Leetcode 2807: Insert Greatest Common Divisors in Linked List
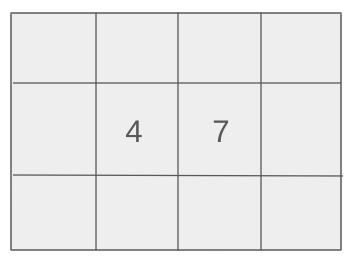
You are given the head of a linked list, where each node contains an integer. For each pair of adjacent nodes, insert a new node with a value equal to the greatest common divisor (GCD) of the two nodes. Return the modified linked list after performing this operation.
Problem
Approach
Steps
Complexity
Input: You are given a linked list where each node has an integer value. The linked list is represented as a sequence of integers.
Example: Input: head = [12, 8, 24, 36]
Constraints:
• 1 <= Node.val <= 1000
• The number of nodes in the list is between 1 and 5000.
Output: Return the linked list after inserting new nodes with the GCD between adjacent nodes.
Example: Output: [12, 4, 8, 4, 24, 12, 36]
Constraints:
Goal: The goal is to iterate through the linked list, compute the GCD of every pair of adjacent nodes, and insert a new node with the GCD value in between them.
Steps:
• 1. Iterate through the linked list.
• 2. For each pair of adjacent nodes, compute the GCD of their values.
• 3. Create a new node with the GCD value and insert it between the two adjacent nodes.
• 4. Continue this process until the end of the list.
Goal: The list will have between 1 and 5000 nodes, and each node will contain a value between 1 and 1000.
Steps:
• The number of nodes in the list is between 1 and 5000.
• 1 <= Node.val <= 1000
Assumptions:
• The linked list contains at least one node.
• Adjacent nodes will always be present unless the list has only one node.
• Input: Input: head = [12, 8, 24, 36]
• Explanation: Between 12 and 8, the GCD is 4. Between 8 and 24, the GCD is 8. Between 24 and 36, the GCD is 12. So, the final linked list is [12, 4, 8, 4, 24, 12, 36].
• Input: Input: head = [5]
• Explanation: Since there is only one node in the list, no insertions are made, and the original list remains unchanged.
Approach: We will iterate through the list, compute the GCD for each pair of adjacent nodes, and insert the new node with the GCD value between them.
Observations:
• The problem is straightforward, involving GCD calculations and linked list manipulation.
• The main challenge lies in correctly inserting the new nodes between existing nodes while maintaining the integrity of the linked list.
Steps:
• 1. Start from the head of the list.
• 2. For each adjacent pair of nodes, calculate their GCD.
• 3. Create a new node with the computed GCD and insert it between the nodes.
• 4. Move to the next pair and repeat until the end of the list.
Empty Inputs:
• There are no empty inputs, as the list will always have at least one node.
Large Inputs:
• The function needs to handle up to 5000 nodes, but the logic remains efficient with a linear time complexity.
Special Values:
• If the list contains only one node, no new nodes will be inserted.
Constraints:
• Ensure that all GCD calculations are performed correctly and efficiently for each pair of nodes.
ListNode* insertGreatestCommonDivisors(ListNode* head) {
ListNode* res = head;
while(head && head->next) {
ListNode* node = new ListNode(__gcd(head->val, head->next->val));
ListNode* tmp = head->next;
head->next = node;
node->next = tmp;
head = tmp;
}
return res;
}
1 : Function Definition
ListNode* insertGreatestCommonDivisors(ListNode* head) {
Function definition: Starts the function to insert GCDs into a linked list.
2 : Initialization
ListNode* res = head;
Initializes a pointer 'res' to keep track of the head of the linked list.
3 : Loop
while(head && head->next) {
Starts a while loop that continues as long as there are at least two nodes.
4 : GCD Calculation
ListNode* node = new ListNode(__gcd(head->val, head->next->val));
Creates a new node containing the GCD of the current node and the next node.
5 : Node Manipulation
ListNode* tmp = head->next;
Stores a temporary reference to the next node in the list.
6 : Node Manipulation
head->next = node;
Updates the current node to point to the new GCD node.
7 : Node Manipulation
node->next = tmp;
Sets the next pointer of the GCD node to the original next node.
8 : Pointer Update
head = tmp;
Moves the 'head' pointer to the next original node for the next iteration.
9 : Return
return res;
Returns the head of the modified linked list with the inserted GCDs.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) where n is the number of nodes, as we iterate through the list once and perform constant-time GCD calculations for each adjacent pair.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) due to the extra nodes being inserted into the list.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus