Leetcode 2826: Sorting Three Groups
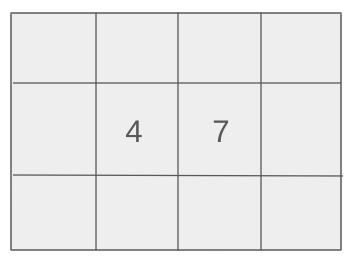
You are given an array
nums
containing elements that are either 1, 2, or 3. In each operation, you can remove an element from the array. The task is to return the minimum number of operations required to make the array non-decreasing.Problem
Approach
Steps
Complexity
Input: The input consists of an array `nums` of integers, where each element is one of the values 1, 2, or 3.
Example: For example, `nums = [3, 1, 2, 3, 1]`
Constraints:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 3
Output: Return the minimum number of operations required to make the array `nums` non-decreasing.
Example: For `nums = [2, 1, 3, 2, 3]`, the output is `2`.
Constraints:
Goal: The goal is to find the minimum number of deletions to make the array non-decreasing.
Steps:
• Track how many operations are needed to make the array non-decreasing for each possible value (1, 2, 3).
• Iterate through the array and compare each element with its previous value to determine if it should be removed.
Goal: The input will be a non-empty array of integers.
Steps:
• The length of `nums` will be between 1 and 100.
• Each element in `nums` will be 1, 2, or 3.
Assumptions:
• The array contains only 1, 2, or 3 as its elements.
• The array length is manageable (<= 100).
• Input: For `nums = [3, 1, 2, 3, 1]`
• Explanation: By removing the first, third, and fourth elements, we can make the array non-decreasing: `[1, 2, 3]`.
Approach: The solution involves calculating the minimum number of operations required to make the array non-decreasing by tracking the number of deletions for each element in `nums`.
Observations:
• The elements in `nums` are restricted to 1, 2, and 3, which simplifies the problem.
• We need to track the number of removals for each value and minimize the number of deletions.
Steps:
• Initialize three variables to track the number of deletions for each value (1, 2, and 3).
• Iterate over the array and for each element, update the corresponding variable to reflect the minimum deletions required.
Empty Inputs:
• If the array is empty (which won't happen according to constraints), no deletions are needed.
Large Inputs:
• Handle inputs with the maximum length of 100 efficiently.
Special Values:
• If all elements are already in non-decreasing order, no deletions are required.
Constraints:
• Ensure the solution works within time limits for the maximum input size.
int minimumOperations(vector<int>& A) {
int a = 0, b = 0, c = 0;
for (int x: A) {
a += x != 1;
b = min(a, b + (x != 2));
c = min(b, c + (x != 3));
}
return c;
}
1 : Function Definition
int minimumOperations(vector<int>& A) {
The function 'minimumOperations' is defined, taking a vector of integers 'A' as input and returning the minimum number of operations needed to transform 'A' into the sequence [1, 2, 3].
2 : Variable Initialization
int a = 0, b = 0, c = 0;
Variables 'a', 'b', and 'c' are initialized to zero. These variables keep track of the number of operations required to transform elements into 1, 2, and 3, respectively.
3 : Loop Start
for (int x: A) {
A for loop is started, iterating through each element 'x' of the vector 'A'. This loop is used to process each element in 'A' and update the operation counts.
4 : Update a
a += x != 1;
The variable 'a' is incremented if the current element 'x' is not equal to 1, indicating that an operation is needed to transform this element to 1.
5 : Update b
b = min(a, b + (x != 2));
The variable 'b' is updated to the minimum of the current 'a' and 'b + 1' if the current element 'x' is not equal to 2. This step tracks the operations needed to transform elements into 2.
6 : Update c
c = min(b, c + (x != 3));
The variable 'c' is updated to the minimum of the current 'b' and 'c + 1' if the current element 'x' is not equal to 3. This step tracks the operations needed to transform elements into 3.
7 : Return Statement
return c;
The function returns the value of 'c', which represents the minimum number of operations needed to transform the entire sequence into [1, 2, 3].
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the `nums` array.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1), as only a few variables are used.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus