Leetcode 2845: Count of Interesting Subarrays
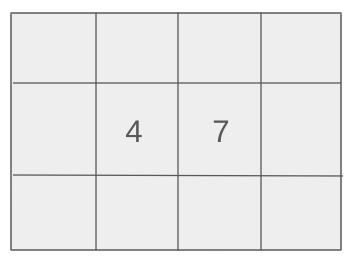
You are given an array of integers ’nums’, a positive integer ‘modulo’, and a non-negative integer ‘k’. Your task is to count the number of subarrays in ’nums’ that are interesting. A subarray ’nums[l..r]’ is interesting if the count of elements ’nums[i]’ such that ’nums[i] % modulo == k’ satisfies the condition ‘cnt % modulo == k’. Return the total count of such interesting subarrays.
Problem
Approach
Steps
Complexity
Input: An integer array 'nums', a positive integer 'modulo', and a non-negative integer 'k'.
Example: nums = [2, 4, 6], modulo = 2, k = 0
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
• 1 <= modulo <= 10^9
• 0 <= k < modulo
Output: The count of interesting subarrays in the given input.
Example: Output: 4
Constraints:
• The output should be a single integer representing the count of interesting subarrays.
Goal: Count the number of subarrays that satisfy the condition where the count of elements divisible by 'modulo' and equal to 'k' satisfies 'cnt % modulo == k'.
Steps:
• Initialize a map to store the frequency of prefix sums modulo 'modulo'.
• Iterate through the array, updating the prefix sum and checking if the current prefix sum satisfies the condition with previously encountered prefix sums.
Goal: The constraints on the input values for the problem.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^9
• 1 <= modulo <= 10^9
• 0 <= k < modulo
Assumptions:
• The input array is non-empty.
• The modulo and k values are valid as per the given constraints.
• Input: nums = [2, 4, 6], modulo = 2, k = 0
• Explanation: The subarrays that satisfy the condition are those where the count of elements divisible by 2 and equal to 0 gives a remainder of 0 when divided by 2.
Approach: The approach involves iterating through the array and using a map to track the frequency of prefix sums modulo 'modulo' to count the interesting subarrays.
Observations:
• We need to track the count of elements that satisfy 'nums[i] % modulo == k'.
• The condition 'cnt % modulo == k' can be verified by maintaining a prefix sum modulo 'modulo'.
• The problem can be solved efficiently by using a map to count the prefix sum modulo occurrences.
Steps:
• Initialize a map to keep track of the prefix sum modulo counts.
• Iterate through the array, calculate the current prefix sum modulo 'modulo', and check if the condition holds using the map.
• Count the subarrays that satisfy the condition and return the result.
Empty Inputs:
• No empty input arrays are expected as per the problem statement.
Large Inputs:
• The algorithm should handle large arrays efficiently, up to the length of 10^5.
Special Values:
• Handle cases where no subarrays satisfy the condition.
Constraints:
• The approach should work within the time and space constraints provided.
long long countInterestingSubarrays(vector<int>& nums, int mod, int k) {
unordered_map<long long,long long> mp;
long long ans = 0, prefix = 0, n = nums.size();
mp[0]++;
for(int i=0;i<n;i++) {
if(nums[i]%mod==k) prefix++;
prefix%=mod;
if(mp.find((prefix-k+mod)%mod)!=mp.end())
ans += mp[(prefix-k+mod)%mod];
mp[prefix]++;
}
return ans;
}
1 : Variable Initialization
long long countInterestingSubarrays(vector<int>& nums, int mod, int k) {
Defines the function 'countInterestingSubarrays' which takes a vector of integers, and two integers 'mod' and 'k'.
2 : Variable Declaration
unordered_map<long long,long long> mp;
Initializes an unordered map 'mp' to store frequencies of mod values encountered during the iteration.
3 : Variable Initialization
long long ans = 0, prefix = 0, n = nums.size();
Declares and initializes the variables 'ans' to store the result, 'prefix' to track the current modulo sum, and 'n' for the number of elements in the input array.
4 : Map Initialization
mp[0]++;
Increments the count of mod 0 in the map to account for an initial base case.
5 : Loop Setup
for(int i=0;i<n;i++) {
Begins a loop that iterates through each element in the input array 'nums'.
6 : Conditional Check
if(nums[i]%mod==k) prefix++;
Checks if the current element of 'nums' modulo 'mod' equals 'k', and increments the 'prefix' if true.
7 : Prefix Modulo
prefix%=mod;
Calculates the prefix modulo to ensure the prefix is within the bounds of the modulo value.
8 : Condition Check
if(mp.find((prefix-k+mod)%mod)!=mp.end())
Checks if the adjusted prefix value exists in the map, indicating a valid subarray has been found.
9 : Update Answer
ans += mp[(prefix-k+mod)%mod];
Adds the frequency of the adjusted prefix to 'ans' to accumulate the number of interesting subarrays.
10 : Update Map
mp[prefix]++;
Increments the count of the current 'prefix' value in the map.
11 : Return Statement
return ans;
Returns the total count of interesting subarrays stored in 'ans'.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) because we only iterate over the array once.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) due to the map used for storing prefix sums modulo.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus