Leetcode 844: Backspace String Compare
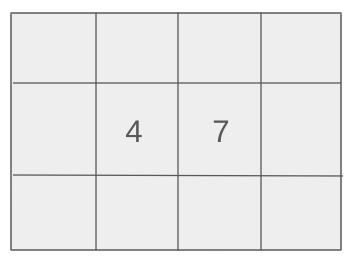
You are given an integer array
arr
. A mountain array is an array where the elements first strictly increase to a peak and then strictly decrease after that peak. Your task is to find the length of the longest mountain subarray in the given array. If no such subarray exists, return 0.Problem
Approach
Steps
Complexity
Input: You are given an integer array 'arr' where the elements are non-negative integers.
Example: Input: arr = [3, 2, 5, 6, 4, 3, 1]
Constraints:
• 1 <= arr.length <= 10^4
• 0 <= arr[i] <= 10^4
Output: Return the length of the longest mountain subarray in the given array. If no such subarray exists, return 0.
Example: Output: 5
Constraints:
• The array must have at least 3 elements to form a mountain.
Goal: To find the longest subarray that first strictly increases and then strictly decreases.
Steps:
• Step 1: Traverse through the array and find all potential peaks where the element is greater than its neighbors.
• Step 2: For each peak, expand to the left and right to determine the length of the mountain.
• Step 3: Keep track of the longest mountain found during the traversal.
Goal: Ensure the array has a valid mountain shape, with a peak element and elements that strictly increase and then strictly decrease.
Steps:
• The array must have at least 3 elements to form a valid mountain.
• The array elements must strictly increase before the peak and strictly decrease after it.
Assumptions:
• The array is not empty.
• You are working with integer values only.
• Input: Input: [3, 2, 5, 6, 4, 3, 1]
• Explanation: The longest mountain is [5, 6, 4, 3], which has length 5.
• Input: Input: [1, 2, 3, 4]
• Explanation: There is no mountain because the array only increases.
• Input: Input: [9, 8, 7]
• Explanation: There is no mountain because the array only decreases.
Approach: The approach involves scanning through the array to find peaks and expanding from those peaks to check for valid mountains. This can be done in a single pass with careful checking of elements before and after the peak.
Observations:
• The problem requires finding subarrays with a specific structure (strictly increasing and then strictly decreasing).
• We can utilize a two-pointer or sliding window technique to efficiently check for the longest mountain.
• A linear pass through the array could be sufficient if we check each element to see if it's a peak, and then expand outwards to find the boundaries of the mountain.
Steps:
• Step 1: Iterate over the array and find peaks, which are elements that are greater than their immediate neighbors.
• Step 2: For each peak, check the elements to the left and right to find the boundaries of the mountain.
• Step 3: Keep track of the longest mountain found and return its length.
Empty Inputs:
• The input cannot be empty as the array must contain at least 3 elements.
Large Inputs:
• Ensure that the solution handles large inputs efficiently, especially when the array length is at the upper constraint of 10^4.
Special Values:
• Arrays with all elements the same do not form a mountain.
• Arrays that strictly increase or strictly decrease will not form a valid mountain.
Constraints:
• Arrays with fewer than 3 elements cannot form a valid mountain.
bool backspaceCompare(string S, string T) {
int i = S.length() - 1, j = T.length() - 1, back;
while (true) {
back = 0;
while (i >= 0 && (back > 0 || S[i] == '#')) {
back += S[i] == '#' ? 1 : -1;
i--;
}
back = 0;
while (j >= 0 && (back > 0 || T[j] == '#')) {
back += T[j] == '#' ? 1 : -1;
j--;
}
if (i >= 0 && j >= 0 && S[i] == T[j]) {
i--;
j--;
} else {
break;
}
}
return i == -1 && j == -1;
}
1 : Function Definition
bool backspace Compare(string S, string T) {
Defines the function `backspace Compare` that takes two strings `S` and `T` and compares them after processing the backspaces.
2 : Variable Initialization
int i = S.length() - 1, j = T.length() - 1, back;
Initializes two pointers, `i` and `j`, to point to the end of both strings, and a variable `back` to track the number of backspaces.
3 : Loop Start
while (true) {
Starts an infinite loop to process the strings until the end of the comparison.
4 : Variable Reset
back = 0;
Resets the `back` variable to 0 at the start of each loop iteration.
5 : Backspace Processing S
while (i >= 0 && (back > 0 || S[i] == '#')) {
Processes the string `S` by skipping characters that are backspaces or undoing the effect of backspaces.
6 : Update Back
back += S[i] == '#' ? 1 : -1;
Updates the `back` variable, incrementing it when a backspace ('#') is encountered and decrementing it otherwise.
7 : Pointer Decrement S
i--;
Moves the pointer `i` to the previous character in string `S`.
8 : Variable Reset Again
back = 0;
Resets the `back` variable before processing the string `T`.
9 : Backspace Processing T
while (j >= 0 && (back > 0 || T[j] == '#')) {
Processes string `T` by skipping backspaces or undoing the effect of backspaces.
10 : Update Back Again
back += T[j] == '#' ? 1 : -1;
Updates the `back` variable for string `T`, incrementing for backspaces and decrementing otherwise.
11 : Pointer Decrement T
j--;
Moves the pointer `j` to the previous character in string `T`.
12 : Character Comparison
if (i >= 0 && j >= 0 && S[i] == T[j]) {
Compares the characters at positions `i` and `j` in `S` and `T`.
13 : Pointer Decrement Both
i--;
Moves the pointer `i` to the previous character in string `S`.
14 : Pointer Decrement Both
j--;
Moves the pointer `j` to the previous character in string `T`.
15 : Mismatch Found
} else {
Handles the case where the characters at `S[i]` and `T[j]` do not match.
16 : Exit Loop
break;
Exits the loop when a mismatch is found.
17 : Final Check
return i == -1 && j == -1;
Returns `true` if both strings `S` and `T` have been fully processed and no mismatches were found; otherwise, returns `false`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear as we make a single pass over the array, with constant time checks for each element.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant since we only need a few variables to track the peak and the longest mountain length.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus