Leetcode 2956: Find Common Elements Between Two Arrays
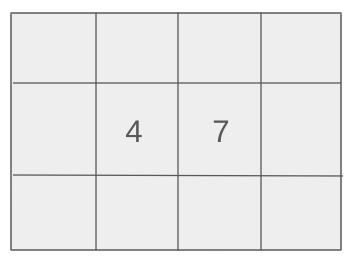
You are given two integer arrays, nums1 and nums2, with sizes n and m respectively. Your task is to find the number of indices i such that nums1[i] exists in nums2 and the number of indices i such that nums2[i] exists in nums1. Return both values as an array [answer1, answer2].
Problem
Approach
Steps
Complexity
Input: Two integer arrays nums1 and nums2.
Example: nums1 = [2, 3, 2], nums2 = [1, 2]
Constraints:
• 1 <= n, m <= 100
• 1 <= nums1[i], nums2[i] <= 100
Output: Return an array of two integers: [answer1, answer2]. answer1 is the number of elements from nums1 that are present in nums2, and answer2 is the number of elements from nums2 that are present in nums1.
Example: [2, 1]
Constraints:
Goal: To find the count of matching elements between the two arrays in both directions.
Steps:
• Convert nums1 and nums2 into sets for efficient lookup.
• Iterate through nums1 and count how many of its elements exist in nums2.
• Similarly, iterate through nums2 and count how many of its elements exist in nums1.
Goal: The arrays have a maximum size of 100 elements, and each element is between 1 and 100.
Steps:
• n == nums1.length
• m == nums2.length
• 1 <= nums1[i], nums2[i] <= 100
Assumptions:
• Both input arrays will have at least one element.
• Input: Input: nums1 = [2, 3, 2], nums2 = [1, 2]
• Explanation: The elements in nums1 that exist in nums2 are 2 and 2, so answer1 is 2. The element 2 in nums2 exists in nums1, so answer2 is 1.
Approach: The approach is to count the number of matching elements between nums1 and nums2 by using set lookups for efficient matching.
Observations:
• Using sets will allow for O(1) average-time lookups.
• We can use two sets to check how many elements from nums1 exist in nums2 and vice versa.
Steps:
• Convert both arrays into sets.
• For each element in nums1, check if it exists in nums2.
• For each element in nums2, check if it exists in nums1.
Empty Inputs:
• If one or both arrays are empty, the result will be [0, 0].
Large Inputs:
• Ensure the solution runs efficiently when n and m are at their maximum size of 100.
Special Values:
• Arrays with no common elements should return [0, 0].
Constraints:
• n and m are guaranteed to be between 1 and 100.
vector<int> findIntersectionValues(vector<int>& nums1, vector<int>& nums2) {
set<int> n1, n2;
for(int x: nums1) n1.insert(x);
for(int x: nums2) n2.insert(x);
int cnt1 = 0;
for(int x: nums1) if(n2.count(x)) cnt1++;
int cnt2 = 0;
for(int x: nums2) if(n1.count(x)) cnt2++;
return vector<int>{cnt1, cnt2};
}
1 : Function Definition
vector<int> findIntersectionValues(vector<int>& nums1, vector<int>& nums2) {
Defines the function 'findIntersectionValues', which takes two vectors of integers, 'nums1' and 'nums2', and returns a vector of integers with the counts of common elements from each array.
2 : Declare Sets
set<int> n1, n2;
Declares two sets, 'n1' and 'n2', to store the unique elements from 'nums1' and 'nums2', respectively. Sets automatically handle duplicates.
3 : Populate Set n1
for(int x: nums1) n1.insert(x);
Iterates over 'nums1' and inserts each element into the set 'n1'. This ensures all elements in 'nums1' are unique.
4 : Populate Set n2
for(int x: nums2) n2.insert(x);
Iterates over 'nums2' and inserts each element into the set 'n2'. This ensures all elements in 'nums2' are unique.
5 : Initialize Count Variables
int cnt1 = 0;
Initializes 'cnt1' to zero. This variable will keep track of the number of common elements between 'nums1' and 'nums2'.
6 : Count Common Elements from nums1
for(int x: nums1) if(n2.count(x)) cnt1++;
Iterates over 'nums1' and checks if each element exists in 'n2'. If it does, it increments 'cnt1', counting how many elements in 'nums1' are also in 'nums2'.
7 : Initialize Second Count Variable
int cnt2 = 0;
Initializes 'cnt2' to zero. This variable will keep track of the number of common elements between 'nums2' and 'nums1'.
8 : Count Common Elements from nums2
for(int x: nums2) if(n1.count(x)) cnt2++;
Iterates over 'nums2' and checks if each element exists in 'n1'. If it does, it increments 'cnt2', counting how many elements in 'nums2' are also in 'nums1'.
9 : Return Result
return vector<int>{cnt1, cnt2};
Returns a vector containing the counts of common elements: 'cnt1' (common elements from 'nums1') and 'cnt2' (common elements from 'nums2').
Best Case: O(n + m)
Average Case: O(n + m)
Worst Case: O(n + m)
Description: Converting arrays into sets takes O(n) and O(m) time, and each lookup is O(1).
Best Case: O(n + m)
Worst Case: O(n + m)
Description: The space complexity is O(n + m) due to the storage of the sets.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus