Leetcode 2856: Minimum Array Length After Pair Removals
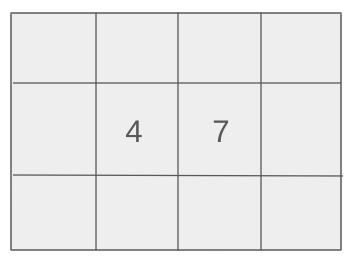
You are given a non-decreasing sorted integer array. Perform operations to remove pairs of elements where nums[i] < nums[j] and return the minimum length of the array after the operations.
Problem
Approach
Steps
Complexity
Input: The input consists of a sorted list of integers.
Example: nums = [1, 2, 3, 4]
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
• nums is sorted in non-decreasing order.
Output: Return the minimum length of the array after applying the operations.
Example: Output: 0
Constraints:
• The output is an integer, representing the remaining array length.
Goal: Determine the minimum length of the array after applying zero or more operations to remove pairs.
Steps:
• Count the frequency of each element in the array.
• Identify the largest frequency of any element.
• Based on the frequency, determine how many elements can be removed.
• Return the minimum remaining length based on the possible operations.
Goal: Constraints for the input array.
Steps:
• The length of the array is between 1 and 100,000.
• The elements of the array are distinct and fall within the range of 1 to 1,000,000,000.
Assumptions:
• The input array is sorted in non-decreasing order.
• Only valid pairs can be removed (i.e., nums[i] < nums[j]).
• Input: nums = [1, 2, 3, 4]
• Explanation: All elements can be removed in pairs, leaving the array empty.
• Input: nums = [1, 1, 2, 2, 3, 3]
• Explanation: All elements can be removed in pairs, leaving the array empty.
• Input: nums = [1000000000, 1000000000]
• Explanation: Both elements are equal, so no pairs can be removed, and the array remains with 2 elements.
• Input: nums = [2, 3, 4, 4, 4]
• Explanation: One element can be removed (either 2 or 3), leaving one element.
Approach: The approach is to count the frequency of each element and decide how many pairs can be removed based on the largest frequencies.
Observations:
• The problem can be reduced to counting frequencies and checking how many pairs can be removed.
• If no valid pairs exist (i.e., all elements are the same), the answer will be the size of the array.
• The key insight is that elements with the same value cannot form a valid pair. The task is to find how many pairs can be removed by checking frequencies.
Steps:
• Count the frequency of each element.
• Find the maximum frequency of any element.
• If the maximum frequency is greater than half the length of the array, the remaining length will be determined based on that frequency.
• Return the minimum remaining length after removing valid pairs.
Empty Inputs:
• If the input array is empty, the result is 0.
Large Inputs:
• For large inputs, ensure that counting frequencies and removing elements is efficient.
Special Values:
• If all elements are the same, no pairs can be removed, and the array will remain unchanged.
Constraints:
• The array must contain distinct integers, sorted in non-decreasing order.
int minLengthAfterRemovals(vector<int>& nums) {
int n = nums.size();
unordered_map<int, int> mp;
for(int i : nums){
mp[i]++;
}
int maxi = 0;
for(auto it : mp){
maxi = max(maxi, it.second);
}
if(maxi <= n/2){
if(n%2){
return 1;
}
else{
return 0;
}
}
return 2*maxi - n;
}
1 : Function Definition
int minLengthAfterRemovals(vector<int>& nums) {
The function definition starts here. It takes a vector of integers 'nums' and returns the minimum length after removals.
2 : Variable Initialization
int n = nums.size();
Initialize the variable 'n' to the size of the input vector 'nums'.
3 : Map Initialization
unordered_map<int, int> mp;
Initialize an unordered map 'mp' to store the frequency of each element in the vector.
4 : Loop Start
for(int i : nums){
Start a loop to iterate through each element 'i' in the vector 'nums'.
5 : Frequency Count
mp[i]++;
Increment the count for the current element 'i' in the map 'mp'.
6 : Max Frequency Initialization
int maxi = 0;
Initialize 'maxi' to store the maximum frequency found in the map.
7 : Frequency Loop
for(auto it : mp){
Start a loop to iterate through each element in the frequency map 'mp'.
8 : Update Maximum Frequency
maxi = max(maxi, it.second);
Update the 'maxi' variable with the maximum frequency found in the map.
9 : Condition Check
if(maxi <= n/2){
Check if the maximum frequency is less than or equal to half the size of the vector.
10 : Odd Size Check
if(n%2){
If the size of the vector 'n' is odd, return 1.
11 : Return 1
return 1;
Return 1 if the array size is odd and the maximum frequency is less than or equal to half the size.
12 : Even Size Check
else{
If the size of the vector 'n' is even, return 0.
13 : Return 0
return 0;
Return 0 if the array size is even and the maximum frequency is less than or equal to half the size.
14 : Return Calculation
return 2*maxi - n;
Return the result of '2*maxi - n', which is the minimum length after removals.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we iterate through the array once to count frequencies and check the conditions.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the frequency count stored in a hash map.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus