Leetcode 3099: Harshad Number
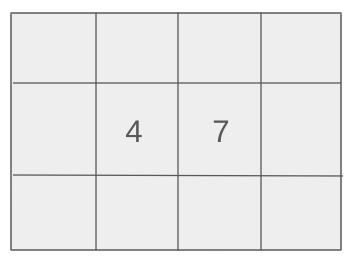
An integer is called a Harshad number if it is divisible by the sum of its digits. You are given an integer
x
. Your task is to return the sum of the digits of x
if x
is a Harshad number. Otherwise, return -1.Problem
Approach
Steps
Complexity
Input: The input consists of a single integer `x` where `1 <= x <= 100`.
Example: x = 36
Constraints:
• 1 <= x <= 100
Output: Return the sum of the digits of `x` if `x` is a Harshad number. Otherwise, return -1.
Example: Output: 9
Constraints:
Goal: To determine if the number is divisible by the sum of its digits and return the appropriate result.
Steps:
• Calculate the sum of the digits of the number `x`.
• Check if `x` is divisible by this sum.
• If divisible, return the sum of the digits. Otherwise, return -1.
Goal: The integer `x` must be between 1 and 100 inclusive.
Steps:
• 1 <= x <= 100
Assumptions:
• The input will always be a positive integer less than or equal to 100.
• Input: x = 36
• Explanation: The sum of the digits of `x` is `9`, and since `36` is divisible by `9`, the result is `9`.
• Input: x = 25
• Explanation: The sum of the digits of `x` is `7`, and since `25` is not divisible by `7`, the result is `-1`.
Approach: To solve this problem, we need to compute the sum of the digits of `x` and check if `x` is divisible by this sum.
Observations:
• We can easily calculate the sum of the digits by repeatedly extracting the last digit and adding it to the sum.
• We need to check if the number is divisible by the sum of its digits and return the sum or -1 accordingly.
Steps:
• 1. Calculate the sum of digits of `x`.
• 2. Check if `x` is divisible by the sum of its digits.
• 3. Return the sum if divisible, otherwise return -1.
Empty Inputs:
• This problem does not have any empty inputs since `x` is always a positive integer.
Large Inputs:
• The maximum value for `x` is 100, so performance will not be an issue.
Special Values:
• If `x` is a single-digit number, it will always be divisible by the sum of its digits.
Constraints:
• The solution should work efficiently for all integers in the range from 1 to 100.
int sumOfTheDigitsOfHarshadNumber(int x) {
int sum = 0;
int tmp = x;
while(tmp > 0) {
sum += tmp % 10;
tmp /= 10;
}
return x % sum == 0? sum : -1;
}
1 : Function Definition
int sumOfTheDigitsOfHarshadNumber(int x) {
Defines the function `sumOfTheDigitsOfHarshadNumber`, which takes an integer `x` and returns the sum of its digits if `x` is divisible by this sum.
2 : Variable Initialization
int sum = 0;
Initializes a variable `sum` to 0, which will be used to store the sum of the digits of `x`.
3 : Variable Initialization
int tmp = x;
Copies the value of `x` into `tmp`. `tmp` will be used for manipulating the digits of `x` without modifying the original value.
4 : Loop Setup
while(tmp > 0) {
Begins a `while` loop to process each digit of `tmp` by extracting and summing the digits until all digits are processed.
5 : Digit Extraction
sum += tmp % 10;
Extracts the last digit of `tmp` using the modulo operator (`% 10`), adds it to `sum`, and prepares for the next iteration.
6 : Digit Removal
tmp /= 10;
Removes the last digit from `tmp` by performing integer division by 10.
7 : Harshad Check
return x % sum == 0? sum : -1;
Checks if `x` is divisible by the sum of its digits. If true, returns the sum; otherwise, returns -1 to indicate that `x` is not a Harshad number.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The solution involves iterating through the digits of the number `x` once, which makes the time complexity O(1) for the given constraints.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) since we are only using a few variables for storing the sum of digits and the result.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus