Leetcode 2895: Minimum Processing Time
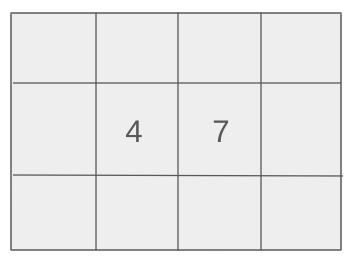
You are given multiple processors with 4 cores each and tasks that need to be assigned to these processors. The goal is to compute the minimum time needed to complete all tasks, where the time taken by a processor is determined by the maximum completion time of its assigned tasks.
Problem
Approach
Steps
Complexity
Input: You are given two arrays: processorTime and tasks.
Example: processorTime = [5, 10], tasks = [1, 2, 3, 4, 6, 7, 2, 3]
Constraints:
• 1 <= n == processorTime.length <= 25000
• 1 <= tasks.length <= 10^5
• tasks.length == 4 * n
Output: Return the minimum time required to complete all tasks, where the time is determined by the maximum time a processor finishes its assigned tasks.
Example: For input processorTime = [5, 10], tasks = [1, 2, 3, 4, 6, 7, 2, 3], the output is 16.
Constraints:
Goal: The goal is to assign tasks to processors and calculate the minimum total time required to complete all tasks.
Steps:
• Sort both the processorTime array and the tasks array.
• Assign the most time-consuming tasks to the processors in a way that minimizes the total time.
• Calculate the maximum time taken by each processor and return the maximum of those times.
Goal: The solution must efficiently handle large inputs.
Steps:
• 1 <= n == processorTime.length <= 25000
• 1 <= tasks.length <= 10^5
• tasks.length == 4 * n
Assumptions:
• The processorTime array is already sorted or can be sorted efficiently.
• The number of tasks is always a multiple of the number of processors.
• Input: For input processorTime = [5, 10], tasks = [1, 2, 3, 4, 6, 7, 2, 3], the output is 16.
• Explanation: Tasks are assigned to processors such that the total time is minimized. The first processor will finish its tasks at time 16, and the second processor will finish at time 14. The minimum time is 16.
Approach: The approach involves sorting the processors and tasks, and assigning the tasks in a way that minimizes the overall completion time.
Observations:
• The number of tasks is fixed at 4 times the number of processors.
• We need to minimize the time by assigning tasks to processors optimally.
• Sorting both the processors and tasks can help in assigning the largest tasks to processors in a way that minimizes the total time.
Steps:
• Sort the processorTime array in ascending order.
• Sort the tasks array in descending order.
• Assign the largest tasks to the processors sequentially.
• For each processor, calculate the maximum time to finish its assigned tasks and return the maximum of all processors' times.
Empty Inputs:
• The processorTime and tasks arrays should never be empty due to the problem's constraints.
Large Inputs:
• The solution must efficiently handle the maximum constraints of up to 25000 processors and 100000 tasks.
Special Values:
• Handle cases where processors have very high or very low availability times compared to the tasks.
Constraints:
• Ensure that the approach works for cases where processorTime and tasks arrays are large.
int minProcessingTime(vector<int>& t, vector<int>& v) {
int n=v.size();
sort(t.begin(),t.end());
sort(v.begin(),v.end());
int j=n-1;
int m=t.size();
int ans=0;
for(int i=0;i<m;i++)
{
int c=0;
while(c<4)
{
ans=max(ans,t[i]+v[j]);
c++;
j--;
}
}
return ans;
}
1 : Function Definition
int minProcessingTime(vector<int>& t, vector<int>& v) {
Define the function that calculates the minimum processing time by sorting and pairing elements from two vectors.
2 : Variable Initialization
int n=v.size();
Get the size of the vector `v` and store it in variable `n`.
3 : Sorting
sort(t.begin(),t.end());
Sort the vector `t` in ascending order.
4 : Sorting
sort(v.begin(),v.end());
Sort the vector `v` in ascending order.
5 : Variable Initialization
int j=n-1;
Initialize `j` to the last index of vector `v`.
6 : Variable Initialization
int m=t.size();
Get the size of the vector `t` and store it in variable `m`.
7 : Variable Initialization
int ans=0;
Initialize `ans` to 0. This will store the maximum processing time.
8 : For Loop
for(int i=0;i<m;i++)
Start a loop to iterate over the elements of vector `t`.
9 : Variable Initialization
int c=0;
Initialize the counter variable `c` to 0, which will be used for the inner while loop.
10 : While Loop
while(c<4)
Start a while loop that runs 4 times for each element in `t`.
11 : Max Function
ans=max(ans,t[i]+v[j]);
Calculate the sum of the current elements `t[i]` and `v[j]`, and update `ans` if the sum is larger.
12 : Counter Update
c++;
Increment the counter variable `c`.
13 : Pointer Update
j--;
Decrement the pointer `j` to move to the next element in vector `v`.
14 : Return Statement
return ans;
Return the calculated maximum processing time stored in `ans`.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is O(n log n) due to the sorting of processorTime and tasks arrays.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) as we store both the processorTime and tasks arrays.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus