Leetcode 2760: Longest Even Odd Subarray With Threshold
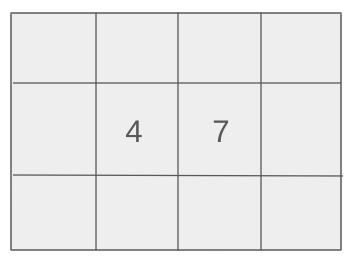
You are given a 0-indexed integer array ’nums’ and an integer ’threshold’. Find the length of the longest subarray of ’nums’ that satisfies the following conditions: (1) ’nums[l]’ is even, (2) the elements alternate between even and odd, and (3) all elements in the subarray are <= threshold.
Problem
Approach
Steps
Complexity
Input: You will be given an array of integers, 'nums', and a threshold integer.
Example: nums = [1, 4, 3, 2, 7], threshold = 5
Constraints:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
• 1 <= threshold <= 100
Output: Return the length of the longest subarray satisfying the conditions.
Example: For nums = [1, 4, 3, 2, 7] and threshold = 5, the output is 4.
Constraints:
• Return the length of the longest valid subarray.
Goal: Find the longest subarray that satisfies all three conditions: starting with an even number, alternating between even and odd, and all elements <= threshold.
Steps:
• Iterate through the array and check the conditions for each potential subarray.
• Track the length of valid subarrays and update the result when a longer valid subarray is found.
Goal: The array length is between 1 and 100. All array elements are between 1 and 100, and the threshold is also between 1 and 100.
Steps:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
• 1 <= threshold <= 100
Assumptions:
• The input array will have at least one element.
• Input: For nums = [1, 4, 3, 2, 7] and threshold = 5
• Explanation: We are looking for the longest subarray that starts with an even number, alternates between even and odd numbers, and has all numbers <= 5. The valid subarray is [4, 3, 2, 7], which satisfies all conditions, and its length is 4.
Approach: The solution involves iterating over the array, checking for subarrays that meet the required conditions, and keeping track of the longest valid subarray.
Observations:
• The subarray must start with an even number and alternate between even and odd numbers.
• We need to check if all numbers in the subarray are <= threshold.
• To find the longest valid subarray, we can iterate through the array while checking if the current subarray meets the conditions.
Steps:
• Initialize variables to track the longest valid subarray.
• Iterate through the array and check if the conditions are met for each subarray.
• For each valid subarray, update the result if it is longer than the previous longest subarray.
Empty Inputs:
• The input array will always have at least one element.
Large Inputs:
• The array is small enough (up to 100 elements) that the solution can handle it in a reasonable amount of time.
Special Values:
• All elements in the array may be odd or even, and the threshold may be larger than the maximum element in the array.
Constraints:
• Ensure that the subarrays are contiguous and that all elements satisfy the threshold condition.
int longestAlternatingSubarray(vector<int>& n, int threshold) {
int res = 0;
for (int l = 0, cnt = 0; l < n.size(); ++l) {
if (n[l] <= threshold)
cnt = cnt == 0 ?
n[l] % 2 == 0 :
n[l] % 2 == n[l - 1] % 2 ? n[l] % 2 == 0 : cnt + 1;
else
cnt = 0;
res = max(res, cnt);
}
return res;
}
1 : Function Definition
int longestAlternatingSubarray(vector<int>& n, int threshold) {
Defines the function `longestAlternatingSubarray`, which takes a vector of integers `n` and an integer `threshold` as input and returns the length of the longest alternating subarray.
2 : Variable Initialization
int res = 0;
Initializes the variable `res` to 0, which will store the length of the longest alternating subarray found during the loop.
3 : For Loop Setup
for (int l = 0, cnt = 0; l < n.size(); ++l) {
Starts a for loop with index `l` to iterate through the array `n`. It also initializes a counter `cnt` to track the length of the current alternating subarray.
4 : Threshold Check
if (n[l] <= threshold)
Checks if the current element `n[l]` is less than or equal to the specified `threshold`. If it is, the element can be considered for the alternating subarray.
5 : First Alternating Element
cnt = cnt == 0 ?
If `cnt` is 0 (i.e., the first element of a potential alternating subarray), it checks whether the current element is even. If it is even, it sets `cnt` to 1 to start the subarray.
6 : Alternating Check
n[l] % 2 == 0 :
Checks if the current element is even (i.e., `n[l] % 2 == 0`). If so, `cnt` is initialized to 1.
7 : Alternating Subarray Continuation
n[l] % 2 == n[l - 1] % 2 ? n[l] % 2 == 0 : cnt + 1;
If the current element is part of an alternating subarray, it checks if the element has the same parity (odd/even) as the previous element. If it alternates correctly, it increments `cnt`; otherwise, it resets `cnt` to start a new alternating subarray.
8 : Element Greater Than Threshold
else
If the current element exceeds the threshold, it resets the counter `cnt` because the element cannot be part of the alternating subarray.
9 : Reset Counter After Invalid Element
cnt = 0;
Resets the counter `cnt` to 0 if the current element is greater than the threshold, indicating the end of a potential alternating subarray.
10 : Update Result
res = max(res, cnt);
Updates the result `res` to the maximum value between the current longest alternating subarray length (`res`) and the current subarray length (`cnt`).
11 : Return Result
return res;
Returns the result `res`, which contains the length of the longest alternating subarray found in the array `n`.
Best Case: O(n), where n is the length of the input array.
Average Case: O(n).
Worst Case: O(n).
Description: We iterate through the array once, checking the conditions for each element, which results in linear time complexity.
Best Case: O(1).
Worst Case: O(1).
Description: We only need a few variables to store the current subarray length and the result, so the space complexity is constant.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus