Leetcode 2874: Maximum Value of an Ordered Triplet II
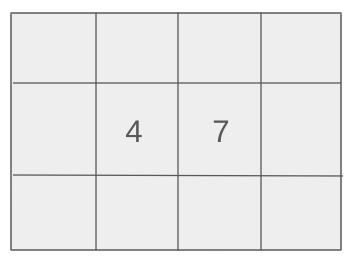
You are given an array nums of integers. Find the maximum value over all possible triplets of indices (i, j, k) such that i < j < k. The value of a triplet (i, j, k) is calculated as (nums[i] - nums[j]) * nums[k]. If all triplets produce a negative value, return 0.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array nums where 3 <= nums.length <= 10^5, and 1 <= nums[i] <= 10^6.
Example: nums = [5, 3, 2, 4, 6]
Constraints:
• 3 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^6
Output: Return the maximum value over all triplets of indices (i, j, k) such that i < j < k. If all values are negative, return 0.
Example: For input nums = [5, 3, 2, 4, 6], the output is 24.
Constraints:
Goal: The goal is to maximize the value of (nums[i] - nums[j]) * nums[k] for triplets (i, j, k) where i < j < k.
Steps:
• Iterate through each element in the array.
• For each element, calculate the value of all possible triplets using it as i, j, or k.
• Track the maximum value found during the iteration.
• Return the maximum value or 0 if no valid triplet exists.
Goal: The solution must handle arrays with lengths up to 100,000 and values up to 1 million efficiently.
Steps:
• 3 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^6
Assumptions:
• The array has at least 3 elements.
• Input: For input nums = [5, 3, 2, 4, 6], the output is 24.
• Explanation: The triplet (0, 2, 4) results in the maximum value (5 - 2) * 6 = 24.
Approach: To solve this problem, we need to iterate through all possible triplets and calculate their values. The maximum value can then be returned.
Observations:
• The problem requires examining every triplet (i, j, k).
• The values of the triplets depend on the subtraction of nums[i] and nums[j] multiplied by nums[k].
• Instead of checking all triplets brute force, we can iterate through the array while keeping track of the maximum possible values efficiently.
Steps:
• Initialize variables to keep track of the maximum values.
• For each element in the array, calculate the potential value for all possible triplets with that element.
• Update the maximum value whenever a higher value is found.
• Return the maximum value or 0 if no valid triplet exists.
Empty Inputs:
• The input will always have at least 3 elements, so no need to handle empty inputs.
Large Inputs:
• Ensure that the solution efficiently handles arrays with up to 100,000 elements.
Special Values:
• Consider cases where no valid triplet results in a non-negative value.
Constraints:
• The algorithm must run efficiently even for the largest inputs.
long long maximumTripletValue(vector<int>& nums) {
long res = 0, n = nums.size();
long maxa = 0, maxab = 0;
for(int a: nums) {
res = max(res, 1L * maxab * a);
maxab = max(maxab, (long) maxa - a);
maxa = max(maxa, (long)a);
}
return res;
}
1 : Function Declaration
long long maximumTripletValue(vector<int>& nums) {
This line declares the function, which takes a vector of integers 'nums' as input and returns a long integer representing the maximum triplet value.
2 : Variable Initialization
long res = 0, n = nums.size();
Here, 'res' is initialized to 0, which will store the maximum triplet value, and 'n' is set to the size of the input vector 'nums'.
3 : Variable Initialization
long maxa = 0, maxab = 0;
'maxa' stores the maximum value seen so far during the iteration, and 'maxab' stores the maximum value of (maxa - current element). Both are initialized to 0.
4 : Loop Setup
for(int a: nums) {
A for loop is initiated to iterate over each element 'a' in the input vector 'nums'.
5 : Max Calculation
res = max(res, 1L * maxab * a);
The result 'res' is updated by comparing its current value with the product of 'maxab' and the current element 'a'. The multiplication is done with 1L to ensure that the result is calculated as a long integer.
6 : Update maxab
maxab = max(maxab, (long) maxa - a);
The 'maxab' variable is updated to store the maximum value between its current value and the difference between 'maxa' and the current element 'a'. The cast to 'long' ensures proper handling of integer overflow.
7 : Update maxa
maxa = max(maxa, (long)a);
'maxa' is updated to hold the maximum value encountered so far, which is the greater of its current value or the current element 'a'.
8 : Return Result
return res;
After iterating through all elements, the final result 'res', which holds the maximum triplet value, is returned.
Best Case: O(n)
Average Case: O(n^2)
Worst Case: O(n^2)
Description: The time complexity is O(n^2) because we are considering all possible triplets.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we use a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus