Leetcode 2910: Minimum Number of Groups to Create a Valid Assignment
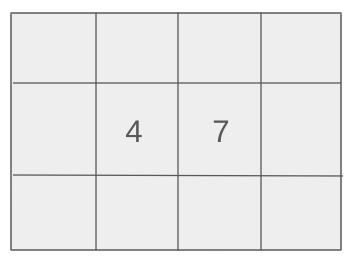
You are given a collection of balls, each marked with a number. Your task is to sort the balls into boxes in a way that follows two rules: Balls with the same number must be placed in the same box, but if there are multiple balls with the same number, they can be placed in different boxes. Additionally, the biggest box can only have one more ball than the smallest box. Return the fewest number of boxes required to sort these balls while following these rules.
Problem
Approach
Steps
Complexity
Input: You are given an array of integers, where each integer represents a ball. The array can have up to 10^5 elements.
Example: balls = [4, 4, 4, 2, 1, 1, 5]
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
Output: Return the fewest number of boxes required to sort the balls into according to the given rules.
Example: For the input [4, 4, 4, 2, 1, 1, 5], the output should be 4.
Constraints:
Goal: The goal is to find the minimum number of boxes that can accommodate the balls while adhering to the constraints on box sizes and ball distribution.
Steps:
• Count the occurrences of each number in the balls array.
• Determine the smallest number of boxes required by calculating how many groups can be formed where the group sizes differ by at most 1.
• Return the minimum number of groups (boxes) required to sort all balls according to the rules.
Goal: The size of the input array is within reasonable limits for processing in linear time.
Steps:
• The number of elements in the array will be at least 1 and at most 10^5.
• Each element in the array will be between 1 and 10^9.
Assumptions:
• The input will always contain at least one ball.
• It is guaranteed that the number of balls is non-negative and the input is well-formed.
• Input: balls = [4, 4, 4, 2, 1, 1, 5]
• Explanation: In this example, we need 4 boxes: one for the balls marked '4', one for the balls marked '2', one for the balls marked '1', and one for the ball marked '5'. The total number of boxes is 4.
Approach: We aim to find the fewest number of boxes that can accommodate the balls by analyzing their frequencies and balancing the distribution of balls across the boxes.
Observations:
• The problem boils down to partitioning the balls into groups where each group can fit into a box, and the size of the boxes can differ by at most one ball.
• By considering the frequency of each ball number and calculating how to evenly distribute them across boxes, we can minimize the number of boxes required.
Steps:
• Count the frequency of each ball number.
• Determine the smallest group size that satisfies the box size constraint.
• Calculate the minimum number of groups by balancing the distribution of balls into as few boxes as possible.
Empty Inputs:
• The input will always have at least one ball, so there will never be an empty input.
Large Inputs:
• The solution should handle large inputs efficiently, up to 10^5 balls.
Special Values:
• Consider cases where all balls are the same, or where there is only one ball with no other numbers present.
Constraints:
• Input values for each ball will be between 1 and 10^9.
int split(unordered_map<int, int> &cnt, int g1) {
int groups = 0, g = g1 - 1;
for (const auto &[_, c] : cnt) {
int group_cnt = c / g1, last_group = c % g1;
if (last_group && last_group + group_cnt < g)
return INT_MAX;
groups += group_cnt + (last_group > 0);
}
return groups;
}
int minGroupsForValidAssignment(vector<int>& nums) {
unordered_map<int, int> cnt;
for (auto n : nums)
++cnt[n];
int g = min_element(begin(cnt), end(cnt), [](const auto &p1, const auto &p2){
return p1.second < p2.second;})->second;
for (; split(cnt, g + 1) == INT_MAX; --g) ;
return split(cnt, g + 1);
}
};
1 : Function Definition
int split(unordered_map<int, int> &cnt, int g1) {
Defines the `split` function, which calculates the minimum number of groups based on the counts of elements in the `cnt` unordered map and a given group size `g1`.
2 : Variable Initialization
int groups = 0, g = g1 - 1;
Initializes two variables: `groups` to track the total number of groups and `g` to represent the target group size minus one.
3 : Loop: Iterate over Element Counts
for (const auto &[_, c] : cnt) {
Iterates through each element and its count (`c`) in the `cnt` unordered map.
4 : Calculate Group Counts
int group_cnt = c / g1, last_group = c % g1;
Calculates the number of full groups (`group_cnt`) that can be formed from the count `c` and determines the remaining elements (`last_group`) that don't fill a full group.
5 : Conditional Check: Valid Grouping
if (last_group && last_group + group_cnt < g)
Checks if the remaining elements (`last_group`) plus the full groups (`group_cnt`) are less than the target group size `g`. If so, returns `INT_MAX` to indicate an invalid grouping.
6 : Return on Invalid Grouping
return INT_MAX;
Returns `INT_MAX` when the grouping is invalid, which indicates that the current group size `g1` is not possible.
7 : Update Group Count
groups += group_cnt + (last_group > 0);
Updates the `groups` count by adding the number of full groups (`group_cnt`) and an additional group if there are any remaining elements (`last_group > 0`).
8 : Return Group Count
return groups;
Returns the total number of groups after iterating through all the elements in `cnt`.
9 : Function Definition
int minGroupsForValidAssignment(vector<int>& nums) {
Defines the `minGroupsForValidAssignment` function, which calculates the minimum number of groups needed for a valid assignment of elements in the array `nums`.
10 : Variable Initialization
unordered_map<int, int> cnt;
Initializes an unordered map `cnt` to store the count of each element in the array `nums`.
11 : Counting Elements
for (auto n : nums)
Loops through each element `n` in the array `nums`.
12 : Increment Count
++cnt[n];
Increments the count of the element `n` in the unordered map `cnt`.
13 : Find Minimum Element Count
int g = min_element(begin(cnt), end(cnt), [](const auto &p1, const auto &p2){
Finds the element with the minimum count in the unordered map `cnt` using a lambda function to compare the counts.
14 : End of Min Element Count
return p1.second < p2.second;})->second;
Completes the finding of the minimum element count and assigns it to the variable `g`.
15 : Loop: Adjust Group Size
for (; split(cnt, g + 1) == INT_MAX; --g) ;
Starts a loop that decreases `g` until a valid group size is found. It calls the `split` function to check if the current group size (`g + 1`) is valid.
16 : Return Final Group Count
return split(cnt, g + 1);
Returns the minimum number of groups for a valid assignment by calling the `split` function with the final valid group size `g + 1`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the number of balls, as we only need to pass through the array a constant number of times.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) because we store the count of each ball number in a map.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus