Leetcode 3179: Find the N-th Value After K Seconds
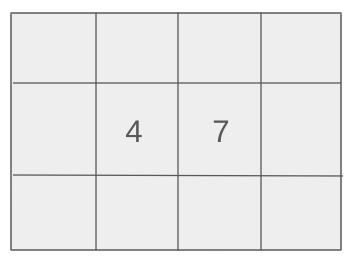
You are given two integers,
n
and k
. Initially, you have an array a
of size n
, where each element is initialized to 1. After each second, every element in the array is updated to be the sum of all preceding elements plus the element itself. The process repeats for k
seconds. You need to return the value of the last element of the array, a[n - 1]
, after k
seconds. Since the result may be very large, return it modulo (10^9 + 7).Problem
Approach
Steps
Complexity
Input: The input consists of two integers: `n` (the number of elements in the array) and `k` (the number of seconds the array undergoes updates).
Example: Example 1:
Input: n = 4, k = 3
Output: 20
Explanation: After 3 seconds, the last element becomes 20.
Constraints:
• 1 <= n, k <= 1000
Output: Return the value of the last element of the array after `k` seconds, modulo (10^9 + 7).
Example: Example 2:
Input: n = 5, k = 4
Output: 35
Explanation: After 4 seconds, the last element becomes 35.
Constraints:
• The output will be an integer modulo (10^9 + 7).
Goal: Simulate the update of the array for `k` seconds and compute the value of the last element modulo (10^9 + 7).
Steps:
• Initialize an array `a` of size `n` with all elements set to 1.
• For each second, update each element of the array such that each element becomes the sum of all preceding elements plus the element itself.
• Repeat this for `k` seconds.
• Return the value of the last element modulo (10^9 + 7).
Goal: The input should satisfy the following constraints.
Steps:
• 1 <= n <= 1000
• 1 <= k <= 1000
Assumptions:
• All elements in the array are initially set to 1.
• The number of seconds is limited to 1000, making an iterative approach feasible.
• Input: Example 1:
• Explanation: For `n = 4` and `k = 3`, the array starts as [1, 1, 1, 1]. After 1 second, the array becomes [1, 2, 3, 4]. After 2 seconds, it becomes [1, 3, 6, 10], and after 3 seconds, it becomes [1, 4, 10, 20]. Therefore, the last element after 3 seconds is 20.
• Input: Example 2:
• Explanation: For `n = 5` and `k = 4`, the array starts as [1, 1, 1, 1, 1]. After 1 second, the array becomes [1, 2, 3, 4, 5], after 2 seconds it becomes [1, 3, 6, 10, 15], after 3 seconds it becomes [1, 4, 10, 20, 35], and after 4 seconds, the array becomes [1, 5, 15, 35, 70]. Thus, the last element after 4 seconds is 35.
Approach: We will simulate the array updates for `k` seconds. After each second, every element of the array is updated based on the sum of all previous elements. This can be efficiently implemented using dynamic programming.
Observations:
• The problem can be solved through dynamic programming by iterating over the seconds and updating the array in-place.
• The last element after `k` seconds is influenced by all the previous elements.
• We need to be mindful of performance given that both `n` and `k` can go up to 1000. Using dynamic programming with a 2D array should work efficiently within these limits.
Steps:
• Initialize a 2D array `mtx` where each row represents the state of the array at a given second. The initial state is all elements set to 1.
• For each second (from 1 to k), update the elements such that `mtx[i][j] = (mtx[i-1][j] + mtx[i][j-1]) % MOD`, where `MOD = 10^9 + 7`.
• After `k` seconds, return the value of `mtx[k][n-1]`.
Empty Inputs:
• There are no empty inputs since the problem always provides values for `n` and `k`.
Large Inputs:
• The approach should handle the maximum values of `n = 1000` and `k = 1000` efficiently, using dynamic programming.
Special Values:
• For `n = 1` and `k = 1`, the result is trivially 1 because the array does not change.
Constraints:
• The solution should be able to handle the constraints up to `n = 1000` and `k = 1000` efficiently.
int mod = (int) 1e9 + 7;
int valueAfterKSeconds(int n, int k) {
vector<vector<long>> mtx(k + 1, vector<long>(n, 1));
for(int i = 1; i <= k; i++) {
for(int j = 1; j < n; j++) {
mtx[i][j] = (mtx[i - 1][j] + mtx[i][j - 1]) % mod;
}
}
return mtx[k][n - 1];
}
1 : Modulo Initialization
int mod = (int) 1e9 + 7;
The modulo value `mod` is initialized to `1e9 + 7`, a common modulus used to prevent overflow in large numbers.
2 : Function Definition
int valueAfterKSeconds(int n, int k) {
The function `valueAfterKSeconds` is defined, taking two parameters: `n` (the number of positions) and `k` (the number of seconds).
3 : Matrix Initialization
vector<vector<long>> mtx(k + 1, vector<long>(n, 1));
A 2D matrix `mtx` is initialized with dimensions `(k + 1) x n`, and all elements are set to `1`.
4 : Outer Loop Start
for(int i = 1; i <= k; i++) {
The outer loop begins, iterating over `k` seconds (from `1` to `k`).
5 : Inner Loop Start
for(int j = 1; j < n; j++) {
The inner loop starts, iterating over `n` positions (from `1` to `n-1`).
6 : Matrix Update
mtx[i][j] = (mtx[i - 1][j] + mtx[i][j - 1]) % mod;
The current matrix element `mtx[i][j]` is updated by adding the values from the previous row and the previous column, and then applying the modulo operation to avoid overflow.
7 : Return Statement
return mtx[k][n - 1];
The function returns the final value located at the `k`-th row and the `n-1`-th column of the matrix.
Best Case: O(k * n)
Average Case: O(k * n)
Worst Case: O(k * n)
Description: The time complexity is O(k * n) because we update the array for each second, and each element takes constant time to update.
Best Case: O(k * n)
Worst Case: O(k * n)
Description: The space complexity is O(k * n) due to the storage of the 2D array `mtx` used for dynamic programming.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus