Leetcode 2656: Maximum Sum With Exactly K Elements
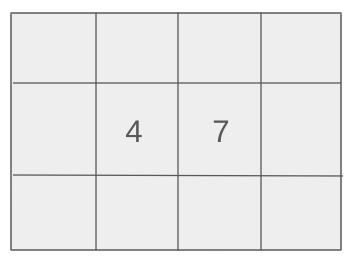
You are given a 0-indexed array of positive integers ’nums’ and an integer ‘k’. You need to perform the following operation exactly k times to maximize your score: Select an element from nums, remove it from the array, and add a new element with a value one greater than the selected element. The score is increased by the value of the selected element. Your task is to return the maximum score you can achieve after performing the operation exactly k times.
Problem
Approach
Steps
Complexity
Input: The input consists of an array of positive integers nums and an integer k.
Example: Input: nums = [3, 8, 5, 1], k = 4
Constraints:
• 1 <= nums.length <= 100
• 1 <= nums[i] <= 100
• 1 <= k <= 100
Output: Return the maximum score you can achieve after performing the operation exactly k times.
Example: Output: 29
Constraints:
• The output should be an integer representing the maximum score achievable.
Goal: Maximize the score by selecting the largest possible number from the array at each step and incrementing it.
Steps:
• Step 1: Find the largest number in the array.
• Step 2: Perform the operation on this number, adding it to the score, and increment the number.
• Step 3: Repeat the operation exactly k times, always selecting the largest number available.
Goal: Ensure that the solution works efficiently within the given constraints of nums.length, nums[i], and k.
Steps:
• The solution should handle up to 100 elements in nums and handle values up to 100 for nums[i] efficiently.
Assumptions:
• The array nums is non-empty, with at least one element.
• It is guaranteed that the operation can be performed exactly k times.
• Input: Input: nums = [3, 8, 5, 1], k = 4
• Explanation: In this case, to maximize the score, we select the elements in the following order: 8, 9, 9, 10. The resulting score is 8 + 9 + 9 + 10 = 29.
• Input: Input: nums = [1, 1, 1], k = 2
• Explanation: Here, we choose the element 1 in the first operation, resulting in nums = [1, 1, 2]. In the second operation, we choose 2, resulting in nums = [1, 3, 2]. The score is 1 + 2 = 3.
Approach: To solve this problem, we can use a greedy approach. In each step, we select the largest element from the array, add it to the score, and increment the value of that element.
Observations:
• We need to maximize the score by selecting the largest element at each step, which can be efficiently done using a priority queue or sorting.
• The largest element will always provide the highest score when selected, so the approach should focus on efficiently retrieving and updating the largest element.
Steps:
• Step 1: Sort the array or use a max-heap to efficiently retrieve the largest element.
• Step 2: Perform the operation exactly k times, selecting the largest element at each step.
• Step 3: After each operation, increment the selected element and update the score.
Empty Inputs:
• There will be no empty arrays as per the constraints.
Large Inputs:
• The input size can be up to 100, so the solution should work efficiently for up to 100 elements.
Special Values:
• If all elements are the same, we should select the largest element repeatedly, as incrementing the same number will maximize the score.
Constraints:
• Ensure the solution works within the time limits for the maximum input size.
int maximizeSum(vector<int>& nums, int k) {
int i = *max_element(nums.begin(), nums.end());
return i * k + k * (k - 1) / 2;
}
1 : Variable Initialization
int maximizeSum(vector<int>& nums, int k) {
This line defines the function maximizeSum, which takes in a vector of integers (nums) and an integer k as inputs. The goal is to maximize the sum of the sequence derived from these inputs.
2 : Function Call
int i = *max_element(nums.begin(), nums.end());
Here, we find the maximum element in the input vector using the max_element function. The result is stored in the variable 'i'. This step ensures we are working with the largest number in the sequence.
3 : Mathematical Operations
return i * k + k * (k - 1) / 2;
This line calculates the final result. The first part, i * k, gives the sum of the largest element (i) repeated k times. The second part, k * (k - 1) / 2, is the sum of the integers from 0 to (k-1), which is added to the result.
Best Case: O(n log n)
Average Case: O(n log n)
Worst Case: O(n log n)
Description: Sorting the array takes O(n log n) time, and each operation after sorting takes constant time, so the overall complexity is dominated by the sorting step.
Best Case: O(n)
Worst Case: O(n)
Description: The space complexity is O(n) due to the space required for the array or a max-heap.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus