Leetcode 3168: Minimum Number of Chairs in a Waiting Room
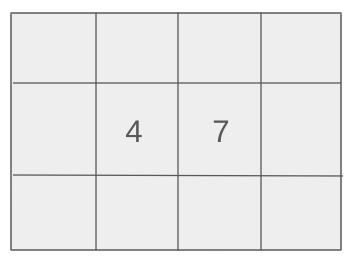
You are given a string
s
representing a sequence of events where each character is either ‘E’ (a person enters the waiting room) or ‘L’ (a person leaves the waiting room). The waiting room starts empty. Simulate the events and determine the minimum number of chairs required to ensure there is always a chair available for every person who enters the room.Problem
Approach
Steps
Complexity
Input: The input consists of a string `s` containing the characters 'E' and 'L'.
Example: Example 1:
Input: s = "LLLEEEE"
Output: 3
Constraints:
• 1 <= s.length <= 50
• s consists only of the characters 'E' and 'L'.
• s represents a valid sequence of entries and exits.
Output: Return the minimum number of chairs required to accommodate all the people entering the waiting room.
Example: Example 1:
Input: s = "LLLEEEE"
Output: 3
Constraints:
• The output will be an integer.
Goal: The goal is to track the number of people in the waiting room at any given time and determine the maximum number of chairs required at any point.
Steps:
• Initialize a counter to track the number of people in the waiting room.
• Iterate over the events in the string `s`.
• For each 'E', increment the counter (a person enters).
• For each 'L', decrement the counter (a person leaves).
• Keep track of the maximum value of the counter during the iteration. This represents the maximum number of people in the room at once, which is the minimum number of chairs needed.
Goal: The input string must meet the following constraints.
Steps:
• 1 <= s.length <= 50
• s consists only of 'E' and 'L'.
• The sequence of events is valid, with no more people leaving than those who have entered.
Assumptions:
• The sequence of entries and exits is valid (no 'L' without an 'E' before it).
• The waiting room starts with 0 people and is initially empty.
• Input: Example 1:
• Explanation: For `s = "LLLEEEE"`, at the start no one is in the room, then 3 people leave and 4 people enter, so the maximum number of people in the room at any time is 3. Therefore, the minimum number of chairs required is 3.
• Input: Example 2:
• Explanation: For `s = "ELELEEL"`, after each entry and exit, the number of people in the room fluctuates between 1 and 2. The maximum number of people in the room at any time is 2, so the minimum number of chairs needed is 2.
Approach: The approach involves iterating through the string `s` to simulate each event. We track the number of people in the waiting room at any point and compute the maximum number of people in the room, which gives the number of chairs needed.
Observations:
• We need to track the current number of people in the room and update it as we process each event.
• The approach should efficiently handle the maximum input size (length up to 50). We will simply keep a counter and update it while tracking the maximum number of people in the room.
Steps:
• Initialize a variable to track the current number of people in the waiting room.
• Initialize a variable to store the maximum number of people seen in the waiting room at any point.
• Iterate through each character in the string `s`.
• For each 'E', increment the number of people in the room.
• For each 'L', decrement the number of people in the room.
• Update the maximum number of people seen in the room as you go.
• Return the maximum value as the minimum number of chairs required.
Empty Inputs:
• There will never be an empty string as input.
Large Inputs:
• The solution should handle strings with lengths up to 50 efficiently.
Special Values:
• Ensure the solution works with edge cases such as all people leaving immediately or all people entering.
Constraints:
• Ensure that the number of chairs is calculated accurately even with mixed events of entries and exits.
int minimumChairs(string s) {
int mx = 0, cnt = 0;
for(char x: s) {
cnt += (x == 'E'? 1: -1);
mx = max(mx, cnt);
}
return mx;
}
1 : Function Definition
int minimumChairs(string s) {
Defines the function 'minimumChairs' that accepts a string 's', representing a series of events, and returns the minimum number of chairs needed.
2 : Variable Initialization
int mx = 0, cnt = 0;
Initializes two integer variables: 'mx' to store the maximum number of people present at any point, and 'cnt' to keep track of the current number of people inside.
3 : For Loop
for(char x: s) {
Begins a for loop that iterates through each character 'x' in the string 's'.
4 : Condition Check
cnt += (x == 'E'? 1: -1);
Checks if the character 'x' is 'E' (entry event), in which case it increments 'cnt' by 1. If 'x' is not 'E', it decrements 'cnt' by 1 (exit event).
5 : Max Update
mx = max(mx, cnt);
Updates the value of 'mx' to be the maximum of 'mx' and the current 'cnt', ensuring it tracks the peak number of people inside.
6 : Return Statement
return mx;
Returns the value of 'mx', which represents the maximum number of people inside at any point, i.e., the minimum number of chairs required.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the string `s`. We only need to iterate through the string once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because we are only using a constant amount of extra space for the counters.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus