Leetcode 3005: Count Elements With Maximum Frequency
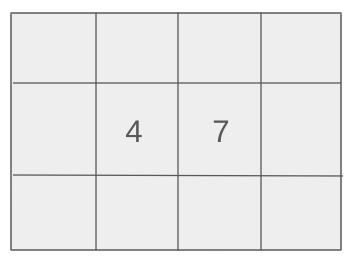
You are given an array
nums
containing positive integers. Your task is to find the total frequency of the elements in nums
that appear the maximum number of times. The frequency of an element is defined as the number of times that element occurs in the array. You need to return the sum of frequencies of all elements that have the maximum frequency.Problem
Approach
Steps
Complexity
Input: The input consists of an array `nums` of positive integers. The task is to compute the sum of the frequencies of the elements in `nums` with the maximum frequency.
Example: nums = [2, 3, 2, 4, 5, 2]
Constraints:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
Output: Return the sum of frequencies of elements in the array that have the maximum frequency.
Example: Output: 6
Constraints:
Goal: The goal is to calculate the total frequency of the elements that appear the most frequently in the array.
Steps:
• Create a frequency map to store the count of each element in the array.
• Determine the maximum frequency of any element in the array.
• Sum the frequencies of all elements that have the maximum frequency.
Goal: The array `nums` contains integers between 1 and 100, and its length is between 1 and 100.
Steps:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
Assumptions:
• The input will always have at least one element in the array.
• Input: Input: nums = [2, 3, 2, 4, 5, 2]
• Explanation: The frequency of 2 is the highest (3 occurrences). Thus, the total frequency of the element with the highest frequency is 6 (3 + 3).
• Input: Input: nums = [1, 1, 2, 2, 3, 3]
• Explanation: All elements appear twice, so the sum of the maximum frequencies is 6 (2 + 2 + 2).
• Input: Input: nums = [10, 20, 30]
• Explanation: All elements have a frequency of 1. Thus, the sum of the maximum frequencies is 3 (1 + 1 + 1).
Approach: To solve this problem, we can use a frequency map (or hash map) to count the occurrences of each element. After counting, we find the maximum frequency and sum the frequencies of all elements with that maximum count.
Observations:
• We need to efficiently count the occurrences of elements and track the maximum frequency.
• Using a frequency map allows us to count occurrences in linear time, making the solution efficient.
Steps:
• Initialize an empty frequency map.
• Iterate through the array `nums`, counting the occurrences of each element.
• Determine the maximum frequency from the frequency map.
• Sum the frequencies of all elements that have the maximum frequency.
Empty Inputs:
• Since `nums` will always have at least one element, we don't need to handle empty input.
Large Inputs:
• Ensure that the solution works efficiently for arrays of length 100, the upper constraint.
Special Values:
• If all elements are distinct, the maximum frequency is 1, and we sum the frequencies of all elements.
Constraints:
• The array contains integers between 1 and 100, and its length is between 1 and 100.
int maxFrequencyElements(vector<int>& nums) {
unordered_map<int, int> frq;
int mx = 0;
for (int num : nums) {
frq[num]++;
mx = max(mx, frq[num]);
}
int cnt = 0;
for (auto it : frq) {
if (it.second == mx)
cnt++;
}
int net = mx * cnt;
return net;
}
1 : Function Definition
int maxFrequencyElements(vector<int>& nums) {
Defines the function `maxFrequencyElements` that takes a vector of integers `nums` and returns an integer representing the product of the maximum frequency of elements in the array and the count of such elements.
2 : Data Structure Initialization
unordered_map<int, int> frq;
Initializes an unordered map `frq` to store the frequency of each element in the array `nums`.
3 : Variable Initialization
int mx = 0;
Initializes the variable `mx` to store the maximum frequency found while processing the elements of `nums`.
4 : Loop Over Elements
for (int num : nums) {
Starts a loop to iterate through each element `num` in the input vector `nums`.
5 : Update Frequency
frq[num]++;
Increments the frequency of the current element `num` in the `frq` map.
6 : Track Maximum Frequency
mx = max(mx, frq[num]);
Updates `mx` to hold the maximum frequency found so far by comparing the current frequency of `num` with the previous maximum.
7 : Initialize Count Variable
int cnt = 0;
Initializes the variable `cnt` to count how many elements have the maximum frequency `mx`.
8 : Loop Over Frequency Map
for (auto it : frq) {
Starts a loop to iterate over each key-value pair (`it`) in the frequency map `frq`.
9 : Check for Maximum Frequency
if (it.second == mx)
Checks if the frequency of the current element (`it.second`) equals the maximum frequency `mx`.
10 : Update Count
cnt++;
Increments the count `cnt` if the current element's frequency matches the maximum frequency.
11 : Calculate Result
int net = mx * cnt;
Calculates the result `net` as the product of the maximum frequency `mx` and the count `cnt` of elements with that frequency.
12 : Return Result
return net;
Returns the calculated result `net`, which represents the product of the maximum frequency and the count of elements with that frequency.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we iterate through the array once to count frequencies and once to find the maximum frequency.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the storage of frequencies in a map, where `n` is the number of unique elements in the array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus