Leetcode 861: Score After Flipping Matrix
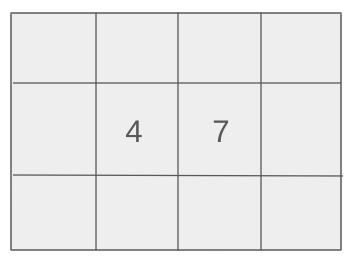
You are given a binary matrix where each row and column can be toggled independently. A toggle operation flips all values in a row or column (changing 0s to 1s and 1s to 0s). The matrix’s score is calculated by interpreting each row as a binary number and summing up these values. Your task is to determine the maximum score possible after performing any number of toggle operations.
Problem
Approach
Steps
Complexity
Input: The input is a binary matrix represented as a 2D array of integers. Each element is either 0 or 1.
Example: Input: grid = [[1,0,0],[0,1,1],[1,1,0]]
Constraints:
• m == grid.length
• n == grid[i].length
• 1 <= m, n <= 20
• grid[i][j] is either 0 or 1.
Output: Return an integer representing the highest possible score of the matrix after performing any number of toggles.
Example: Output: 14
Constraints:
Goal: To maximize the matrix's score by toggling rows and columns strategically to maximize the binary number values of each row.
Steps:
• Step 1: Ensure the first column contains all 1s by toggling any rows where the first element is 0.
• Step 2: For each remaining column, determine whether toggling the column increases the number of 1s in that column.
• Step 3: Use the column's majority value (1s or 0s) to calculate its contribution to the total score.
• Step 4: Compute the score by interpreting each row as a binary number and summing up the values.
Goal: The binary matrix and toggle operations must follow the constraints:
Steps:
• The matrix dimensions (m x n) are within the range 1 <= m, n <= 20.
• All elements in the matrix are either 0 or 1.
Assumptions:
• You can perform any number of toggle operations on rows or columns.
• No limit on the number of rows or columns toggled in a single operation.
• The input matrix contains at least one row and one column.
• Input: Input: grid = [[1,0,0],[0,1,1],[1,1,0]]
• Explanation: Toggle the second row to convert it to [1, 0, 0]. Then toggle the third column to get [[1, 0, 1], [1, 0, 1], [1, 1, 1]]. The binary representation is 0b101 (5), 0b101 (5), 0b111 (7). Total score = 5 + 5 + 7 = 17.
• Input: Input: grid = [[0,1],[1,0]]
• Explanation: Toggle the first row to make it [1, 0]. Then toggle the second column to make it [1, 1] and [1, 1]. The binary values are 0b11 (3) and 0b11 (3). Total score = 3 + 3 = 6.
Approach: A greedy approach is used to maximize the score by toggling rows and columns strategically.
Observations:
• The leftmost column has the highest weight in the binary score. Ensuring all 1s in the first column maximizes its contribution.
• For remaining columns, the contribution depends on the number of 1s. Maximizing 1s in each column maximizes the overall score.
• We can toggle rows to ensure the first column is all 1s, and then toggle columns to maximize the 1s in each column.
Steps:
• Step 1: Traverse each row. If the first element is 0, toggle the entire row to make it 1.
• Step 2: Traverse each column starting from the second. Count the number of 1s and 0s.
• Step 3: If the count of 0s is greater than 1s, toggle the column.
• Step 4: Compute the total score by interpreting each row as a binary number and summing the values.
Empty Inputs:
• N/A: The constraints guarantee at least a 1x1 matrix.
Large Inputs:
• The matrix size can be up to 20x20. Ensure the solution efficiently processes matrices of this size.
Special Values:
• If all values are 0 initially, toggling all rows first ensures the maximum score.
• If all values are 1 initially, no toggles are needed.
Constraints:
• Input matrix values must strictly be binary (0 or 1).
int matrixScore(vector<vector<int>>& grid) {
int m = grid.size(), n = grid[0].size();
int res = (1 << n - 1) * m;
for(int j = 1; j < n; j++) {
int cur = 0;
for(int i = 0; i < m; i++)
cur += grid[i][0] == grid[i][j];
res += max(cur, m - cur) * (1<<n-j-1);
}
return res;
}
1 : Function Declaration
int matrixScore(vector<vector<int>>& grid) {
The function begins by accepting a 2D vector `grid` that represents a matrix of binary values.
2 : Variable Initialization
int m = grid.size(), n = grid[0].size();
Two variables `m` and `n` are initialized to store the number of rows and columns in the grid, respectively.
3 : Initial Result Calculation
int res = (1 << n - 1) * m;
The initial score `res` is calculated based on the number of rows and the most significant bit of the matrix (represented by the left-most column).
4 : For Loop (Column Iteration)
for(int j = 1; j < n; j++) {
For each column `j`, starting from column 1, we compute the contribution of the column to the score.
5 : Variable for Current Column Score
int cur = 0;
A variable `cur` is initialized to calculate the score for the current column, based on the grid values.
6 : Inner Loop (Row Iteration)
for(int i = 0; i < m; i++)
This inner loop iterates through each row `i` of the current column `j`.
7 : Check Column Values
cur += grid[i][0] == grid[i][j];
The `cur` score is incremented if the value in the first column matches the value in the current column (`grid[i][j]`).
8 : Update Result
res += max(cur, m - cur) * (1<<n-j-1);
The result `res` is updated by adding the maximum score obtainable for the current column (either flipping the column or not) multiplied by a power of 2 corresponding to the column's position.
9 : Return Statement
return res;
Return the final calculated score after processing all columns.
Best Case: O(m * n), where no toggling is needed and only the binary computation is performed.
Average Case: O(m * n), as we toggle rows and compute column values.
Worst Case: O(m * n), as all rows and columns may need toggling.
Description:
Best Case: O(1), since space usage remains constant.
Worst Case: O(1), as no additional data structures are used apart from counters.
Description:
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus