Leetcode 2905: Find Indices With Index and Value Difference II
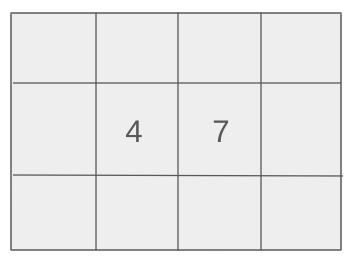
You are given an integer array nums of size n, an integer indexDifference, and an integer valueDifference. Your task is to find two indices i and j that satisfy the following conditions:
- abs(i - j) >= indexDifference,
- abs(nums[i] - nums[j]) >= valueDifference.
Return the indices [i, j] if such a pair exists, or [-1, -1] if no such pair exists. If there are multiple pairs, any valid pair is acceptable.
Problem
Approach
Steps
Complexity
Input: You are given an integer array nums, a positive integer indexDifference, and a positive integer valueDifference.
Example: nums = [7, 3, 8, 2], indexDifference = 2, valueDifference = 4
Constraints:
• 1 <= n == nums.length <= 10^5
• 0 <= nums[i] <= 10^9
• 0 <= indexDifference <= 10^5
• 0 <= valueDifference <= 10^9
Output: Return a two-element array [i, j] if there are indices i and j that satisfy the conditions. If no such pair exists, return [-1, -1].
Example: [0, 3]
Constraints:
• The answer should contain two indices, either valid or [-1, -1].
Goal: To find indices i and j such that the absolute differences between their indices and values meet the specified conditions.
Steps:
• 1. Iterate through the array with two pointers (i and j).
• 2. Check if the difference between the indices and the values meets the criteria.
• 3. Return the valid indices as soon as found, otherwise return [-1, -1].
Goal: The length of nums is between 1 and 100,000, with individual values ranging from 0 to 1 billion. indexDifference and valueDifference range from 0 to 100,000 and 0 to 1 billion respectively.
Steps:
• n, the length of the array, can go up to 10^5.
• nums[i] can be very large, up to 10^9.
Assumptions:
• The input will always be a valid array with non-negative integers.
• Both indexDifference and valueDifference are non-negative.
• Input: Input: nums = [7, 3, 8, 2], indexDifference = 2, valueDifference = 4
• Explanation: The pair (0, 3) satisfies abs(i - j) >= 2 and abs(nums[i] - nums[j]) >= 4, hence the valid pair is [0, 3].
Approach: The problem can be approached by iterating through the array with two pointers, keeping track of the indices that satisfy the difference conditions.
Observations:
• We need to check if both the index difference and value difference conditions are met.
• It’s crucial to maintain two pointers to check for the conditions efficiently.
Steps:
• 1. Initialize two pointers i and j.
• 2. Check if abs(i - j) >= indexDifference and abs(nums[i] - nums[j]) >= valueDifference.
• 3. Return the first valid pair found or [-1, -1] if no such pair exists.
Empty Inputs:
• Input arrays of size 1.
Large Inputs:
• Input arrays of size 10^5.
Special Values:
• IndexDifference or ValueDifference being 0.
Constraints:
• Both nums[i] and indexDifference can have very large values.
vector<int> findIndices(vector<int>& nums, int idif, int vdif) {
int mn = 0, mx = 0;
for (int i = idif, j = 0; i < nums.size(); ++i, ++j) {
mn = nums[mn] < nums[j] ? mn : j;
mx = nums[mx] > nums[j] ? mx : j;
if (abs(nums[i] - nums[mn]) >= vdif)
return {mn, i};
if (abs(nums[i] - nums[mx]) >= vdif)
return {mx, i};
}
return {-1, -1};
}
1 : Function Definition
vector<int> findIndices(vector<int>& nums, int idif, int vdif) {
Defines the function `findIndices` which takes a vector `nums`, an index `idif`, and a difference value `vdif`, and returns a pair of indices where the absolute difference between the values at those indices is at least `vdif`.
2 : Variable Initialization
int mn = 0, mx = 0;
Initializes two integer variables `mn` and `mx` to store the indices of the minimum and maximum values in the window being examined.
3 : Loop Initialization
for (int i = idif, j = 0; i < nums.size(); ++i, ++j) {
Starts a loop to iterate through the `nums` vector, with `i` as the index starting from `idif`, and `j` as the index of the current element in the window.
4 : Compare Min
mn = nums[mn] < nums[j] ? mn : j;
Updates the `mn` variable to the index of the smaller value between the current `mn` and `j`. This tracks the minimum value in the window.
5 : Compare Max
mx = nums[mx] > nums[j] ? mx : j;
Updates the `mx` variable to the index of the larger value between the current `mx` and `j`. This tracks the maximum value in the window.
6 : Condition Check for Min
if (abs(nums[i] - nums[mn]) >= vdif)
Checks if the absolute difference between the current element `nums[i]` and the minimum value `nums[mn]` is greater than or equal to `vdif`.
7 : Return Indices for Min
return {mn, i};
If the condition is true, returns the indices of the minimum value and the current index `i`.
8 : Condition Check for Max
if (abs(nums[i] - nums[mx]) >= vdif)
Checks if the absolute difference between the current element `nums[i]` and the maximum value `nums[mx]` is greater than or equal to `vdif`.
9 : Return Indices for Max
return {mx, i};
If the condition is true, returns the indices of the maximum value and the current index `i`.
10 : Return Default Indices
return {-1, -1};
If no valid pair of indices is found, returns `{-1, -1}` to indicate no solution.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: We iterate through the array once, so the time complexity is O(n).
Best Case: O(1)
Worst Case: O(1)
Description: We use a constant amount of space to store variables for tracking the indices.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus