Leetcode 2829: Determine the Minimum Sum of a k-avoiding Array
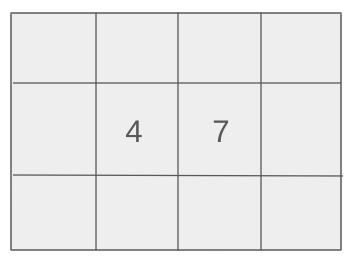
Given two integers,
n
and k
, construct a k-avoiding array of length n
. A k-avoiding array is an array of distinct positive integers where no two elements sum up to k
. Return the minimum possible sum of such an array.Problem
Approach
Steps
Complexity
Input: The input consists of two integers `n` and `k`.
Example: For example, `n = 5, k = 7`.
Constraints:
• 1 <= n, k <= 50
Output: Return the minimum possible sum of a k-avoiding array of length `n`.
Example: For `n = 5, k = 7`, the output is `24`.
Constraints:
Goal: The goal is to find a valid k-avoiding array and compute its sum.
Steps:
• Initialize an empty array to store the valid k-avoiding array.
• Iterate through integers, adding them to the array if they don't form a pair that sums to `k` with any previous element.
• Once the array reaches length `n`, return the sum of its elements.
Goal: The input consists of two integers `n` and `k`.
Steps:
• The values of `n` and `k` are between 1 and 50.
• The k-avoiding array will consist of distinct positive integers.
Assumptions:
• The integers `n` and `k` are within the constraints provided.
• The k-avoiding array will have distinct positive integers.
• Input: For `n = 5, k = 7`
• Explanation: A valid k-avoiding array is `[1, 2, 4, 5, 6]`, with a sum of `24`, where no pair of numbers sum to `7`.
Approach: The solution involves iterating through integers, constructing the k-avoiding array by ensuring no pair sums to `k`, and then returning the minimum possible sum.
Observations:
• We need to avoid pairs of numbers in the array that sum up to `k`.
• By selecting the smallest available integers and ensuring no pair sums to `k`, we can minimize the sum.
Steps:
• Start by selecting the smallest integers and add them to the array if they do not form a pair summing to `k`.
• If a number forms such a pair, skip it and move to the next available number.
• Once the array reaches the required length `n`, calculate and return its sum.
Empty Inputs:
• An empty array scenario will not be encountered due to problem constraints.
Large Inputs:
• Ensure the solution works efficiently when `n` and `k` are at their maximum values.
Special Values:
• Consider cases where `n` is small (e.g., 1 or 2), ensuring the solution handles these properly.
Constraints:
• The algorithm must work efficiently within the constraints, ensuring optimal selection of numbers.
int minimumSum(int n, int k) {
int sum = 0;
vector<int> vis(51, 0);
int i = 1;
while(n > 0 && i <= k) {
if(!vis[i] && !vis[k - i]) {
n--;
sum += i;
vis[i] = true;
}
i++;
}
while(n--) {
sum += i++;
}
return sum;
}
1 : Function Definition
int minimumSum(int n, int k) {
The function 'minimumSum' is defined, taking two integers 'n' (the number of elements) and 'k' (the upper limit for the sum).
2 : Variable Initialization
int sum = 0;
A variable 'sum' is initialized to 0, which will store the cumulative sum of selected integers.
3 : Array Initialization
vector<int> vis(51, 0);
A vector 'vis' of size 51 is initialized with all elements set to 0, to keep track of the integers that have been used.
4 : Variable Initialization
int i = 1;
The variable 'i' is initialized to 1. It will be used to iterate through integers starting from 1.
5 : Loop Start (Condition Check)
while(n > 0 && i <= k) {
A while loop starts, which will continue as long as 'n' is greater than 0 and 'i' is less than or equal to 'k'.
6 : Condition Check
if(!vis[i] && !vis[k - i]) {
An if condition checks whether both 'i' and 'k - i' are not used yet (not marked in the 'vis' vector).
7 : Decrement n
n--;
If the condition is met, 'n' is decremented, indicating that one element has been used.
8 : Update Sum
sum += i;
The current value of 'i' is added to 'sum'. This contributes to the total minimum sum.
9 : Mark as Visited
vis[i] = true;
The value of 'i' is marked as used by setting 'vis[i]' to true.
10 : Increment i
i++;
The value of 'i' is incremented to check the next integer.
11 : Second While Loop
while(n--) {
If there are still elements left (i.e., 'n' is greater than 0), a second while loop begins, incrementing 'i' and adding to the sum.
12 : Add Remaining Integers
sum += i++;
The value of 'i' is added to 'sum', and 'i' is incremented for the next iteration.
13 : Return Statement
return sum;
The function returns the total 'sum' calculated.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), as we iterate through integers and check pairs for validity.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) as we store the k-avoiding array, with the worst case requiring space proportional to `n`.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus