Leetcode 2806: Account Balance After Rounded Purchase
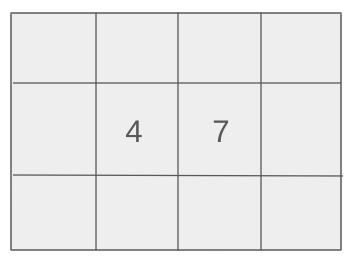
You have a bank account with a balance of 100 dollars. You are given a purchase amount and need to round it to the nearest multiple of 10. Then, deduct the rounded amount from your account balance and return the remaining balance.
Problem
Approach
Steps
Complexity
Input: You are given an integer 'purchaseAmount' representing the price of an item you wish to buy.
Example: Input: purchaseAmount = 18
Constraints:
• 0 <= purchaseAmount <= 100
Output: Return the bank account balance after the rounded amount has been deducted.
Example: Output: 80
Constraints:
Goal: Calculate the nearest multiple of 10 for the given purchase amount and update the bank balance.
Steps:
• 1. Round the 'purchaseAmount' to the nearest multiple of 10.
• 2. Subtract the rounded amount from the initial balance of 100 dollars.
• 3. Return the updated balance.
Goal: The purchase amount will be a non-negative integer less than or equal to 100.
Steps:
• 0 <= purchaseAmount <= 100
Assumptions:
• The purchase amount is always a non-negative integer and will be rounded to the nearest multiple of 10.
• Input: Input: purchaseAmount = 18
• Explanation: The nearest multiple of 10 to 18 is 20. So the account balance becomes 100 - 20 = 80.
• Input: Input: purchaseAmount = 8
• Explanation: The nearest multiple of 10 to 8 is 10. So the account balance becomes 100 - 10 = 90.
Approach: The problem can be solved by determining the nearest multiple of 10 and then subtracting it from the initial balance.
Observations:
• The rounding rules for the purchase amount must be carefully followed to ensure the correct amount is deducted.
• We need to handle both small values (e.g., 1) and large values (e.g., 100) correctly, ensuring rounding happens in all cases.
Steps:
• Step 1: Round the 'purchaseAmount' to the nearest multiple of 10 using conditional checks.
• Step 2: Subtract the rounded value from the initial account balance of 100.
• Step 3: Return the final balance after the purchase.
Empty Inputs:
• The input will always be a valid integer within the given range, so empty inputs are not a concern.
Large Inputs:
• The largest possible input (purchaseAmount = 100) will be handled by rounding it to 100.
Special Values:
• When the purchaseAmount is 5, 15, 25, etc., it will be rounded up to the next multiple of 10.
Constraints:
• The input will always be within the specified range and will not exceed the maximum value of 100.
int accountBalanceAfterPurchase(int val) {
if(val % 10 == 5) val += 5;
else if(val % 10 > 5) {
val = val / 10;
val = (val + 1) * 10;
} else {
val = (val / 10) * 10;
}
return 100 - val;
}
1 : function
int accountBalanceAfterPurchase(int val) {
Start of the function definition to calculate the account balance after a purchase based on a given value.
2 : if_condition
if(val % 10 == 5) val += 5;
Check if the last digit of the value is 5, and if so, increment the value by 5.
3 : else_if_condition
else if(val % 10 > 5) {
If the last digit of the value is greater than 5, then adjust the value by dividing by 10 and rounding up.
4 : operation
val = val / 10;
Divide the value by 10 to remove the last digit.
5 : operation
val = (val + 1) * 10;
Increase the value by 1 and multiply by 10 to round it to the next multiple of 10.
6 : else_condition
} else {
If the last digit is less than 5, proceed with rounding down.
7 : operation
val = (val / 10) * 10;
Divide the value by 10 to remove the last digit and multiply by 10 to round it down.
8 : return_statement
return 100 - val;
Return the final value after subtracting from 100 to calculate the account balance after the purchase.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is O(1) because the operations involved (rounding and subtraction) are constant time operations.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) because only a constant amount of space is used for calculations.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus