Leetcode 3146: Permutation Difference between Two Strings
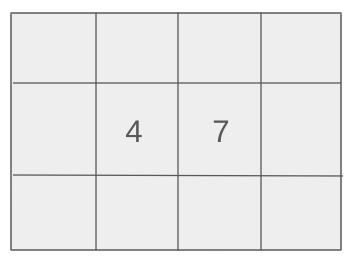
You are given two strings,
s
and t
, where every character occurs at most once in both strings and t
is a permutation of s
. The permutation difference between s
and t
is defined as the sum of the absolute differences between the index of each character in s
and the index of the occurrence of the same character in t
. Your task is to compute the permutation difference between s
and t
.Problem
Approach
Steps
Complexity
Input: The input consists of two strings `s` and `t`.
Example: Example 1:
Input: s = "xys", t = "syx"
Output: 2
Constraints:
• 1 <= s.length <= 26
• Each character occurs at most once in `s`.
• t is a permutation of `s`.
• s consists only of lowercase English letters.
Output: Return the permutation difference between `s` and `t`.
Example: Example 1:
Input: s = "xys", t = "syx"
Output: 2
Constraints:
• The output should be the sum of the absolute differences of character indices in `s` and `t`.
Goal: To compute the permutation difference, iterate over each character in `s` and find the corresponding index in `t`, then calculate the absolute difference in indices.
Steps:
• Iterate through each character in string `s`.
• For each character in `s`, find its index in string `t`.
• Compute the absolute difference between the indices of each character in `s` and `t`.
• Sum these absolute differences and return the result.
Goal: The constraints for the input strings are given below.
Steps:
• 1 <= s.length <= 26
• Each character occurs at most once in `s`.
• t is a permutation of `s`.
• s consists only of lowercase English letters.
Assumptions:
• The input strings `s` and `t` are permutations of each other.
• Each character in `s` and `t` occurs only once.
• Input: Example 1:
• Explanation: For `s = "abc"` and `t = "bac"`, the permutation difference is the sum of absolute differences between indices of characters: |0 - 1| + |1 - 0| + |2 - 2| = 2.
• Input: Example 2:
• Explanation: For `s = "abcdef"` and `t = "dfecba"`, the permutation difference is: |0 - 4| + |1 - 3| + |2 - 5| + |3 - 2| + |4 - 1| + |5 - 0| = 12.
Approach: The approach to solving this problem involves iterating through the string `s`, locating the index of each character in `t`, and calculating the sum of the absolute differences of indices.
Observations:
• The problem requires comparing character positions in two strings and calculating the sum of the differences.
• Since `t` is a permutation of `s`, the character order is shuffled, and finding the index difference will give the required result.
Steps:
• Create a hashmap or list to store the indices of characters in string `t`.
• Iterate through the characters in string `s` and for each character, find its index in `t`.
• Calculate the absolute difference between the indices and accumulate the sum.
Empty Inputs:
• Both strings `s` and `t` will never be empty.
Large Inputs:
• The solution should handle strings of length up to 26 efficiently.
Special Values:
• The solution should handle cases where characters are shifted by several positions in `t`.
Constraints:
• Ensure the solution handles cases where `s` and `t` are minimal (length 1).
int findPermutationDifference(string s, string t) {
int sum=0;
for(int i=0;i<s.length();i++)
{
for(int j=0;j<t.length();j++)
{
if(s[i]==t[j])
sum+=abs(i-j);
}
}
return sum;
}
1 : Initialization
int findPermutationDifference(string s, string t) {
Defines the function header for 'findPermutationDifference', taking two string parameters 's' and 't'.
2 : Variable Declaration
int sum=0;
Initializes a variable 'sum' to store the total of the absolute differences between the indices of matching characters.
3 : Outer Loop
for(int i=0;i<s.length();i++)
Starts the outer loop that iterates through each character in string 's'.
4 : Inner Loop
for(int j=0;j<t.length();j++)
Starts the inner loop that iterates through each character in string 't'.
5 : Condition Check
if(s[i]==t[j])
Checks if the characters at position 'i' in string 's' and position 'j' in string 't' are equal.
6 : Sum Calculation
sum+=abs(i-j);
If the characters are equal, it calculates the absolute difference between their indices and adds it to 'sum'.
7 : Return Statement
return sum;
Returns the value of 'sum', which contains the total of the absolute differences between the indices of matching characters.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear, where `n` is the length of the input strings, as each character is processed once.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to storing the indices of characters in `t`.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus