Leetcode 3046: Split the Array
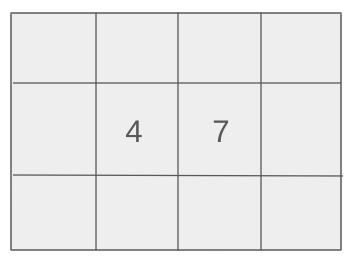
You are given an integer array ’nums’ of even length. Your task is to determine whether it is possible to divide the array into two subarrays, ’nums1’ and ’nums2’, such that both subarrays have distinct elements and the same length. Each subarray must contain half of the total elements from the original array.
Problem
Approach
Steps
Complexity
Input: You are given an array of integers nums.
Example: nums = [5, 6, 7, 7, 8, 8]
Constraints:
• 1 <= nums.length <= 100
• nums.length % 2 == 0
• 1 <= nums[i] <= 100
Output: Return true if it's possible to split the array into two subarrays with distinct elements, otherwise return false.
Example: Output: true
Constraints:
Goal: Determine if it's possible to split the given array into two subarrays with distinct elements.
Steps:
• Count the frequency of each number in the array.
• If any number appears more than twice, return false.
• If all numbers appear at most twice, return true.
Goal: Ensure the given array satisfies the constraints mentioned below.
Steps:
• nums.length is even.
• Each element is between 1 and 100.
Assumptions:
• The array has an even number of elements.
• The array elements are within the specified range.
• Input: nums = [5, 6, 7, 7, 8, 8]
• Explanation: In this case, we can split the array into nums1 = [5, 6, 7] and nums2 = [7, 8, 8]. Both subarrays have distinct elements, so the result is true.
Approach: To solve this problem, we need to check if it's possible to split the array into two subarrays with distinct elements.
Observations:
• We need to check the frequency of each number in the array.
• If a number appears more than twice, it would be impossible to split the array.
Steps:
• Iterate over the array and count the frequency of each number.
• If any number appears more than twice, return false.
• If all numbers appear at most twice, return true.
Empty Inputs:
• If nums is empty, we can return false.
Large Inputs:
• If nums has 100 elements, we need to handle it efficiently.
Special Values:
• Arrays where all numbers are the same will return false.
Constraints:
• The array must have an even length.
bool isPossibleToSplit(vector<int>& nums) {
map<int, int> mp;
for(int x: nums) {
mp[x]++;
if(mp[x] > 2) return false;
}
return true;
}
1 : Function Definition
bool isPossibleToSplit(vector<int>& nums) {
Defines the function `isPossibleToSplit` which accepts a vector of integers `nums` and returns a boolean indicating whether the array can be split with the restriction that no number appears more than twice.
2 : Variable Initialization
map<int, int> mp;
Declares a map `mp` to track the frequency of each element in the `nums` array.
3 : Loop
for(int x: nums) {
Iterates through each element `x` in the `nums` array.
4 : Map Update
mp[x]++;
Increments the count of the element `x` in the map `mp`.
5 : Condition Check
if(mp[x] > 2) return false;
Checks if any element in the array appears more than twice by comparing its count in `mp`. If true, returns `false`.
6 : Return Statement
return true;
If no element appears more than twice, returns `true` indicating the array can be split as required.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where n is the length of the array, as we are iterating over the array once.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage required for counting the frequency of elements.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus