Leetcode 2860: Happy Students
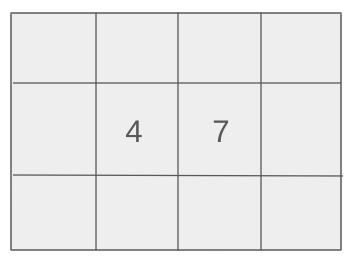
You are given a list of integers where each integer represents a student’s happiness threshold. The task is to determine the number of ways to select a group of students so that all students in the group remain happy.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer list representing the happiness thresholds of each student.
Example: nums = [2, 2]
Constraints:
• 1 <= nums.length <= 10^5
• 0 <= nums[i] < nums.length
Output: Return the number of valid ways to select students such that all students are happy.
Example: Output: 3
Constraints:
• The output is an integer representing the number of valid student selection ways.
Goal: Determine the number of ways to select a group of students such that all students in the group are happy.
Steps:
• Sort the input list to facilitate easier comparison of thresholds.
• Iterate through the list while tracking the number of students selected.
• Check if the current number of selected students satisfies the happiness condition for the student at each index.
• Count the valid groupings based on these conditions.
Goal: Constraints for the input list and the happiness threshold of each student.
Steps:
• The list contains between 1 and 100,000 students.
• Each student's threshold is between 0 and the number of students.
Assumptions:
• The input list will not be empty.
• Each student's happiness threshold is valid according to the given constraints.
• Input: nums = [2, 2]
• Explanation: The valid ways to select students are: select no student, select the first student, select the second student, or select both students.
• Input: nums = [1, 0, 3, 2, 1, 4]
• Explanation: The valid ways to select students are: select no students, select the second student only, select students with indices 1 and 2, or select all students.
Approach: The solution involves iterating through the list of students, sorting the thresholds, and using a simple check to count the valid ways to form groups where all students are happy.
Observations:
• We need to find all subsets of students where the selected students' count meets the happiness conditions for each student.
• Sorting the list helps in efficiently finding the valid groups by comparing thresholds with the current count of selected students.
Steps:
• Sort the list of students based on their happiness thresholds.
• Initialize a variable to track the number of selected students.
• Iterate through the sorted list and check the happiness conditions for each student.
• Count and return the number of valid ways to select the students.
Empty Inputs:
• The list will not be empty based on the given constraints.
Large Inputs:
• The solution should efficiently handle lists with up to 100,000 students.
Special Values:
• When all students have a threshold of 0, there are many possible ways to select groups.
Constraints:
• The input list must contain between 1 and 100,000 students.
int countWays(vector<int>& nums) {
sort(nums.begin(),nums.end());
int ans = 0, n = nums.size();
int selected = 0;
if(nums[0]!=0) ans = 1; // Not Selecting AnyOne
for(int i=0;i<n;i++) {
selected++;
if(selected>nums[i]) { // No. of Selected Students is strictly greater than nums[i].
if(i+1<n && selected<nums[i+1]) // Considering from (i+1) to n Students is not Selected.
ans++;
else if(i+1==n) ans++; // Last Student
}
}
return ans;
}
1 : Function Definition
int countWays(vector<int>& nums) {
Defines the function `countWays`, which accepts a vector `nums` and returns the number of valid selections of students.
2 : Sorting
sort(nums.begin(),nums.end());
Sorts the array `nums` in ascending order to facilitate the selection process based on increasing thresholds.
3 : Variable Initialization
int ans = 0, n = nums.size();
Initializes `ans` to 0 for counting valid selections and `n` to store the size of the `nums` array.
4 : Selection Counter
int selected = 0;
Initializes the variable `selected` to keep track of the number of selected students.
5 : Initial Condition
if(nums[0]!=0) ans = 1; // Not Selecting AnyOne
Checks if the first element in the sorted `nums` is not zero, and if so, it sets `ans` to 1, indicating a valid selection (not selecting anyone).
6 : Main Loop
for(int i=0;i<n;i++) {
Starts a loop that iterates through each student represented by the array `nums`.
7 : Increment Selection
selected++;
Increments the `selected` counter for each iteration to represent selecting a student.
8 : Condition Check
if(selected>nums[i]) { // No. of Selected Students is strictly greater than nums[i].
Checks if the number of selected students is strictly greater than the threshold value `nums[i]`.
9 : Next Student Check
if(i+1<n && selected<nums[i+1]) // Considering from (i+1) to n Students is not Selected.
Checks if the next student in the array is not selected and the selected count is still valid.
10 : Valid Selection
ans++;
Increments the answer `ans` if the selection condition is satisfied.
11 : Last Student Check
else if(i+1==n) ans++; // Last Student
Increments the answer `ans` if the current student is the last one in the array, meaning the selection is valid.
12 : Return Statement
return ans;
Returns the final count of valid ways to select students.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: The time complexity is dominated by the sorting step, which is O(n log n), where n is the number of students.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space required to store the sorted list of students.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus