Leetcode 2871: Split Array Into Maximum Number of Subarrays
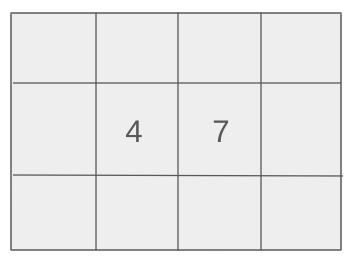
You are given an array nums consisting of non-negative integers. Your task is to divide the array into subarrays such that the sum of the scores of the subarrays is minimized. The score of a subarray nums[l..r] is defined as nums[l] AND nums[l + 1] AND … AND nums[r], where AND is the bitwise AND operation. Your goal is to return the maximum number of subarrays that you can split the array into while achieving the minimum possible sum of scores.
Problem
Approach
Steps
Complexity
Input: The input consists of an array of non-negative integers nums. You need to divide the array into subarrays while minimizing the sum of their scores.
Example: nums = [3, 0, 5, 0, 3, 5]
Constraints:
• 1 <= nums.length <= 105
• 0 <= nums[i] <= 106
Output: Return the maximum number of subarrays that you can split the array into while minimizing the sum of the subarray scores.
Example: For input nums = [3, 0, 5, 0, 3, 5], the output is 3.
Constraints:
Goal: The goal is to find the maximum number of subarrays that can be formed while achieving the minimum score for the entire array.
Steps:
• Initialize the variable to track the current AND result for the subarray.
• Iterate over the array and continuously apply the AND operation until a subarray with a score of 0 is found.
• Once a subarray with score 0 is found, split the array and reset the AND result for the next subarray.
• Track the number of subarrays formed and ensure that the score sum is minimized.
Goal: The solution must handle arrays of size up to 100,000 and element values up to 1,000,000 efficiently.
Steps:
• 1 <= nums.length <= 105
• 0 <= nums[i] <= 106
Assumptions:
• The array will have at least one element.
• Elements are non-negative integers.
• Input: For input nums = [3, 0, 5, 0, 3, 5], the output is 3.
• Explanation: We can split the array into three subarrays with scores [0, 0, 1], minimizing the sum of scores.
Approach: The problem requires minimizing the sum of scores of subarrays formed by dividing the original array. This can be achieved by leveraging the bitwise AND operation to split the array into the maximum number of subarrays.
Observations:
• The AND operation makes the score reduce or stay the same as we include more elements in the subarray.
• By continuously performing the AND operation and resetting when the score reaches 0, we can maximize the number of subarrays.
Steps:
• Initialize a counter for the subarrays and a variable to keep track of the current AND result.
• Iterate through the array, updating the AND result for the current subarray.
• When the AND result becomes 0, split the subarray and count it as a valid subarray.
• Continue the process until all elements are processed.
Empty Inputs:
• The array will always have at least one element.
Large Inputs:
• The algorithm should efficiently handle arrays up to 100,000 elements.
Special Values:
• An array where all elements are identical may require fewer subarrays.
Constraints:
• The solution must handle large arrays efficiently.
int maxSubarrays(vector<int>& nums) {
int res = 0, cur = 0;
for (int n : nums) {
cur = cur == 0 ? n : cur & n;
res += cur == 0;
}
return max(1, res);
}
1 : Function Declaration
int maxSubarrays(vector<int>& nums) {
Function declaration, taking a vector of integers 'nums' as input, and returning the maximum number of subarrays.
2 : Variable Initialization
int res = 0, cur = 0;
Initialize variables: 'res' for the result and 'cur' for the current bitwise AND value.
3 : Loop Setup
for (int n : nums) {
Iterate through each element 'n' in the input vector 'nums'.
4 : Bitwise AND Operation
cur = cur == 0 ? n : cur & n;
If 'cur' is 0, set it to the current element 'n'. Otherwise, perform a bitwise AND operation between 'cur' and 'n'.
5 : Count Subarrays
res += cur == 0;
If the result of the AND operation is 0, increment the 'res' counter, indicating a valid subarray.
6 : Return Result
return max(1, res);
Return the maximum value between 1 and the result, ensuring at least 1 subarray is counted.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we iterate through the array once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we use a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus