Leetcode 3100: Water Bottles II
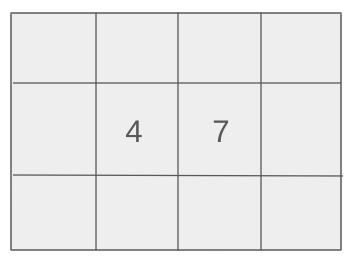
You are given two integers: numBottles, representing the number of full water bottles you initially have, and numExchange, representing the number of empty bottles required to exchange for a full bottle. In one operation, you can drink any number of full water bottles, turning them into empty bottles, or exchange numExchange empty bottles for one full bottle, with numExchange increasing by 1 after each exchange. Return the maximum number of water bottles you can drink.
Problem
Approach
Steps
Complexity
Input: The input consists of two integers: numBottles (1 <= numBottles <= 100) and numExchange (1 <= numExchange <= 100).
Example: numBottles = 12, numExchange = 4
Constraints:
• 1 <= numBottles <= 100
• 1 <= numExchange <= 100
Output: Return the maximum number of water bottles you can drink after performing all possible operations.
Example: Output: 15
Constraints:
Goal: Maximize the number of water bottles consumed by performing exchanges while possible.
Steps:
• 1. Start by drinking all the initial full bottles.
• 2. After drinking, accumulate empty bottles and attempt to exchange them for full bottles if possible.
• 3. Increase numExchange by 1 after each exchange.
• 4. Repeat the process until no further exchanges can be made.
Goal: The problem constraints are the limits on numBottles and numExchange.
Steps:
• 1 <= numBottles <= 100
• 1 <= numExchange <= 100
Assumptions:
• numBottles and numExchange will always be within the given constraints.
• Input: numBottles = 12, numExchange = 4
• Explanation: You drink 12 full bottles initially, exchange empty bottles for full bottles while possible, and keep track of the total number of bottles drunk, which amounts to 15.
• Input: numBottles = 8, numExchange = 3
• Explanation: You drink 8 full bottles initially, then exchange empty bottles for full bottles and continue until no more exchanges can be made, resulting in 10 bottles drunk.
Approach: To solve this problem, we can simulate the process of drinking and exchanging bottles, keeping track of the full and empty bottles after each operation.
Observations:
• We need to track the full and empty bottles separately, and perform exchanges while possible.
• The number of bottles drunk can be maximized by continuously performing exchanges as long as there are enough empty bottles.
Steps:
• 1. Initialize variables for full bottles, empty bottles, and total bottles drunk.
• 2. Drink all the full bottles, converting them to empty bottles.
• 3. Check if there are enough empty bottles to perform an exchange.
• 4. If possible, exchange the empty bottles for full bottles, increase numExchange, and repeat until no further exchanges can be made.
• 5. Return the total number of bottles drunk.
Empty Inputs:
• This problem doesn't have any empty inputs as numBottles and numExchange are always positive.
Large Inputs:
• The input limits of 1 <= numBottles <= 100 and 1 <= numExchange <= 100 are small, so performance is not an issue.
Special Values:
• The case where numBottles is exactly divisible by numExchange should be handled to ensure that exchanges are performed optimally.
Constraints:
• The solution should efficiently handle the given input constraints.
int maxBottlesDrunk(int bot, int ex) {
int full = bot;
int empty = 0;
int drunk = 0;
while((empty + full) >= ex) {
drunk += full;
empty += full;
full = 0;
while(empty >= ex) {
empty -= ex;
full += 1;
ex += 1;
}
}
return drunk + full;
}
1 : Function Definition
int maxBottlesDrunk(int bot, int ex) {
Defines the function `maxBottlesDrunk` that takes two integers, `bot` (the number of full bottles) and `ex` (the number of empty bottles needed to get a full bottle), and returns the total number of drunk bottles.
2 : Variable Initialization
int full = bot;
Initializes the `full` variable to the number of full bottles (`bot`).
3 : Variable Initialization
int empty = 0;
Initializes the `empty` variable to 0, which will keep track of the number of empty bottles.
4 : Variable Initialization
int drunk = 0;
Initializes the `drunk` variable to 0, which will accumulate the total number of drunk bottles.
5 : While Loop
while((empty + full) >= ex) {
Enters the `while` loop as long as the total number of full and empty bottles is greater than or equal to `ex`, the number of empty bottles needed to get one full bottle.
6 : Bottle Consumption
drunk += full;
Adds all the current full bottles to the `drunk` count, as all full bottles will be consumed.
7 : Bottle Movement
empty += full;
Adds the consumed full bottles to the `empty` count, since they are now empty after being drunk.
8 : Bottle Reset
full = 0;
Resets the `full` variable to 0, since all full bottles have been consumed.
9 : Inner While Loop Setup
while(empty >= ex) {
Enters a nested `while` loop, which continues as long as there are enough empty bottles to exchange for full ones.
10 : Empty Bottle Reduction
empty -= ex;
Reduces the `empty` count by `ex`, the number of empty bottles required to obtain one full bottle.
11 : Full Bottle Increase
full += 1;
Increases the `full` count by 1, since a new full bottle has been obtained.
12 : Empty Bottle Exchange
ex += 1;
Increases the value of `ex` (the number of empty bottles required to exchange for a full one) by 1, simulating a change in exchange rules or a progressive difficulty.
13 : Return Statement
return drunk + full;
Returns the total number of drunk bottles (`drunk`) plus any remaining full bottles (`full`).
Best Case: O(1)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the number of full bottles. This is because we simulate each drink and exchange operation.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because we use a constant amount of extra space to track the bottles.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus