Leetcode 2831: Find the Longest Equal Subarray
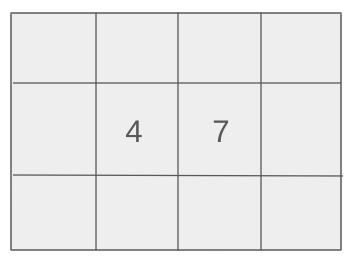
You are given a list of integers nums and an integer k. Your task is to find the length of the longest subarray where all the elements are equal after you delete at most k elements.
Problem
Approach
Steps
Complexity
Input: The input consists of an integer array nums and an integer k.
Example: nums = [1, 3, 2, 3, 1, 3], k = 3
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= nums.length
• 0 <= k <= nums.length
Output: Return the length of the longest subarray where all the elements are equal after deleting at most k elements.
Example: 3
Constraints:
• The output should be a single integer representing the length of the longest possible subarray.
Goal: To calculate the longest possible subarray of equal elements after deleting at most k elements.
Steps:
• Group the indices of each element in nums.
• For each element, use a sliding window to count how many elements can be deleted to form a subarray of equal elements.
• Calculate the maximum length of such subarrays.
Goal: The input and output sizes are constrained as follows:
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= nums.length
• 0 <= k <= nums.length
Assumptions:
• The elements of the nums array are between 1 and the length of the nums array.
• You are allowed to delete at most k elements.
• Input: nums = [1, 3, 2, 3, 1, 3], k = 3
• Explanation: After deleting two elements at indices 2 and 4, the array becomes [1, 3, 3, 3], and the longest subarray of equal elements is [3, 3, 3] with a length of 3.
• Input: nums = [1, 1, 2, 2, 1, 1], k = 2
• Explanation: After deleting two elements at indices 2 and 3, the array becomes [1, 1, 1, 1], which is the longest subarray of equal elements with a length of 4.
Approach: The problem can be solved using a sliding window approach, where we track the number of deletions needed to make a subarray of equal elements.
Observations:
• We need to find subarrays where all the elements are the same after deleting at most k elements.
• We can group the indices of each element and check which subarray can be made equal with minimal deletions.
• Sliding window technique is ideal for problems involving subarrays where we need to calculate the longest valid segment.
Steps:
• Group the indices of each element in the array.
• For each element, use a sliding window to calculate the longest subarray with at most k deletions.
Empty Inputs:
• The array may be empty, in which case the result should be 0.
Large Inputs:
• For large arrays, efficient handling of the sliding window and grouping is required.
Special Values:
• If k is 0, no deletions are allowed, and the subarray must already be equal.
Constraints:
• Ensure that the time complexity remains efficient, especially for large inputs.
int longestEqualSubarray(vector<int>& nums, int k) {
int n = nums.size();
vector<vector<int>> grid(n + 1);
int ans = 0;
for(int i = 0; i < n; i++) {
grid[nums[i]].push_back(i);
}
for(int e = 1; e <= n; e++) {
if(grid[e].size() == 0) continue;
int left = 0, right = 1, rm = 0;
while(left < grid[e].size() && right < grid[e].size()) {
rm += (grid[e][right] - grid[e][right - 1] - 1);
if (rm <= k) {
ans = max(ans, 1 + (right - left));
right++;
} else {
right++;
left++;
if(left < grid[e].size())
rm -= (grid[e][left] - grid[e][left - 1] - 1);
}
}
}
return max(ans, 1);
}
1 : Function Definition
int longestEqualSubarray(vector<int>& nums, int k) {
Define the function `longestEqualSubarray`, which takes a vector `nums` and an integer `k` as input.
2 : Variable Initialization
int n = nums.size();
Initialize `n` to the size of the `nums` array.
3 : Grid Setup
vector<vector<int>> grid(n + 1);
Create a 2D grid (vector of vectors) to store the indices of each element in `nums`.
4 : Answer Initialization
int ans = 0;
Initialize the variable `ans` to store the maximum length of the subarray found.
5 : Iterating Over nums
for(int i = 0; i < n; i++) {
Iterate over each element `i` in `nums`.
6 : Populating Grid
grid[nums[i]].push_back(i);
For each element in `nums`, push its index into the corresponding grid slot based on the element's value.
7 : Iterating Over Grid
for(int e = 1; e <= n; e++) {
Iterate through the grid from index `1` to `n`.
8 : Skipping Empty Grids
if(grid[e].size() == 0) continue;
If the grid at index `e` is empty, skip to the next iteration.
9 : Sliding Window Initialization
int left = 0, right = 1, rm = 0;
Initialize the sliding window pointers `left`, `right`, and the variable `rm` to keep track of the remaining space within the window.
10 : Sliding Window Loop
while(left < grid[e].size() && right < grid[e].size()) {
Start a `while` loop to manage the sliding window, adjusting the `left` and `right` pointers.
11 : Updating Remaining Space
rm += (grid[e][right] - grid[e][right - 1] - 1);
Update the remaining space `rm` by calculating the gaps between consecutive indices in the grid.
12 : Checking Window Validity
if (rm <= k) {
If the remaining space `rm` is within the allowed threshold `k`, update the answer.
13 : Updating Answer
ans = max(ans, 1 + (right - left));
Update the answer by taking the maximum of the current `ans` and the length of the window (right - left).
14 : Incrementing Right Pointer
right++;
Increment the `right` pointer to expand the window.
15 : Else Condition
} else {
If the `rm` exceeds `k`, reduce the window from the left.
16 : Incrementing Right Pointer Again
right++;
Increment the `right` pointer to expand the window.
17 : Incrementing Left Pointer
left++;
Increment the `left` pointer to shrink the window.
18 : Updating Remaining Space After Shrinking
if(left < grid[e].size())
rm -= (grid[e][left] - grid[e][left - 1] - 1);
After adjusting the `left` pointer, update the remaining space `rm` by recalculating the gap.
19 : Returning Result
return max(ans, 1);
Return the maximum of `ans` and `1` as the result, ensuring at least a length of 1.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear, as we iterate through the elements and use a sliding window approach.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is linear due to the storage of index groups for each element.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus