Leetcode 2765: Longest Alternating Subarray
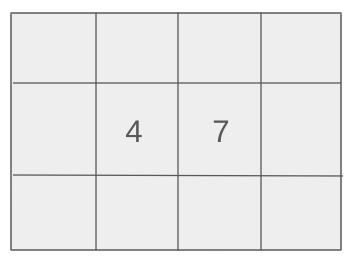
You are given a 0-indexed integer array ’nums’. A subarray ’s’ is called alternating if it follows a specific pattern of alternating differences between consecutive elements: +1, -1, +1, -1, and so on. Return the maximum length of all alternating subarrays, or -1 if no such subarray exists.
Problem
Approach
Steps
Complexity
Input: The input consists of a single integer array 'nums'.
Example: nums = [1, 2, 3, 2, 3]
Constraints:
• 2 <= nums.length <= 100
• 1 <= nums[i] <= 10^4
Output: Return the maximum length of all alternating subarrays, or -1 if no alternating subarray exists.
Example: For nums = [1, 2, 3, 2, 3], the output is 4.
Constraints:
• The output should be a single integer representing the maximum length of alternating subarrays.
Goal: Find the longest alternating subarray by iterating over the array and checking the alternating pattern condition.
Steps:
• Initialize variables to store the maximum length and the current subarray's length.
• Iterate through the array to find subarrays that follow the alternating pattern.
• If an alternating subarray is found, update the maximum length.
Goal: The array contains at least two elements, and each element is within the range 1 to 10^4.
Steps:
• 2 <= nums.length <= 100
• 1 <= nums[i] <= 10^4
Assumptions:
• The input array contains at least two elements.
• Input: For nums = [1, 2, 3, 2, 3]
• Explanation: The alternating subarrays are [1, 2], [2, 3], [3, 2, 3], and [2, 3, 2, 3]. The longest one is [2, 3, 2, 3], with length 4.
• Input: For nums = [5, 6, 7]
• Explanation: The alternating subarrays are [5, 6] and [6, 7], both of length 2.
Approach: We use a two-pointer technique to check for alternating subarrays and track the longest one.
Observations:
• The array length is relatively small (<= 100), so a brute force approach is feasible.
• By iterating through the array and checking the alternating pattern, we can efficiently find the longest alternating subarray.
Steps:
• Initialize variables for the maximum subarray length and a temporary length counter.
• Loop through the array, checking the alternating condition between each pair of elements.
• If an alternating pattern is maintained, increment the length counter.
• If the pattern is broken, reset the counter and update the maximum length if necessary.
• Return the maximum length found, or -1 if no alternating subarray exists.
Empty Inputs:
• The input will always have at least two elements, so no need to handle empty arrays.
Large Inputs:
• Handle arrays with 100 elements, ensuring the solution runs efficiently within the time limit.
Special Values:
• If no alternating subarray is found, return -1.
Constraints:
• Ensure that the solution works within the time constraints for arrays of size up to 100.
int alternatingSubarray(vector<int>& A) {
int n = A.size(), res = 0, j = 0;
for (int i = 0; i < n; ++i)
for (j = i + 1; j < n && A[j] == A[i] + (j - i) % 2; ++j)
res = max(res, j - i + 1);
return res > 1 ? res : -1;
}
1 : Function Definition
int alternatingSubarray(vector<int>& A) {
Defines the function `alternatingSubarray`, which takes a vector `A` and returns the length of the longest alternating subarray.
2 : Variable Initialization
int n = A.size(), res = 0, j = 0;
Initializes variables: `n` as the size of the vector `A`, `res` to store the result (longest alternating subarray length), and `j` as a helper index.
3 : Outer Loop
for (int i = 0; i < n; ++i)
Starts an outer loop with `i` to iterate through each possible starting index of the subarray.
4 : Inner Loop (Check Alternating Pattern)
for (j = i + 1; j < n && A[j] == A[i] + (j - i) % 2; ++j)
Starts an inner loop with `j`, checking if the current element alternates with the previous one (difference between elements alternates between 1 and -1).
5 : Update Result
res = max(res, j - i + 1);
Updates `res` to store the maximum length of the alternating subarray found so far.
6 : Return Result
return res > 1 ? res : -1;
Returns the length of the longest alternating subarray, or -1 if no such subarray with length greater than 1 exists.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n), where n is the length of the array. We loop through the array once.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is O(1) as we use only a few variables to track the lengths.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus