Leetcode 2739: Total Distance Traveled
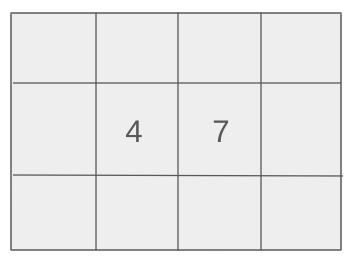
A truck has two fuel tanks, one main tank and one additional tank. The truck’s mileage is 10 km per liter, and fuel from the additional tank can be injected into the main tank after every 5 liters of fuel consumed. Your task is to calculate the maximum distance the truck can travel, considering both the main tank and the additional tank.
Problem
Approach
Steps
Complexity
Input: The input consists of two integers: `mainTank` and `additionalTank`, representing the fuel available in the main tank and the additional tank in liters.
Example: mainTank = 8, additionalTank = 12
Constraints:
• 1 <= mainTank <= 100
• 1 <= additionalTank <= 100
Output: Return the maximum distance the truck can travel.
Example: Output: 80
Constraints:
Goal: Calculate the total distance the truck can travel by consuming fuel from the main tank and transferring fuel from the additional tank when necessary.
Steps:
• While there is fuel in the main tank, calculate the distance that can be traveled with the current fuel.
• For every 5 liters consumed, transfer 1 liter from the additional tank to the main tank if possible.
• Repeat until the main tank is empty.
Goal: The problem guarantees that both `mainTank` and `additionalTank` will contain at least 1 liter of fuel and no more than 100 liters.
Steps:
• 1 <= mainTank <= 100
• 1 <= additionalTank <= 100
Assumptions:
• Fuel injection only happens after 5 liters are consumed from the main tank.
• The truck’s mileage is always 10 km per liter of fuel.
• Input: mainTank = 8, additionalTank = 12
• Explanation: Initially, the truck starts with 8 liters in the main tank, allowing it to travel 80 km. After consuming 5 liters, the additional tank injects 1 liter into the main tank. This continues until the main tank is empty.
• Input: mainTank = 3, additionalTank = 6
• Explanation: The truck starts with 3 liters in the main tank, which allows it to travel 30 km. No fuel is injected from the additional tank because fuel transfer only happens after consuming 5 liters.
Approach: The approach is to simulate the process of consuming fuel from the main tank and transferring fuel from the additional tank at the appropriate intervals.
Observations:
• The additional tank only injects fuel after every 5 liters consumed from the main tank.
• The total distance is calculated by considering the fuel available in the main tank and the injections from the additional tank.
• The process is a repetitive calculation until the main tank is empty.
Steps:
• Initialize a variable to track the total distance.
• While there is fuel in the main tank, consume 5 liters or less and add to the distance.
• If 5 liters are consumed and the additional tank has fuel, transfer 1 liter to the main tank.
• Repeat the process until the main tank is empty.
Empty Inputs:
• Both tanks are empty at the start (not valid in this problem).
Large Inputs:
• Maximum values for both tanks (100 liters each).
Special Values:
• If the main tank has less than 5 liters initially, no injection occurs.
Constraints:
• Ensure fuel injection happens only after 5 liters are consumed.
int distanceTraveled(int mt, int at) {
int net = 0;
while(mt > 0) {
int red = min(5, mt);
mt -= red;
net += red * 10;
if(red == 5 && at > 0) {
mt += 1;
at--;
}
}
return net;
}
1 : Function Definition
int distanceTraveled(int mt, int at) {
The function `distanceTraveled` is defined with parameters `mt` (the remaining distance to travel) and `at` (the available time buffer). It returns an integer representing the total distance traveled.
2 : Variable Initialization
int net = 0;
The variable `net` is initialized to zero to keep track of the total distance traveled.
3 : Loop - Condition
while(mt > 0) {
A `while` loop starts, which will continue as long as there is remaining distance (`mt > 0`).
4 : Variable Calculation
int red = min(5, mt);
The variable `red` is assigned the minimum value between 5 and the remaining distance `mt`, representing the distance that can be covered in the current iteration.
5 : Variable Update
mt -= red;
The remaining distance `mt` is decreased by the value of `red`.
6 : Distance Calculation
net += red * 10;
The total distance traveled (`net`) is updated by adding the distance covered in this iteration, multiplied by 10 (penalty factor).
7 : Conditional Check
if(red == 5 && at > 0) {
A condition checks if exactly 5 units were covered (`red == 5`) and if there is any available time buffer (`at > 0`).
8 : Variable Update
mt += 1;
If the condition is true, 1 unit is added back to `mt` (this represents a penalty for the time buffer).
9 : Variable Update
at--;
The available time buffer `at` is decremented by 1.
10 : Return Statement
return net;
The function returns the total distance traveled (`net`), which has been accumulated during the `while` loop.
Best Case: O(1)
Average Case: O(n) where n is the amount of fuel in the main tank.
Worst Case: O(n) since the process continues until the main tank is empty.
Description: The time complexity is linear as we process each fuel consumption step.
Best Case: O(1) since no extra space is needed.
Worst Case: O(1) as we only use a constant amount of space.
Description: The space complexity is constant because we only need variables to track the fuel amounts.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus