Leetcode 2810: Faulty Keyboard
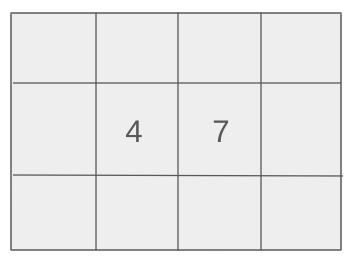
You are given a string s. On your faulty keyboard, whenever you press ‘i’, the string typed so far is reversed. Pressing any other character types it normally. Simulate typing the entire string and return the final string displayed on the screen.
Problem
Approach
Steps
Complexity
Input: A string s consisting of lowercase English letters, where the first character is not 'i'.
Example: Input: s = "hello"
Constraints:
• 1 <= s.length <= 100
• s consists of lowercase English letters
• s[0] != 'i'
Output: Return the final string that will be displayed on the screen after typing all characters in the string s.
Example: Output: "hll"
Constraints:
Goal: Simulate the process of typing each character of the string on a faulty keyboard, where typing 'i' reverses the string.
Steps:
• 1. Initialize an empty string to simulate the screen content.
• 2. For each character in the string s, add it to the string unless it is 'i'.
• 3. If the character is 'i', reverse the string typed so far.
Goal: Ensure that the solution can handle strings up to the maximum length efficiently.
Steps:
• 1 <= s.length <= 100
• s consists of lowercase English letters
• s[0] != 'i'
Assumptions:
• The string length will not exceed 100 characters.
• The input string will always begin with a character other than 'i'.
• Input: Input: s = "hello"
• Explanation: Typing the characters one by one and applying the reverse operation on encountering 'i' leads to the final string 'hll'.
• Input: Input: s = "worldi"
• Explanation: After typing 'world' and then encountering 'i', the string is reversed to 'dworl'.
Approach: We will simulate the process of typing the string and apply the reverse operation when encountering 'i'.
Observations:
• We only need to reverse the string when 'i' is encountered, which makes the problem manageable.
• We need to ensure that the string is updated after every character input, and we reverse only when needed.
Steps:
• 1. Start with an empty string representing the screen content.
• 2. For each character in the input string, either add it normally to the screen or reverse the string if 'i' is encountered.
Empty Inputs:
• There will always be at least one character in the string.
Large Inputs:
• Ensure the solution works efficiently for strings of length up to 100.
Special Values:
• If the string contains no 'i', the output will simply be the same as the input string.
Constraints:
• The solution must handle the string reversal operation efficiently.
string finalString(string s) {
string res = "";
for(auto c : s){
if(c == 'i'){
if(res.size()){
reverse(res.begin(), res.end());
}
}else
res+=c;
}
return res;
}
1 : Function Definition
string finalString(string s) {
The function 'finalString' takes a string 's' as input and returns a string as output after processing.
2 : Variable Initialization
string res = "";
Initialize an empty string 'res' to store the modified characters of the input string.
3 : Looping
for(auto c : s){
Iterate through each character 'c' in the input string 's'.
4 : Condition Check
if(c == 'i'){
Check if the current character 'c' is 'i'. If true, the string 'res' will be reversed.
5 : Condition Check
if(res.size()){
Check if the string 'res' is non-empty before attempting to reverse it.
6 : String Reversal
reverse(res.begin(), res.end());
Reverse the contents of the string 'res' using the 'reverse' function from the C++ standard library.
7 : Condition Check
}else
If the character is not 'i', continue processing the character by adding it to 'res'.
8 : String Concatenation
res+=c;
Append the current character 'c' to the string 'res'.
9 : Return Statement
return res;
Return the modified string 'res' after all characters have been processed.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: In the worst case, we reverse the string on each 'i' encounter, leading to a linear time complexity.
Best Case: O(n)
Worst Case: O(n)
Description: We store the result string which can grow up to the size of the input string.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus