Leetcode 2716: Minimize String Length
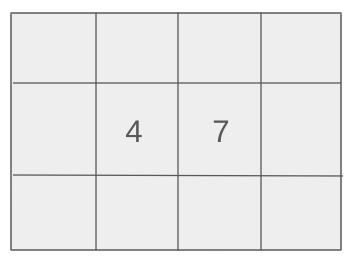
You are given a string
s
. You can perform two types of operations: (1) Choose an index i
and remove the closest occurrence of the character at i
to the left of i
. (2) Choose an index i
and remove the closest occurrence of the character at i
to the right of i
. Minimize the length of the string s
by performing these operations and return the final minimized length.Problem
Approach
Steps
Complexity
Input: A string `s` consisting of lowercase English letters.
Example: s = "abbccaa"
Constraints:
• 1 <= s.length <= 100
• s consists only of lowercase English letters.
Output: Return the length of the minimized string after performing the operations.
Example: 3
Constraints:
• The output is a non-negative integer.
Goal: The goal is to minimize the length of the string by repeatedly applying the operations where possible.
Steps:
• Identify the closest duplicate characters in the string.
• Remove one of the duplicates by performing one of the operations.
• Repeat this process until no duplicates are left.
Goal: The input string will have a length between 1 and 100 characters.
Steps:
• 1 <= s.length <= 100
• The string contains only lowercase English letters.
Assumptions:
• The input string is non-empty and contains only lowercase English letters.
• Input: Example 1
• Explanation: For the input 'abbccaa', applying the operations step-by-step reduces the string to 'bca' with length 3.
• Input: Example 2
• Explanation: For the input 'abc', no operations are performed, and the length of the string remains 3.
• Input: Example 3
• Explanation: For the input 'aabbbcc', applying the operations reduces the string to 'abc', with a final length of 3.
Approach: We can solve this problem by iterating through the string and finding duplicate characters. For each duplicate, we perform an operation to remove one of them and continue until no duplicates remain.
Observations:
• The string is reduced by removing duplicate characters.
• The operations only affect characters that have duplicates.
• The key observation is that the minimum string length is determined by the number of unique characters in the string.
Steps:
• Iterate through the string and store all characters in a set to identify unique characters.
• The length of the set will give the number of unique characters, which is the minimized string length.
Empty Inputs:
• An empty string is not a valid input.
Large Inputs:
• The string length is at most 100 characters, which can be handled efficiently.
Special Values:
• If the string has no repeated characters, no operations are needed.
Constraints:
• The solution must be efficient for strings with lengths up to 100.
int minimizedStringLength(string s) {
unordered_set<char> st;
for(auto c: s) st.insert(c);
return st.size();
}
1 : Function Definition
int minimizedStringLength(string s) {
This line defines the function `minimizedStringLength`, which takes a string `s` as an argument and returns an integer value representing the count of unique characters in the string.
2 : Set Initialization
unordered_set<char> st;
An unordered set `st` is created to store unique characters from the input string `s`. The unordered set ensures that each character is stored only once.
3 : Loop Through String
for(auto c: s) st.insert(c);
A loop iterates through each character `c` in the string `s`, and each character is inserted into the unordered set `st`. If a character is already present in the set, it is not added again.
4 : Return Size
return st.size();
After processing all characters in the string, the size of the unordered set `st` is returned. This size represents the number of unique characters in the string.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The solution runs in linear time since we only need to traverse the string once and find unique characters.
Best Case: O(1)
Worst Case: O(n)
Description: The space complexity is O(n) for storing unique characters in a set.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus