Leetcode 2869: Minimum Operations to Collect Elements
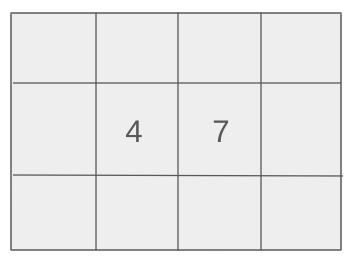
You are given an array nums containing positive integers and an integer k. In each operation, you can remove the last element from the array and add it to your collection. Your task is to determine the minimum number of operations needed to collect all elements from 1 to k (inclusive).
Problem
Approach
Steps
Complexity
Input: The input consists of an array of positive integers nums and an integer k. The goal is to collect elements from 1 to k in the minimum number of operations.
Example: nums = [6, 3, 8, 5, 7, 1, 4, 2], k = 3
Constraints:
• 1 <= nums.length <= 50
• 1 <= nums[i] <= nums.length
• 1 <= k <= nums.length
Output: Return the minimum number of operations needed to collect all elements from 1 to k in the collection.
Example: For input nums = [6, 3, 8, 5, 7, 1, 4, 2], k = 3, the output is 6.
Constraints:
Goal: The goal is to collect all numbers from 1 to k in the least number of operations.
Steps:
• Start removing elements from the end of the array one by one.
• Keep track of the elements you collect in your collection.
• Stop when all elements from 1 to k are collected.
Goal: The solution must handle arrays with up to 50 elements and work for all possible values of k.
Steps:
• 1 <= nums.length <= 50
• 1 <= nums[i] <= nums.length
• 1 <= k <= nums.length
Assumptions:
• The input array nums contains all the elements from 1 to k at least once.
• Input: For input nums = [6, 3, 8, 5, 7, 1, 4, 2], k = 3, the output is 6.
• Explanation: After 6 operations, elements 1, 2, and 3 will be collected. Thus, the total number of operations is 6.
Approach: We will process the array from the last element towards the first, collecting elements until we have all the elements from 1 to k in our collection.
Observations:
• The challenge is to determine how many operations it takes to collect the elements from 1 to k, starting from the end of the array.
• One efficient way to approach this is by using a set or array to keep track of the elements that have been collected.
Steps:
• Initialize a set to keep track of collected elements.
• Iterate over the array from the end, collecting elements.
• Stop when all elements from 1 to k are in the set.
Empty Inputs:
• The input array will always contain at least one element.
Large Inputs:
• The solution must efficiently handle arrays of size up to 50 elements.
Special Values:
• If all elements are in order or all elements from 1 to k are already in the array, fewer operations will be needed.
Constraints:
• The solution must respect the constraints on the number of elements and the values of k.
int minOperations(vector<int>& nums, int k) {
int cnt[51] = {}, i = nums.size() - 1;
for (int found = 0; found < k; --i)
if (nums[i] <= k)
found += cnt[nums[i]]++ == 0;
return nums.size() - i - 1;
}
1 : Code Declaration
int minOperations(vector<int>& nums, int k) {
Function declaration that takes a vector of integers and an integer k as input, returning an integer result.
2 : Variable Initialization
int cnt[51] = {}, i = nums.size() - 1;
Initialize an array to count occurrences of numbers up to 50 and set the index variable i to the last element of nums.
3 : Loop Setup
for (int found = 0; found < k; --i)
Start a for loop that iterates backwards through the array, with a stopping condition based on found elements.
4 : Condition Check
if (nums[i] <= k)
Condition that checks if the current element is less than or equal to k.
5 : Frequency Count
found += cnt[nums[i]]++ == 0;
Increment the 'found' variable if the current number has not been seen before, while updating its count in the cnt array.
6 : Result Calculation
return nums.size() - i - 1;
Return the difference between the size of the nums vector and the current index, minus one, which represents the result of the operation.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we only need to iterate through the array once.
Best Case: O(k)
Worst Case: O(k)
Description: The space complexity is O(k) for storing the collected elements in the set.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus