Leetcode 2839: Check if Strings Can be Made Equal With Operations I
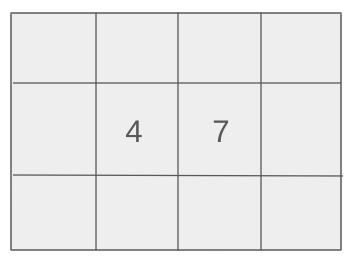
You are given two strings s1 and s2, each consisting of 4 lowercase English letters. You can perform a specific operation multiple times, where you swap two characters at indices i and j such that j - i = 2. The task is to determine if it’s possible to make s1 equal to s2 after performing any number of such operations.
Problem
Approach
Steps
Complexity
Input: The input consists of two strings, s1 and s2, each containing 4 lowercase English letters.
Example: s1 = 'abcd', s2 = 'cdab'
Constraints:
• Both s1 and s2 have a length of 4.
• Both strings consist only of lowercase English letters.
Output: Return true if it's possible to make the two strings equal using the described operations, otherwise return false.
Example: true
Constraints:
• The output should be a boolean value indicating whether the strings can be made equal or not.
Goal: The goal is to check if it's possible to transform s1 into s2 using the allowed operations.
Steps:
• Check if s1 is already equal to s2.
• If not, attempt to swap characters at indices (0, 2) and (1, 3).
• If the resulting string matches s2 after any number of swaps, return true.
• If no such transformation is possible, return false.
Goal: The constraints on the input are as follows:
Steps:
• s1 and s2 are of length 4.
• Both strings consist only of lowercase English letters.
Assumptions:
• Only the swap operation between indices (i, j) where j - i = 2 is allowed.
• Both input strings are of the same length (4).
• Input: s1 = 'abcd', s2 = 'cdab'
• Explanation: We can swap characters at indices (0, 2) and (1, 3) to transform s1 into s2.
• Input: s1 = 'abcd', s2 = 'dacb'
• Explanation: No number of swaps can make s1 equal to s2.
Approach: The problem can be solved by checking if it's possible to transform s1 into s2 by performing the allowed swap operations.
Observations:
• We are given that both strings are of length 4, so there are only a limited number of swap operations to check.
• By swapping characters at indices (0, 2) and (1, 3), we should be able to check if the strings can be made equal.
Steps:
• If the strings are already equal, return true.
• If not, swap characters at indices (0, 2) and check if the strings match.
• Next, swap characters at indices (1, 3) and check again.
• If any swap leads to the strings matching, return true; otherwise, return false.
Empty Inputs:
• Since the strings always have a length of 4, empty inputs are not possible.
Large Inputs:
• The problem has a fixed size input (length of 4), so large inputs are not a concern.
Special Values:
• The strings may consist of identical characters or all different characters.
Constraints:
• Ensure that the logic works within the fixed input size.
bool canBeEqual(string s1, string s2) {
if(s1 == s2) return true;
if(s1[0] != s2[0]) swap(s1[0],s1[2]);
if(s1 == s2) return true;
if(s1[1] != s2[1]) swap(s1[1],s1[3]);
if(s1 == s2) return true;
return false;
}
1 : Initialization
bool canBeEqual(string s1, string s2) {
Function definition to check if two strings can be made equal by swapping characters.
2 : Base Case
if(s1 == s2) return true;
Base case check if both strings are already equal.
3 : Swap Step 1
if(s1[0] != s2[0]) swap(s1[0],s1[2]);
Checks if the first characters are unequal, swaps the first and third characters of s1.
4 : Base Case
if(s1 == s2) return true;
Checks again if the strings are now equal after the first swap.
5 : Swap Step 2
if(s1[1] != s2[1]) swap(s1[1],s1[3]);
Checks if the second characters are unequal, swaps the second and fourth characters of s1.
6 : Base Case
if(s1 == s2) return true;
Checks again if the strings are now equal after the second swap.
7 : Return
return false;
Returns false if the strings are still not equal after the swaps.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is constant as the input size is fixed at 4.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant as we only need a few variables to store the strings.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus