Leetcode 2711: Difference of Number of Distinct Values on Diagonals
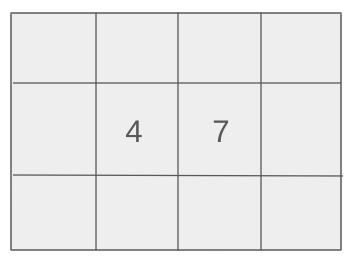
Given a 2D grid of size m x n, you are tasked with finding a new matrix where each cell value is the absolute difference between the count of distinct values in the diagonal cells to the left and above it, and the count of distinct values in the diagonal cells to the right and below it.
Problem
Approach
Steps
Complexity
Input: The input consists of a 2D grid (matrix) with m rows and n columns, where each cell contains an integer.
Example: Input: grid = [[4, 2, 7], [1, 5, 8], [9, 6, 3]]
Constraints:
• 1 <= m, n <= 50
• 1 <= grid[i][j] <= 50
Output: Return a new matrix where each element at position [r][c] is the absolute difference between the number of distinct values on the diagonal to the left and above the cell and the number of distinct values on the diagonal to the right and below the cell.
Example: Output: [[0, 0, 1], [0, 0, 1], [1, 0, 0]]
Constraints:
• The result should be an m x n matrix.
Goal: To compute the difference between the number of distinct values on diagonals of the matrix for each cell.
Steps:
• Step 1: For each cell in the matrix, compute the distinct values on the diagonal above and to the left (leftAbove).
• Step 2: For each cell in the matrix, compute the distinct values on the diagonal below and to the right (rightBelow).
• Step 3: For each cell, compute the absolute difference between the sizes of the distinct values in leftAbove and rightBelow, and store this in the result matrix.
Goal: The matrix has at least one row and one column and at most 50 rows and 50 columns.
Steps:
• Each value in the grid is an integer between 1 and 50.
Assumptions:
• The input grid is non-empty and contains valid integers within the specified range.
• Input: Input: grid = [[4, 2, 7], [1, 5, 8], [9, 6, 3]]
• Explanation: The output matrix is calculated as follows:
For cell [0][0], leftAbove is empty and rightBelow contains distinct values {5, 6, 3}, so the result is |0 - 3| = 3. Repeat this for all cells.
• Input: Input: grid = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
• Explanation: For each cell, we calculate the leftAbove and rightBelow diagonals and their distinct values, then compute the absolute difference.
Approach: The approach involves iterating over the grid and for each element, finding the distinct values in the diagonals above-left and below-right, then calculating the absolute difference.
Observations:
• Each element's value is determined by its position in the grid and the diagonals to the left and above, and to the right and below.
• Using sets will help in counting distinct values on each diagonal.
Steps:
• Step 1: Initialize a result matrix of the same size as the input grid.
• Step 2: For each cell, calculate the leftAbove diagonal values using a set.
• Step 3: For each cell, calculate the rightBelow diagonal values using a set.
• Step 4: For each cell, calculate the absolute difference between the sizes of the two sets and store the result.
Empty Inputs:
• The input grid will not be empty as per the constraints.
Large Inputs:
• For large grids (50x50), ensure that the solution is optimized for performance.
Special Values:
• For grids where all values are the same, the result matrix will be all zeros.
Constraints:
• Ensure that the solution works for both square and rectangular matrices.
vector<vector<int>> differenceOfDistinctValues(vector<vector<int>>& grid) {
int m = grid.size(), n = grid[0].size();
vector<vector<int>> ans(m, vector<int>(n, 0));
set<int> ls, rs;
for(int i = 0; i < m; i++)
for(int j = 0; j < n; j++) {
int r = i - 1, c = j - 1;
while(r >= 0 && c >= 0) {
ls.insert(grid[r][c]);
r--;
c--;
}
r = i + 1, c = j + 1;
while(r < m && c < n) {
rs.insert(grid[r][c]);
r++;
c++;
}
int res = ls.size() - rs.size();
ans[i][j] = abs(res);
ls.clear();
rs.clear();
}
return ans;
}
1 : Function Declaration
vector<vector<int>> differenceOfDistinctValues(vector<vector<int>>& grid) {
The function `differenceOfDistinctValues` is declared, which takes a reference to a 2D vector `grid` and returns a 2D vector of integers representing the difference in distinct values for each grid element.
2 : Variable Initialization
int m = grid.size(), n = grid[0].size();
Initialize variables `m` and `n` to store the number of rows and columns of the grid, respectively.
3 : 2D Vector Initialization
vector<vector<int>> ans(m, vector<int>(n, 0));
Initialize the 2D vector `ans` with the same dimensions as the grid and fill it with zeros. This will store the result of the distinct value differences.
4 : Set Declaration
set<int> ls, rs;
Declare two sets, `ls` and `rs`, to store the distinct values found in the upper-left and bottom-right diagonals, respectively.
5 : Outer Loop (Rows)
for(int i = 0; i < m; i++)
Iterate through each row of the grid using the index `i`.
6 : Inner Loop (Columns)
for(int j = 0; j < n; j++) {
Iterate through each column of the grid using the index `j`.
7 : Diagonal Calculation
int r = i - 1, c = j - 1;
Initialize variables `r` and `c` to traverse the upper-left diagonal from the current position `(i, j)`. The initial values set the pointers to the position just above and to the left of the current element.
8 : Diagonal Traversal (Left-Up)
while(r >= 0 && c >= 0) {
Begin a loop to traverse the upper-left diagonal, stopping when we reach the boundary of the grid.
9 : Set Insertion (Left-Up)
ls.insert(grid[r][c]);
Insert the current element from the upper-left diagonal into the set `ls`, ensuring only distinct values are stored.
10 : Pointer Update (Left-Up)
r--;
Move the pointer `r` upwards to continue traversing the diagonal.
11 : Pointer Update (Left-Up)
c--;
Move the pointer `c` leftwards to continue traversing the diagonal.
12 : Diagonal Setup (Right-Down)
r = i + 1, c = j + 1;
Reinitialize `r` and `c` to point to the position just below and to the right of the current element `(i, j)` for the bottom-right diagonal traversal.
13 : Diagonal Traversal (Right-Down)
while(r < m && c < n) {
Begin a loop to traverse the bottom-right diagonal, stopping when we reach the boundary of the grid.
14 : Set Insertion (Right-Down)
rs.insert(grid[r][c]);
Insert the current element from the bottom-right diagonal into the set `rs`, ensuring only distinct values are stored.
15 : Pointer Update (Right-Down)
r++;
Move the pointer `r` downwards to continue traversing the diagonal.
16 : Pointer Update (Right-Down)
c++;
Move the pointer `c` rightwards to continue traversing the diagonal.
17 : Difference Calculation
int res = ls.size() - rs.size();
Calculate the difference between the number of distinct elements in the upper-left (`ls`) and bottom-right (`rs`) diagonals.
18 : Store Result
ans[i][j] = abs(res);
Store the absolute value of the difference in the result matrix `ans` at position `(i, j)`.
19 : Clear Sets
ls.clear();
Clear the `ls` set in preparation for the next iteration.
20 : Clear Sets
rs.clear();
Clear the `rs` set in preparation for the next iteration.
21 : Return Statement
return ans;
Return the `ans` matrix, which contains the differences of distinct values for each element in the grid.
Best Case: O(m * n)
Average Case: O(m * n)
Worst Case: O(m * n)
Description: The time complexity is O(m * n) because we need to process each cell and its diagonals, where m is the number of rows and n is the number of columns.
Best Case: O(1)
Worst Case: O(m * n)
Description: The space complexity is O(m * n) for storing the result matrix, and additional space is used for the sets storing distinct values in the diagonals.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus