Leetcode 3120: Count the Number of Special Characters I
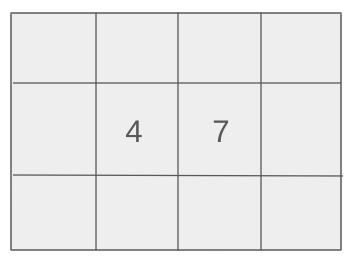
You are given a string
word
. A letter is considered special if it appears both in its lowercase and uppercase form in the string. Return the number of special letters in word
.Problem
Approach
Steps
Complexity
Input: The input consists of a string `word` of length n.
Example: word = "aAAbcdBC"
Constraints:
• 1 <= word.length <= 50
• word consists of only lowercase and uppercase English letters
Output: Return the number of special letters in the string `word`.
Example: Output: 3
Constraints:
Goal: Identify letters that appear in both lowercase and uppercase in the string and count them.
Steps:
• 1. Create a set to store unique characters in `word`.
• 2. Loop through each letter in the alphabet ('a' to 'z').
• 3. For each letter, check if both the lowercase and uppercase forms exist in the set.
• 4. Count and return the total number of special letters.
Goal: The problem constraints define the limits on the length and content of the string.
Steps:
• 1 <= word.length <= 50
• word consists of only lowercase and uppercase English letters
Assumptions:
• The input string will only contain lowercase and uppercase English letters.
• The string will have a length between 1 and 50 characters.
• Input: word = "aAAbcdBC"
• Explanation: The special characters are 'a', 'b', and 'c', as they appear in both uppercase and lowercase in `word`.
• Input: word = "abcdef"
• Explanation: No special characters exist in this string, as no letter appears in both uppercase and lowercase.
• Input: word = "AbBcC"
• Explanation: The special characters are 'b' and 'c', as they appear both in lowercase and uppercase.
Approach: We can solve the problem by using a set to store all the letters in the string and then check if both the lowercase and uppercase versions of each letter exist in the set.
Observations:
• We need to check for each letter if both its lowercase and uppercase forms exist in the string.
• By iterating through all the letters in the string, we can efficiently find special letters using a set.
Steps:
• 1. Initialize a set to store all unique characters from the string.
• 2. For each letter in the alphabet ('a' to 'z'), check if both the lowercase and uppercase versions are present in the set.
• 3. Count and return the total number of special letters.
Empty Inputs:
• The string will always have at least one character as per the constraints.
Large Inputs:
• The solution should efficiently handle strings with lengths up to 50 characters.
Special Values:
• If the string contains only lowercase or only uppercase letters, the output should be 0.
Constraints:
• The string is constrained to only contain lowercase and uppercase English letters.
int numberOfSpecialChars(string word) {
set<char> ch;
for(char x: word)
ch.insert(x);
int cnt = 0;
for(int i = 0; i < 26; i++) {
if(ch.count('a' + i) && ch.count('A' + i))
cnt++;
}
return cnt;
}
1 : Function Definition
int numberOfSpecialChars(string word) {
Defines the function `numberOfSpecialChars` that takes a string `word` as input and returns the count of special characters, where a special character is a pair of the same letter in both lowercase and uppercase.
2 : Set Initialization
set<char> ch;
Initializes a set `ch` to store unique characters from the input string `word`. Using a set ensures that each character is only stored once.
3 : Loop Through String
for(char x: word)
Iterates through each character `x` in the string `word`.
4 : Insert Character into Set
ch.insert(x);
Inserts the character `x` into the set `ch`, ensuring that only unique characters are stored.
5 : Counter Initialization
int cnt = 0;
Initializes the counter variable `cnt` to 0, which will be used to count the number of special characters (pairs of lowercase and uppercase letters).
6 : Loop Over Alphabet
for(int i = 0; i < 26; i++) {
Starts a `for` loop that iterates over the 26 letters of the alphabet (from 'a' to 'z').
7 : Check Pair of Letters
if(ch.count('a' + i) && ch.count('A' + i))
Checks if both the lowercase and uppercase versions of the letter (corresponding to `i`) are present in the set `ch`.
8 : Increment Counter
cnt++;
If both the lowercase and uppercase characters are found, increments the counter `cnt` to account for one special character.
9 : Return Result
return cnt;
Returns the value of `cnt`, which represents the total number of special characters in the string `word`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the string, since we only loop through the string once and check for each character's presence in the set.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since we only store a constant number of unique characters (26 lowercase and 26 uppercase letters).
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus