Leetcode 2828: Check if a String Is an Acronym of Words
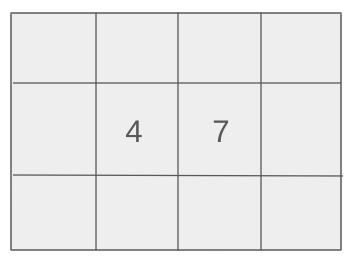
Given an array of strings
words
and a string s
, determine if s
is an acronym of words
. The string s
is considered an acronym of words
if it can be formed by concatenating the first character of each string in words
in order.Problem
Approach
Steps
Complexity
Input: The input consists of an array `words` of strings and a string `s`.
Example: For example, `words = ['orange', 'pear', 'grape']` and `s = 'opg'`
Constraints:
• 1 <= words.length <= 100
• 1 <= words[i].length <= 10
• 1 <= s.length <= 100
• words[i] and s consist of lowercase English letters.
Output: Return `true` if `s` is an acronym of `words`, otherwise return `false`.
Example: For `words = ['dog', 'cat', 'fish']` and `s = 'dcf'`, the output is `true`.
Constraints:
Goal: The goal is to check if the string `s` matches the concatenation of the first characters from each string in `words`.
Steps:
• Initialize an empty string to store the acronym.
• For each word in `words`, add its first character to the acronym string.
• Compare the acronym with `s` and return `true` if they match, otherwise return `false`.
Goal: The input will consist of a list of strings and a target acronym string.
Steps:
• The length of `words` is between 1 and 100.
• Each word in `words` has a length between 1 and 10.
• The length of `s` is between 1 and 100.
Assumptions:
• The input strings only contain lowercase English letters.
• The solution should work within the constraints for both the `words` array and string `s`.
• Input: For `words = ['orange', 'pear', 'grape']` and `s = 'opg'`
• Explanation: The first characters of the words 'orange', 'pear', and 'grape' are 'o', 'p', and 'g', respectively. Hence, `s = 'opg'` is the acronym.
Approach: The solution involves creating the acronym by concatenating the first characters of the strings in `words` and comparing it with `s`.
Observations:
• The task is simple: extract the first letter of each word and compare it with the given string `s`.
• By iterating over the list of words and constructing the acronym, we can efficiently check if `s` matches.
Steps:
• Create an empty string to store the acronym.
• Iterate through each word in `words` and append its first character to the acronym string.
• Compare the final acronym with `s`. Return `true` if they match, otherwise return `false`.
Empty Inputs:
• An empty input for `words` would not happen due to the problem constraints.
Large Inputs:
• Ensure that the algorithm can handle the maximum input size of `words`.
Special Values:
• Handle cases where `s` has fewer characters than the total number of words.
Constraints:
• The solution should run efficiently within the time and space constraints for the given problem.
bool isAcronym(vector<string>& words, string s) {
string res = "";
for(string w: words)
res += w[0];
return s == res;
}
1 : Function Definition
bool isAcronym(vector<string>& words, string s) {
The function 'isAcronym' is defined, taking a vector of strings 'words' and a string 's'. It checks whether 's' is an acronym formed by the first letter of each word in 'words'.
2 : Variable Initialization
string res = "";
A string variable 'res' is initialized as an empty string. This variable will store the concatenated first letters of each word in the 'words' vector.
3 : Loop Start
for(string w: words)
A for loop is initiated, iterating over each word 'w' in the 'words' vector. This loop will extract the first character of each word.
4 : Concatenate First Letter
res += w[0];
The first character of each word 'w' is concatenated to the 'res' string, effectively forming the acronym.
5 : Return Statement
return s == res;
The function compares the formed acronym 'res' with the input string 's'. If they are equal, it returns true; otherwise, false.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the `words` array.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n), where n is the number of words, as we store the acronym string.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus