Leetcode 2772: Apply Operations to Make All Array Elements Equal to Zero
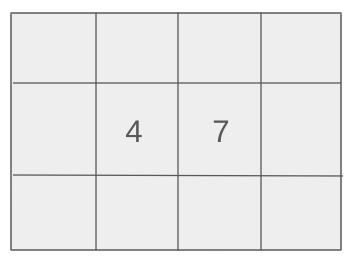
You are given a 0-indexed integer array nums and a positive integer k. You can repeatedly select a contiguous subarray of size k and decrease all its elements by 1. Determine if it is possible to make all elements in nums equal to 0 using this operation.
Problem
Approach
Steps
Complexity
Input: A 0-indexed integer array nums and an integer k.
Example: nums = [3,3,2,1,0], k = 2
Constraints:
• 1 <= k <= nums.length <= 100,000
• 0 <= nums[i] <= 1,000,000
Output: Return a boolean value: true if all elements can be made 0, and false otherwise.
Example: Output: true
Constraints:
• Output is either true or false.
Goal: Check if all elements in the array can be reduced to 0 using the specified operation.
Steps:
• Iterate through the array while keeping track of the cumulative decrease required to meet the operation conditions.
• At each index, ensure the required decrease does not exceed the current element.
• Adjust the cumulative decrease using a sliding window technique to manage subarrays of size k.
• Return true if all elements can be made 0; otherwise, return false.
Goal: Limits and conditions for input and output validity.
Steps:
• The array nums has at least 1 element and at most 100,000 elements.
• k is a positive integer no greater than the length of nums.
• Elements of nums are non-negative integers.
Assumptions:
• The operation can be performed any number of times.
• The array elements are non-negative.
• Input: nums = [3,2,1,0], k = 2
• Explanation: Select the subarray [3,2] to reduce it by 1. Repeat until all elements are 0. The output is true.
• Input: nums = [1,3,1,1], k = 3
• Explanation: It is not possible to reduce all elements to 0. The output is false.
Approach: Use a greedy algorithm combined with a sliding window to manage the operations efficiently.
Observations:
• At each step, only subarrays of size k can be reduced.
• The operation is cumulative and impacts the next elements.
• Keep track of the cumulative decrease to efficiently determine if the operation is valid for each index.
Steps:
• Initialize a variable to track the cumulative decrease.
• Iterate through the array, applying the cumulative decrease to each element.
• If the cumulative decrease exceeds the current element, return false.
• Use a sliding window approach to remove the cumulative impact of elements outside the current subarray of size k.
• After processing all elements, verify if the cumulative decrease has reached 0.
Empty Inputs:
• An empty array is invalid as per constraints.
Large Inputs:
• Test arrays with the maximum size (100,000) and values to ensure efficiency.
Special Values:
• Test arrays with all elements as 0, which should immediately return true.
Constraints:
• Ensure k is less than or equal to the array size.
bool checkArray(vector<int>& A, int k) {
int cur = 0, n = A.size();
for (int i = 0; i < n; ++i) {
if (cur > A[i])
return false;
A[i] -= cur;
cur += A[i];
if (i >= k - 1)
cur -= A[i - k + 1];
}
return cur == 0;
}
1 : Variable Initialization
bool checkArray(vector<int>& A, int k) {
This line declares the function `checkArray` that accepts a reference to an integer vector `A` and an integer `k`, which represents the size of the subarrays we are considering.
2 : Variable Initialization
int cur = 0, n = A.size();
The variable `cur` is initialized to 0, which will be used to keep track of the sum of the elements in the sliding window. `n` stores the size of the array `A`.
3 : Looping
for (int i = 0; i < n; ++i) {
A loop is initiated to iterate through the elements of the array `A` from index `0` to `n-1`.
4 : Conditional Function Call
if (cur > A[i])
This condition checks if the current value `cur` exceeds the current element `A[i]`. If true, it means the transformation is not possible, so the function returns `false`.
5 : Return Statement
return false;
If the condition `cur > A[i]` is met, the function immediately returns `false`, indicating that the desired transformation is not possible.
6 : Variable Manipulation
A[i] -= cur;
The current element `A[i]` is adjusted by subtracting the value of `cur`, essentially modifying the array during the iteration.
7 : Variable Manipulation
cur += A[i];
The modified value of `A[i]` is added to `cur`, updating the sum of the current window.
8 : Conditional Function Call
if (i >= k - 1)
This condition checks if the index `i` has reached or exceeded `k - 1`, which marks the point where we begin reducing the window size.
9 : Variable Manipulation
cur -= A[i - k + 1];
When the condition `i >= k - 1` is true, the value at `A[i - k + 1]` is subtracted from `cur` to shrink the sliding window by removing the effect of the element that has moved out of the window.
10 : Return Statement
return cur == 0;
After completing the loop, the function returns `true` if `cur` is equal to 0, indicating that the transformation is possible, otherwise `false`.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution involves a single pass through the array, with constant-time operations per index.
Best Case: O(1)
Worst Case: O(1)
Description: The solution uses a constant amount of extra space.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus