking the first or the last pile.
Empty Inputs:
• An empty input should not occur as the problem guarantees an even number of piles.
Large Inputs:
• The solution should efficiently handle the upper limit of 500 piles.
Special Values:
• If the piles contain large numbers or small numbers, the solution should still be optimal, considering the memoization approach.
Constraints:
• Ensure that the solution works within the provided constraints, including the odd sum condition.
vector<int> p;
int n;
map<int, map<int,int>> memo;
bool dp(int i, int j) {
if(i == j) return p[i];
if(memo.count(i) && memo[i].count(j)) return memo[i][j];
return memo[i][j] = max(p[i] - dp(i + 1, j), p[j] - dp(i, j - 1)) ;
}
bool stoneGame(vector<int>& piles) {
this->p = piles;
n = piles.size();
return dp(0, n - 1) >= 0;
}
1 : Variable Initialization
vector<int> p;
This initializes a vector `p` to store the piles of stones.
2 : Variable Initialization
int n;
This initializes an integer `n` to store the number of piles.
3 : Memoization Map
map<int, map<int,int>> memo;
This initializes a memoization map `memo` to store previously computed results for subproblems in the dynamic programming solution.
4 : Function Definition
bool dp(int i, int j) {
This defines the recursive `dp` function that computes the maximum score difference from the subarray of piles between indices `i` and `j`.
5 : Base Case
if(i == j) return p[i];
This is the base case of the recursion. If there is only one pile left (i.e., `i == j`), return the value of that pile.
6 : Memoization Check
if(memo.count(i) && memo[i].count(j)) return memo[i][j];
This checks if the result for the subarray between indices `i` and `j` has already been computed. If so, return the memoized result.
7 : Recursive Calculation
return memo[i][j] = max(p[i] - dp(i + 1, j), p[j] - dp(i, j - 1));
This calculates the result for the current subarray by recursively calling `dp` for the two possible choices: taking the first pile or taking the last pile, and storing the result in `memo[i][j]`.
8 : Main Function
bool stoneGame(vector<int>& piles) {
This defines the main function `stoneGame` which initializes the vector `p` with the piles and calculates the number of piles `n`. It then calls the `dp` function to determine if the first player can win.
9 : Variable Assignment
this->p = piles;
This assigns the input vector `piles` to the class member `p`.
10 : Variable Assignment
n = piles.size();
This assigns the size of the input vector `piles` to the integer `n`.
11 : Return Statement
return dp(0, n - 1) >= 0;
This calls the `dp` function with the full range of the array (from 0 to `n - 1`) and returns whether the first player can win (i.e., the score difference is greater than or equal to 0).
Best Case: O(n^2)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The time complexity is O(n^2) due to the memoized recursive calls for each subproblem, where n is the number of piles.
Best Case: O(n^2)
Worst Case: O(n^2)
Description: The space complexity is O(n^2) due to the memoization table used to store the results of subproblems.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus