Leetcode 2651: Calculate Delayed Arrival Time
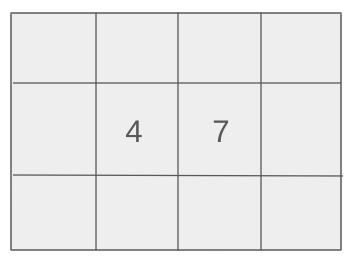
You are given two integers: ‘arrivalTime’, representing the scheduled arrival time of a train in hours (in 24-hour format), and ‘delayedTime’, representing the delay in hours. Your task is to compute the time at which the train will actually arrive at the station, taking into account the delay.
Problem
Approach
Steps
Complexity
Input: The input consists of two integers: 'arrivalTime' (1 <= arrivalTime < 24) and 'delayedTime' (1 <= delayedTime <= 24).
Example: arrivalTime = 9, delayedTime = 4
Constraints:
• 1 <= arrivalTime < 24
• 1 <= delayedTime <= 24
Output: Return the time at which the train will arrive at the station, which can be in 24-hour format.
Example: Output: 13
Constraints:
• The result will be an integer between 0 and 23 (inclusive).
Goal: The goal is to compute the new arrival time after adding the delay to the given arrival time. The result should be returned in 24-hour format.
Steps:
• Step 1: Add the 'delayedTime' to the 'arrivalTime'.
• Step 2: If the sum exceeds 24 hours, subtract 24 to wrap around the time in 24-hour format.
• Step 3: Return the final computed arrival time.
Goal: The solution should handle times correctly in the 24-hour format.
Steps:
• 1 <= arrivalTime < 24
• 1 <= delayedTime <= 24
Assumptions:
• The input values for 'arrivalTime' and 'delayedTime' are valid integers within the given constraints.
• The result is expected in 24-hour format.
• Input: Input: arrivalTime = 9, delayedTime = 4
• Explanation: The scheduled arrival time is 9:00 hours. Adding a delay of 4 hours gives us 13:00 hours, so the output will be 13.
• Input: Input: arrivalTime = 20, delayedTime = 7
• Explanation: The scheduled arrival time is 20:00 hours. Adding a delay of 7 hours gives us 27:00, which, when wrapped around in 24-hour format, becomes 3:00 hours. So the output will be 3.
Approach: The approach is simple: Add the delay to the arrival time and handle wrapping around the 24-hour format using modulo operation.
Observations:
• We need to account for the fact that the time can exceed 24 hours and needs to be wrapped around.
• We can use the modulo operator to handle the wrapping around of time, ensuring the result stays within 0-23 hours.
Steps:
• Step 1: Add the delayedTime to the arrivalTime.
• Step 2: Apply modulo 24 operation to the result to ensure it stays within the range of a 24-hour clock.
• Step 3: Return the final value.
Empty Inputs:
• There are no empty inputs as per the problem's constraints.
Large Inputs:
• Ensure the solution works efficiently when delayedTime equals the upper constraint of 24 hours.
Special Values:
• Consider the edge case where arrivalTime is near midnight (e.g., 23:00) and the delay pushes the time to the next day.
Constraints:
• The time values must always remain within the valid 24-hour format.
int findDelayedArrivalTime(int at, int dt) {
return (at + dt) % 24;
}
1 : Function Definition
int findDelayedArrivalTime(int at, int dt) {
This line defines the function 'findDelayedArrivalTime', which takes two integer parameters: 'at' (current time) and 'dt' (delayed time), and returns the delayed arrival time as an integer.
2 : Calculation
return (at + dt) % 24;
This line calculates the delayed arrival time by adding the current time ('at') to the delay ('dt') and applying the modulus operator to ensure the result is within the 24-hour format.
Best Case: O(1)
Average Case: O(1)
Worst Case: O(1)
Description: The time complexity is constant since the solution involves a fixed number of operations regardless of input size.
Best Case: O(1)
Worst Case: O(1)
Description: The space complexity is constant because we only need a fixed amount of extra space for storing the inputs and result.
LeetCode Solutions Library / DSA Sheets / Course Catalog |
---|
comments powered by Disqus