Leetcode 3110: Score of a String
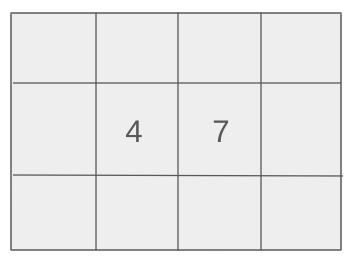
You are given a string s. The score of a string is defined as the sum of the absolute difference between the ASCII values of adjacent characters. Return the score of the string s.
Problem
Approach
Steps
Complexity
Input: The input consists of a string s of length n.
Example: s = "abc"
Constraints:
• 2 <= s.length <= 100
• s consists only of lowercase English letters
Output: Return the score of the string s.
Example: Output: 3
Constraints:
Goal: Calculate the score of the string by summing the absolute differences between ASCII values of adjacent characters.
Steps:
• 1. Initialize a variable to store the sum of absolute differences.
• 2. Loop through the string, comparing each pair of adjacent characters.
• 3. For each pair, compute the absolute difference in ASCII values and add it to the sum.
• 4. Return the total sum as the score.
Goal: The problem constraints limit the string length and characters.
Steps:
• 2 <= s.length <= 100
• s consists only of lowercase English letters
Assumptions:
• The input will always contain at least two characters.
• The string will only contain lowercase English letters.
• Input: s = "abc"
• Explanation: The ASCII values of the characters are: 'a' = 97, 'b' = 98, 'c' = 99. The score is |97 - 98| + |98 - 99| = 3.
• Input: s = "zyx"
• Explanation: The ASCII values of the characters are: 'z' = 122, 'y' = 121, 'x' = 120. The score is |122 - 121| + |121 - 120| = 6.
Approach: To solve this problem, we can iterate through the string, calculate the absolute differences between ASCII values of adjacent characters, and sum them.
Observations:
• The problem asks for a simple iteration over the string and calculation of differences.
• By iterating through the string and summing the absolute differences of ASCII values, we can compute the score efficiently.
Steps:
• 1. Initialize a variable sum to 0.
• 2. Loop through the string from index 0 to length - 2.
• 3. For each adjacent pair, calculate the absolute difference of their ASCII values and add it to sum.
• 4. Return the final sum as the score of the string.
Empty Inputs:
• The input will always contain at least two characters, as per the constraints.
Large Inputs:
• The solution should efficiently handle strings of length up to 100 characters.
Special Values:
• When the string consists of repeated characters (e.g., "aaaa"), the score will be 0.
Constraints:
• The solution must handle strings of length between 2 and 100.
int scoreOfString(string s) {
int sum=0;
for(int i=0;i<s.size()-1;i++){
sum+=abs(s[i]-s[i+1]);
}
return sum;
}
1 : Function Definition
int scoreOfString(string s) {
Defines the function `scoreOfString` that takes a string `s` and returns the total score as an integer. The score is calculated based on the absolute differences between consecutive characters.
2 : Variable Initialization
int sum=0;
Initializes the variable `sum` to 0. This variable will hold the total score of the string as the absolute differences between consecutive characters are accumulated.
3 : For Loop Setup
for(int i=0;i<s.size()-1;i++){
Starts a `for` loop that iterates through each character in the string `s`, except for the last character (since it's compared with the next one).
4 : Score Calculation
sum+=abs(s[i]-s[i+1]);
For each consecutive pair of characters, calculates the absolute difference of their ASCII values using `abs(s[i] - s[i+1])`, and adds the result to `sum`.
5 : Return Statement
return sum;
Returns the final value of `sum`, which represents the total score of the string based on the absolute differences of consecutive characters.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the string. We need to iterate through the string once to calculate the score.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since we only use a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus