Leetcode 2673: Make Costs of Paths Equal in a Binary Tree
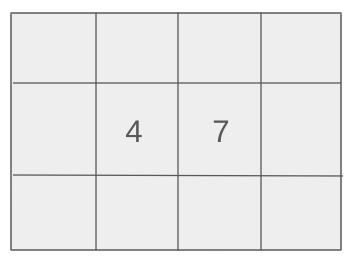
You are given a perfect binary tree with
n
nodes, where each node has a cost associated with it. The tree is numbered from 1 to n, with node 1 as the root. For each node i
, its left child is 2*i
and its right child is 2*i + 1
. You are allowed to increment the cost of any node by 1 any number of times. Your task is to return the minimum number of increments required to make the total cost of the path from the root to each leaf node equal.Problem
Approach
Steps
Complexity
Input: The input consists of an integer `n` representing the number of nodes in a perfect binary tree, followed by a list of integers `cost`, where `cost[i]` is the cost of node `i+1`.
Example: Input: n = 5, cost = [2, 4, 3, 2, 5]
Constraints:
• 3 <= n <= 10^5
• n + 1 is a power of 2
• cost.length == n
• 1 <= cost[i] <= 10^4
Output: The output should be a single integer, which represents the minimum number of increments required to make the cost of all root-to-leaf paths equal.
Example: Output: 4
Constraints:
• The result should be a non-negative integer.
Goal: The goal is to minimize the number of increments required to equalize the path costs.
Steps:
• Step 1: Starting from the leaf nodes, propagate the required increments up to the root node, ensuring each path has the same total cost.
• Step 2: For each non-leaf node, compare the costs of its left and right children. Increment the smaller one to match the larger one and count the number of increments.
• Step 3: Repeat the process until you reach the root node.
Goal: The solution must efficiently handle the constraints specified.
Steps:
• Ensure the solution works within O(n) time complexity to handle the largest inputs.
Assumptions:
• The tree is a perfect binary tree, meaning every non-leaf node has exactly two children.
• All nodes have a non-negative cost value.
• Input: Input: n = 5, cost = [2, 4, 3, 2, 5]
• Explanation: In this case, the two paths from root to leaves are [2, 4, 5] and [2, 3, 5]. To equalize the path costs, we increment the cost of node 2 to match node 3's cost. This requires 4 increments, so the total increments required are 4.
Approach: To solve this problem, we will work our way from the leaf nodes upwards to the root, balancing the path costs by making necessary increments.
Observations:
• We need to ensure that the cost of each root-to-leaf path is the same.
• This requires comparing the costs of left and right children of every node and balancing them.
• Starting from the leaf nodes and adjusting the internal nodes' costs seems to be an efficient way to solve this problem.
Steps:
• Step 1: Initialize an array `cost` of size `n`.
• Step 2: Start from the last non-leaf node and move upwards. For each node, compare the costs of its left and right children and increment the smaller cost to match the larger one.
• Step 3: Accumulate the number of increments required as you move upwards towards the root.
Empty Inputs:
• The input will always have at least 3 nodes, ensuring a valid binary tree structure.
Large Inputs:
• The solution must handle up to 10^5 nodes efficiently.
Special Values:
• If the tree already has equal costs for all root-to-leaf paths, the result should be 0 increments.
Constraints:
• Ensure the solution runs in O(n) time complexity to handle the largest inputs.
int minIncrements(int n, vector<int>& A) {
int res = 0;
for (int i = n / 2 - 1; i >= 0; --i) {
int l = i * 2 + 1, r = i * 2 + 2;
res += abs(A[l] - A[r]);
A[i] += max(A[l], A[r]);
}
return res;
}
1 : Function Definition
int minIncrements(int n, vector<int>& A) {
This is the function definition of 'minIncrements', which takes an integer 'n' (size of the array) and a reference to a vector 'A'. The goal is to compute the minimum number of increments needed to turn the array into a valid heap.
2 : Result Initialization
int res = 0;
We initialize 'res' to 0. This variable will keep track of the total number of increments made while adjusting the array.
3 : Loop Start
for (int i = n / 2 - 1; i >= 0; --i) {
We start a loop that iterates over the parent nodes of the binary heap, starting from the last parent node (at index 'n / 2 - 1') and moving upwards.
4 : Child Nodes Calculation
int l = i * 2 + 1, r = i * 2 + 2;
For each parent node, we calculate the indices of its left ('l') and right ('r') children using the standard binary heap indexing formula.
5 : Difference Calculation
res += abs(A[l] - A[r]);
We calculate the absolute difference between the values of the left and right children, adding this value to 'res' to account for the required increments to make the children equal.
6 : Adjust Parent Value
A[i] += max(A[l], A[r]);
To maintain the heap property, we adjust the parent node's value by adding the maximum of its left or right child's value.
7 : Return Result
return res;
We return the total number of increments made to the array, stored in 'res'.
Best Case: O(n)
Average Case: O(n)
Worst Case: O(n)
Description: The time complexity is O(n) as we only need to traverse the tree once, adjusting costs from the leaves to the root.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space needed for the cost array.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus