Leetcode 2778: Sum of Squares of Special Elements
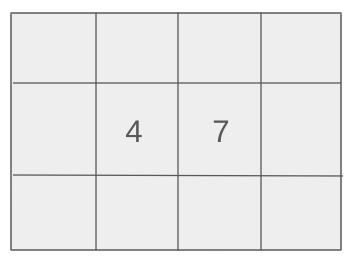
Given a 1-indexed integer array nums of length n, an element nums[i] is considered special if the index i divides the length n (i.e., n % i == 0). Your task is to find the sum of the squares of all special elements in the array.
Problem
Approach
Steps
Complexity
Input: A 1-indexed integer array nums of length n.
Example: nums = [5, 3, 2, 7]
Constraints:
• 1 <= nums.length == n <= 50
• 1 <= nums[i] <= 50
Output: Return the sum of the squares of all special elements in nums.
Example: Output: 58
Constraints:
• Output is an integer.
Goal: Identify all special elements in the array and calculate the sum of their squares.
Steps:
• Iterate through all elements of nums with their respective 1-based indices.
• Check if the index i divides the length n.
• If i divides n, calculate the square of nums[i] and add it to the result.
• Return the sum after processing all indices.
Goal: Limits and conditions for the input and output.
Steps:
• Array length n is between 1 and 50 inclusive.
• Each element in nums is between 1 and 50 inclusive.
Assumptions:
• The input array nums is always 1-indexed.
• The length of nums is a positive integer.
• Input: nums = [4, 5, 6]
• Explanation: n = 3. Special elements are nums[1] (4) and nums[3] (6). Sum of squares = 4^2 + 6^2 = 16 + 36 = 52.
• Input: nums = [1, 3, 7, 2]
• Explanation: n = 4. Special elements are nums[1] (1), nums[2] (3), and nums[4] (2). Sum of squares = 1^2 + 3^2 + 2^2 = 1 + 9 + 4 = 14.
Approach: Iterate through the array using a loop and check divisibility conditions for each 1-based index. Accumulate the sum of the squares for all qualifying elements.
Observations:
• The index i should divide the length n of the array.
• 1-based indexing needs careful handling to align with array operations.
• A direct loop from 1 to n with modulus checks will suffice for this problem due to small constraints.
Steps:
• Initialize a variable to store the sum of squares.
• Loop through the array using 1-based indexing.
• Check if n % i == 0 for the current index i.
• If true, add the square of nums[i-1] to the sum.
• Return the accumulated sum after the loop completes.
Empty Inputs:
• Not applicable as per constraints (n >= 1).
Large Inputs:
• Test cases with nums having maximum length and maximum values.
Special Values:
• Test cases where all elements in nums are the same.
• Test cases where nums contains minimal values (all 1s).
Constraints:
• Ensure the code properly handles 1-based indexing.
int sumOfSquares(vector<int>& nums) {
int ans = 0;
int n = nums.size();
for(int i = 0; i < n; i++)
if(n % (i + 1) == 0) ans += nums[i] * nums[i];
return ans;
}
1 : Function Declaration
int sumOfSquares(vector<int>& nums) {
This line declares the function `sumOfSquares`, which accepts a reference to an integer vector `nums` and returns an integer result.
2 : Variable Initialization
int ans = 0;
The variable `ans` is initialized to 0 and will store the sum of the squares of the appropriate elements in the `nums` array.
3 : Variable Initialization
int n = nums.size();
The variable `n` is initialized to store the size of the array `nums`, which will be used to find divisors of the array's size.
4 : Looping
for(int i = 0; i < n; i++)
A for-loop starts that iterates from 0 to `n-1`, covering all the indices of the `nums` array.
5 : Conditional Function Call
if(n % (i + 1) == 0) ans += nums[i] * nums[i];
Inside the loop, a condition checks if the index `i + 1` is a divisor of `n` (i.e., if `n % (i + 1) == 0`). If true, the square of `nums[i]` is added to `ans`.
6 : Return Statement
return ans;
After completing the loop, the function returns the value of `ans`, which contains the sum of the squares of the elements whose indices are divisors of `n`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution involves a single pass through the array, checking divisibility and accumulating results.
Best Case: O(1)
Worst Case: O(1)
Description: The solution uses constant additional space for computation.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus