Leetcode 870: Advantage Shuffle
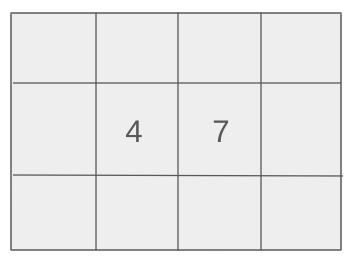
Given two integer arrays
nums1
and nums2
of the same length, the goal is to permute nums1
in a way that maximizes the number of indices i
where nums1[i] > nums2[i]
. Return any permutation of nums1
that achieves this.Problem
Approach
Steps
Complexity
Input: Two integer arrays `nums1` and `nums2`, each containing `n` elements.
Example: Input: nums1 = [1,3,5,7], nums2 = [3,6,8,10]
Constraints:
• 1 <= nums1.length <= 10^5
• nums2.length == nums1.length
• 0 <= nums1[i], nums2[i] <= 10^9
Output: Return any permutation of `nums1` such that the number of indices `i` where `nums1[i] > nums2[i]` is maximized.
Example: Output: [1,7,5,3]
Constraints:
• The output should be a valid permutation of `nums1`.
Goal: Maximize the number of indices `i` where `nums1[i] > nums2[i]` by reordering `nums1`.
Steps:
• Sort `nums1` and `nums2` while keeping track of the original indices of `nums2`.
• For each element in `nums2`, find the smallest element in `nums1` that is greater than the current element in `nums2`.
• If such an element is found, assign it to the corresponding position in the result array, otherwise, use the smallest available element from `nums1`.
Goal: The solution should handle the given constraints efficiently.
Steps:
• 1 <= nums1.length <= 10^5
• nums2.length == nums1.length
• 0 <= nums1[i], nums2[i] <= 10^9
Assumptions:
• Both arrays `nums1` and `nums2` contain non-negative integers.
• The size of both arrays is the same and within the specified limits.
• Input: Input: nums1 = [5, 1, 8, 3], nums2 = [6, 3, 7, 2]
• Explanation: After sorting `nums1` as [1, 3, 5, 8] and `nums2` as [2, 3, 6, 7], the optimal permutation is [1, 8, 5, 3], which maximizes the number of `nums1[i] > nums2[i]`.
• Input: Input: nums1 = [2, 4, 6, 8], nums2 = [1, 5, 7, 9]
• Explanation: The optimal permutation of `nums1` is [2, 4, 6, 8], as all elements in `nums1` are greater than the corresponding elements in `nums2`.
Approach: The solution aims to reorder `nums1` to maximize the number of indices where `nums1[i] > nums2[i]`. This can be done by strategically using a greedy approach with sorting and matching elements.
Observations:
• Sorting the arrays `nums1` and `nums2` allows for efficient matching of elements.
• Greedy algorithms are suitable for maximizing the number of successful comparisons.
• By sorting `nums1` and `nums2`, we can quickly determine the best match for each element in `nums2`.
Steps:
• Sort `nums1` in ascending order and `nums2` in ascending order, keeping track of the original indices of `nums2`.
• Use a greedy approach to assign each number in `nums2` the smallest number in `nums1` that is larger than it.
• If no number in `nums1` is larger, assign the smallest remaining number from `nums1`.
Empty Inputs:
• Not applicable since both arrays will always have the same length and contain integers.
Large Inputs:
• Efficient sorting and matching should be implemented to handle arrays with up to 10^5 elements.
Special Values:
• If all elements in `nums1` are smaller than the corresponding elements in `nums2`, the output will be the sorted array `nums1`.
Constraints:
• The algorithm should work efficiently within the given constraints, particularly when handling arrays of large size.
vector<int> advantageCount(vector<int>& nums1, vector<int>& nums2) {
multiset<int> s(begin(nums1), end(nums1));
for ( auto i = 0; i < nums2.size(); i++) {
auto p = *s.rbegin() <= nums2[i] ? s.begin() : s.upper_bound(nums2[i]);
nums1[i] = *p;
s.erase(p);
}
return nums1;
}
1 : Function Definition
vector<int> advantageCount(vector<int>& nums1, vector<int>& nums2) {
Defines the `advantageCount` function which aims to reorder `nums1` to maximize its advantage over `nums2`.
2 : Multiset Initialization
multiset<int> s(begin(nums1), end(nums1));
Initializes a multiset `s` using the elements of `nums1`, which allows efficient element retrieval and removal.
3 : Loop Setup
for ( auto i = 0; i < nums2.size(); i++) {
Starts a loop to iterate over each element in `nums2`, comparing it against the elements in `nums1`.
4 : Element Selection
auto p = *s.rbegin() <= nums2[i] ? s.begin() : s.upper_bound(nums2[i]);
Selects the best candidate element from `nums1` that can beat the current element in `nums2`. It checks if the largest element in `nums1` (accessed via `s.rbegin()`) can beat the current element in `nums2`; if not, it chooses the smallest element greater than `nums2[i]`.
5 : Assignment
nums1[i] = *p;
Assigns the selected element from `nums1` to the current position in `nums1`.
6 : Erase Element
s.erase(p);
Erases the selected element from the multiset `s`, as it has already been used.
7 : Return Result
return nums1;
Returns the modified `nums1` with elements rearranged to maximize the advantage over `nums2`.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which takes O(n log n) time.
Best Case: O(n)
Worst Case: O(n)
Description: Space is used for sorting and tracking the indices, resulting in a space complexity of O(n).
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus