Leetcode 2808: Minimum Seconds to Equalize a Circular Array
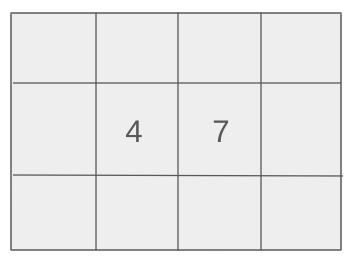
You are given a 0-indexed array nums containing n integers. At each second, replace every nums[i] with either nums[i], nums[(i-1+n)%n], or nums[(i+1)%n]. Return the minimum number of seconds required to make all elements in the array equal.
Problem
Approach
Steps
Complexity
Input: You are given a list of integers nums where nums[i] represents the value at index i.
Example: Input: nums = [3, 5, 5, 3]
Constraints:
• 1 <= n <= 10^5
• 1 <= nums[i] <= 10^9
Output: Return the minimum number of seconds needed to make all the elements in the array equal.
Example: Output: 1
Constraints:
Goal: The goal is to determine how many seconds are needed to transform the array such that all elements become the same.
Steps:
• 1. Identify the indices of each distinct number in the array.
• 2. For each distinct number, calculate the maximum gap between its occurrences.
• 3. Compute the minimum number of seconds required to fill these gaps for all distinct numbers.
Goal: The length of the array is between 1 and 100,000, and the values in the array can range from 1 to 1 billion.
Steps:
• 1 <= n <= 10^5
• 1 <= nums[i] <= 10^9
Assumptions:
• The array will always have at least one element.
• The problem assumes that all elements are integers and the array can be very large.
• Input: Input: nums = [3, 5, 5, 3]
• Explanation: In 1 second, we can replace elements so that the array becomes [5, 5, 5, 5], making all elements equal.
• Input: Input: nums = [1, 3, 2, 3, 1]
• Explanation: It takes 2 seconds to make all elements equal by first turning the array into [1, 3, 3, 3, 3] and then [3, 3, 3, 3, 3].
Approach: We will calculate how many seconds it takes to make all elements in the array equal by identifying the maximum gap for each distinct number and filling those gaps.
Observations:
• The problem requires efficiently tracking the occurrences of each number in the array.
• The key observation is to minimize the time it takes to fill the gaps between occurrences of each distinct number.
Steps:
• 1. Create a map to store the indices of each distinct number in the array.
• 2. For each distinct number, calculate the gaps between its occurrences.
• 3. Find the minimum number of seconds by computing the maximum gap and dividing it by 2.
Empty Inputs:
• The array will not be empty.
Large Inputs:
• The solution should efficiently handle arrays of size up to 100,000 elements.
Special Values:
• If all elements are the same initially, the answer should be 0.
Constraints:
• Ensure the solution runs in O(n) time to handle large inputs efficiently.
int minimumSeconds(vector<int>& nums) {
int n = nums.size();
map<int, vector<int>> pos;
for(int i = 0; i < n; i++)
pos[nums[i]].push_back(i);
int res = INT_MAX;
for(auto [key, val]: pos) {
int sec = 0;
val.push_back(val[0] + n);
for(int i = 1; i < val.size(); i++) {
sec = max(sec, (val[i] - val[i - 1])/ 2);
}
res = min(res, sec);
}
return res;
}
1 : Function Definition
int minimumSeconds(vector<int>& nums) {
The function definition that takes a vector of integers as input and returns the minimum number of seconds.
2 : Initialization
int n = nums.size();
Initializes the variable 'n' to store the size of the input vector 'nums'.
3 : Data Structure Initialization
map<int, vector<int>> pos;
Declares a map 'pos' that stores a vector of integers for each unique element in 'nums', representing the indices of each occurrence.
4 : Loop
for(int i = 0; i < n; i++)
Starts a for loop to iterate through each element of the input vector.
5 : Data Insertion
pos[nums[i]].push_back(i);
Inserts the current index 'i' into the 'pos' map for the current element 'nums[i]'.
6 : Variable Initialization
int res = INT_MAX;
Initializes 'res' to the maximum possible integer value to track the minimum time required.
7 : Loop Through Map
for(auto [key, val]: pos) {
Starts a for-each loop to iterate over the elements of the 'pos' map.
8 : Variable Initialization
int sec = 0;
Initializes a variable 'sec' to track the maximum gap between repeated elements.
9 : Circular List Adjustment
val.push_back(val[0] + n);
Adds a new element to 'val' to simulate a circular list by adding 'n' (the size of the list) to the first element.
10 : Gap Calculation
for(int i = 1; i < val.size(); i++) {
Starts a loop to calculate the gap between consecutive indices in the 'val' vector.
11 : Max Gap Calculation
sec = max(sec, (val[i] - val[i - 1])/ 2);
Updates 'sec' to the maximum gap between consecutive indices divided by 2.
12 : Update Result
res = min(res, sec);
Updates 'res' to the minimum value between 'res' and 'sec'.
13 : Return
return res;
Returns the minimum number of seconds calculated.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where n is the number of elements in the array.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to storing the indices of each distinct number in a map.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus