Leetcode 2919: Minimum Increment Operations to Make Array Beautiful
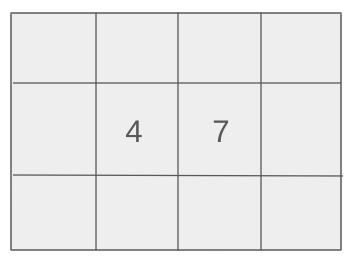
You are given a 0-indexed integer array
nums
of length n
and an integer k
. You can perform an operation where you pick an index i
in the range [0, n - 1]
and increment nums[i]
by 1. You can perform this operation any number of times (including zero). A subarray is considered beautiful if, for every subarray of size 3 or more, the maximum element in that subarray is greater than or equal to k
. Your task is to return the minimum number of increment operations needed to make the array beautiful.Problem
Approach
Steps
Complexity
Input: The input consists of a 0-indexed integer array `nums` and an integer `k`.
Example: nums = [4, 1, 0, 3, 0], k = 6
Constraints:
• 3 <= n == nums.length <= 10^5
• 0 <= nums[i] <= 10^9
• 0 <= k <= 10^9
Output: Return the minimum number of increment operations needed to make the array beautiful.
Example: Output: 4
Constraints:
• The output must be an integer.
Goal: We need to increment values in the array such that for every subarray of size 3 or more, the maximum value is greater than or equal to `k`.
Steps:
• For each element in the array, check how many increments are needed to make it greater than or equal to `k`.
• For each element, determine the minimum number of operations required to ensure all subarrays with size 3 or more satisfy the condition.
Goal: The constraints are based on the lengths of the arrays and the values of the elements.
Steps:
• 3 <= nums.length <= 10^5
• 0 <= nums[i] <= 10^9
• 0 <= k <= 10^9
Assumptions:
• The array has at least three elements.
• The value of `k` is non-negative.
• Input: Input: nums = [4, 1, 0, 3, 0], k = 6
• Explanation: We perform the minimum number of increment operations to ensure that all subarrays of size 3 or more have a maximum value of at least 6. The answer is 4.
Approach: The problem can be solved by iterating over the array and counting the minimum number of operations required to ensure that every subarray of size 3 or more has a maximum value that meets or exceeds `k`.
Observations:
• The solution needs to minimize the number of operations while ensuring the condition is met for every subarray of size 3 or more.
• We can achieve this by iterating over the array and performing the minimum number of increments necessary to make every element greater than or equal to `k` where required.
Steps:
• Iterate over the array `nums`.
• For each element, if it's smaller than `k`, calculate how many increments are required to make it greater than or equal to `k`.
• Keep track of the total number of increments needed.
Empty Inputs:
• The input will always contain at least three elements.
Large Inputs:
• The solution should be optimized to handle up to 10^5 elements efficiently.
Special Values:
• When `k` is 0, no increments are needed if all elements are already non-negative.
Constraints:
• We assume that the constraints are satisfied as given.
long long minIncrementOperations(vector<int>& A, int k) {
long long dp1 = 0, dp2 = 0, dp3 = 0, dp;
for (int& a: A) {
dp = min(dp1, min(dp2, dp3)) + max(k - a, 0);
dp1 = dp2;
dp2 = dp3;
dp3 = dp;
}
return min(dp1, min(dp2, dp3));
}
1 : Function Definition
long long minIncrementOperations(vector<int>& A, int k) {
Defines the function 'minIncrementOperations' which takes an array 'A' and an integer 'k', returning the minimum number of operations to make each element of the array greater than or equal to 'k'.
2 : Variable Initialization
long long dp1 = 0, dp2 = 0, dp3 = 0, dp;
Initializes four long long variables: dp1, dp2, dp3 for dynamic programming state storage and dp for the current operation calculation.
3 : Loop Over Array
for (int& a: A) {
Iterates through the array 'A', using reference to modify each element if necessary.
4 : Dynamic Programming Update
dp = min(dp1, min(dp2, dp3)) + max(k - a, 0);
Calculates the minimum number of operations needed by considering the previous dynamic programming states (dp1, dp2, dp3) and the difference between 'k' and the current element 'a'.
5 : State Transition
dp1 = dp2;
Updates dp1 to the value of dp2 for the next iteration, preparing for the dynamic programming transition.
6 : State Transition
dp2 = dp3;
Updates dp2 to the value of dp3 for the next iteration, continuing the dynamic programming state transition.
7 : State Transition
dp3 = dp;
Sets dp3 to the current value of dp, completing the state transition for dynamic programming.
8 : Return Result
return min(dp1, min(dp2, dp3));
Returns the minimum value among dp1, dp2, and dp3, which represents the minimum number of operations needed to make each element of 'A' greater than or equal to 'k'.
Best Case: O(n), where n is the length of the array.
Average Case: O(n)
Worst Case: O(n)
Description: We iterate over the array once, performing constant-time operations for each element.
Best Case: O(1)
Worst Case: O(1), since no extra space is used beyond a few variables.
Description: The solution uses constant space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus