Leetcode 2789: Largest Element in an Array after Merge Operations
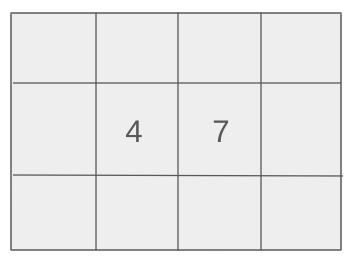
You are given a 0-indexed array ’nums’ consisting of positive integers. You can repeatedly perform an operation where you choose an index ‘i’ such that ’nums[i] <= nums[i + 1]’, replace ’nums[i + 1]’ with ’nums[i] + nums[i + 1]’, and remove ’nums[i]’. Your task is to determine the largest possible value that can remain in the array after performing any number of operations.
Problem
Approach
Steps
Complexity
Input: You are given a 0-indexed array of positive integers, 'nums'.
Example: Input: nums = [2, 5, 7, 9, 3]
Constraints:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^6
Output: Return the largest possible value that can remain in the array after performing the operations.
Example: Output: 21
Constraints:
Goal: Find the largest element that can be obtained after performing the operations.
Steps:
• Iterate through the array from the last element to the first.
• For each element, check if it's greater than or equal to the previous element.
• If so, add the current element to the previous element, remove the current element, and continue.
Goal: Ensure that the problem adheres to the given constraints.
Steps:
• 1 <= nums.length <= 10^5
• 1 <= nums[i] <= 10^6
Assumptions:
• All elements in the input array are positive integers.
• The input array may contain a variety of sizes, ranging from 1 to 10^5 elements.
• Input: Input: nums = [2, 5, 7, 9, 3]
• Explanation: Starting with [2, 5, 7, 9, 3], we perform the operations: [7, 7, 9, 3] -> [7, 16, 3] -> [23, 3]. The largest possible element is 21.
• Input: Input: nums = [4, 1, 3]
• Explanation: After performing the operations [5, 3] -> [8], the largest value is 8.
Approach: To maximize the largest element in the array, we will combine adjacent elements based on the condition that the current element is less than or equal to the next.
Observations:
• The process can be viewed as accumulating values into the largest possible element while traversing from the end of the array.
• I need to efficiently combine values when possible and keep track of the largest value in the array.
Steps:
• Initialize a variable to store the largest value (starting with the last element).
• Traverse the array from right to left.
• If the current element is greater than or equal to the previous element, add it to the previous one and remove it from the array.
• Return the largest value after processing the array.
Empty Inputs:
• The input array is guaranteed to have at least one element, so no need to handle empty inputs.
Large Inputs:
• Ensure the solution is optimized for arrays with a length of up to 10^5.
Special Values:
• If all elements are already in decreasing order, no operation will be possible, and the largest value is the last element.
Constraints:
• Handle arrays with up to 10^5 elements efficiently.
long long maxArrayValue(vector<int>& in) {
int n = in.size();
vector<long long> nums(n, 0);
for(int i = 0; i < n; i++)
nums[i] = in[i];
long long res = nums[n - 1];
for(int i = n - 1; i >= 0; i--) {
res = max(res, (long long)nums[i]);
if(i > 0 && nums[i] >= nums[i - 1]) {
nums[i - 1] += nums[i];
}
}
return res;
}
1 : Function Declaration
long long maxArrayValue(vector<int>& in) {
The function `maxArrayValue` is declared, accepting a reference to a vector of integers `in` and returning a long long value, which is the maximum possible value after applying transformations.
2 : Empty Line
An empty line to separate sections of code for better readability.
3 : Variable Initialization
int n = in.size();
The variable `n` is initialized to store the size of the input vector `in`, which is used to iterate over the array.
4 : Vector Initialization
vector<long long> nums(n, 0);
A vector `nums` of type long long is initialized with `n` elements, all set to 0. This vector will store the transformed values of the input array.
5 : Loop Initialization
for(int i = 0; i < n; i++)
A for loop is initiated to iterate through the entire input vector `in`. Each element will be copied to the corresponding index in `nums`.
6 : Value Assignment
nums[i] = in[i];
Inside the loop, each element of the input array `in` is copied into the `nums` array.
7 : Variable Initialization
long long res = nums[n - 1];
The variable `res` is initialized with the last element of the `nums` array. This will track the maximum value during the iteration.
8 : Loop Initialization
for(int i = n - 1; i >= 0; i--) {
A for loop is initiated to iterate through the `nums` array in reverse order, from the last element to the first.
9 : Maximum Update
res = max(res, (long long)nums[i]);
The `res` variable is updated to the maximum of its current value and the current element in `nums`. This ensures `res` holds the largest value encountered.
10 : Condition Check
if(i > 0 && nums[i] >= nums[i - 1]) {
An if statement checks if the current element `nums[i]` is greater than or equal to the previous element `nums[i - 1]` and if the index is greater than 0.
11 : Value Update
nums[i - 1] += nums[i];
If the condition is true, the previous element `nums[i - 1]` is incremented by the current element `nums[i]`. This transformation ensures that larger values accumulate towards the start of the array.
12 : Return Result
return res;
The function returns the value of `res`, which now holds the maximum possible value obtained after processing all elements of the input array.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is linear, O(n), as we traverse the array once.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) in the worst case when storing a copy of the array, but it can be optimized to O(1) if done in-place.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus